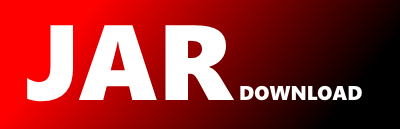
com.day.cq.search.suggest.Term Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* Copyright 1997-2010 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.search.suggest;
import java.util.Comparator;
/**
* Represents a string term with a frequency.
*
*
* Sorted alphabetically by default.
*/
public class Term implements Comparable {
private String term;
private int freq;
public Term(String term, int freq) {
this.term = term;
this.freq = freq;
}
public String term() {
return term;
}
public void setTerm(String term) {
this.term = term;
}
public int freq() {
return freq;
}
public void setFreq(int freq) {
this.freq = freq;
}
public int compareTo(Term o) {
return term().compareTo(o.term());
}
public static Comparator sortByFreq() {
return new Comparator() {
public int compare(Term a, Term b) {
return b.freq() - a.freq();
}
};
}
public static Comparator sortAlphabetically() {
return new Comparator() {
public int compare(Term a, Term b) {
return a.term().compareTo(b.term());
}
};
}
}