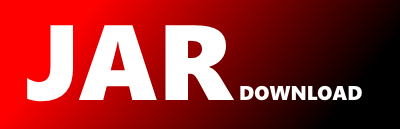
com.day.cq.search.writer.FullNodeHitWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2009 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.search.writer;
import javax.jcr.Node;
import javax.jcr.NodeIterator;
import javax.jcr.PropertyIterator;
import javax.jcr.RepositoryException;
import javax.jcr.Session;
import org.apache.sling.commons.json.JSONException;
import org.apache.sling.commons.json.io.JSONWriter;
import org.apache.sling.commons.json.jcr.JsonItemWriter;
import com.day.cq.search.Query;
import com.day.cq.search.result.Hit;
import org.osgi.service.component.annotations.Component;
import org.osgi.service.component.propertytypes.ServiceVendor;
/**
* {@linkplain FullNodeHitWriter} writes the full node using sling's standard
* jcr node to json mapping.
*/
@ServiceVendor("Adobe Systems Incorporated")
@Component(factory = "com.day.cq.search.writer.ResultHitWriter/full")
public class FullNodeHitWriter implements ResultHitWriter {
public void write(Hit hit, JSONWriter writer, Query query) throws RepositoryException, JSONException {
int maxRecursionLevels = Integer.parseInt(query.getPredicates().get("nodedepth", "0"));
boolean writeACLs = query.getPredicates().getBool("acls");
writer.key("jcr:path").value(hit.getPath());
new JsonNodeDumper(writeACLs).dumpObject(hit.getNode(), writer, 0, maxRecursionLevels);
}
/**
* Helper class that allows to reuse an existing {@link JSONWriter} for
* dumping a JCR node.
*/
private static class JsonNodeDumper extends JsonItemWriter {
private boolean writeACLs = false;
public JsonNodeDumper(boolean writeACLs) {
super(null);
this.writeACLs = writeACLs;
}
@Override
protected void dump(Node node, JSONWriter w, int currentRecursionLevel, int maxRecursionLevels)
throws RepositoryException, JSONException {
w.object();
dumpObject(node, w, currentRecursionLevel, maxRecursionLevels);
w.endObject();
}
public void dumpObject(Node node, JSONWriter w, int currentRecursionLevel, int maxRecursionLevels)
throws RepositoryException, JSONException {
PropertyIterator props = node.getProperties();
if (writeACLs) {
writeACL(node, w);
}
// the node's actual properties
while (props.hasNext()) {
writeProperty(w, props.nextProperty());
}
// the child nodes
if (recursionLevelActive(currentRecursionLevel , maxRecursionLevels)) {
final NodeIterator children = node.getNodes();
while (children.hasNext()) {
final Node n = children.nextNode();
dumpSingleNode(n, w, currentRecursionLevel, maxRecursionLevels);
}
}
}
protected void writeACL(Node node, JSONWriter w) throws RepositoryException, JSONException {
Session session = node.getSession();
w.key("jcr:permissions").object();
// mapping JCR permissions:
// add_node (new node path), set_property (prop path), remove (item path), read (item path)
// onto cq actions (see com.day.cq.security.util.CqActions):
// create, modify, delete, read
// read is omitted, since we only get to this point with a read
w.key("create").value(session.hasPermission(node.getPath() + "/newnode", "add_node"));
w.key("modify").value(session.hasPermission(node.getPath() + "/newprop", "set_property"));
w.key("delete").value(session.hasPermission(node.getPath(), "remove"));
w.endObject();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy