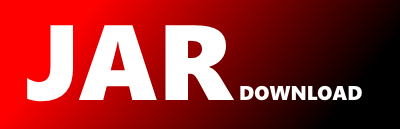
com.day.cq.spellchecker.TextCheckResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.day.cq.spellchecker;
import org.apache.sling.commons.json.JSONException;
import org.apache.sling.commons.json.io.JSONStringer;
import java.util.ArrayList;
import java.util.List;
/**
* This class represents the spellchecking results when checking against an entire text.
*/
public class TextCheckResult {
static private class WordResult {
private final int startPos;
private final int charCnt;
private final WordCheckResult result;
WordResult(int startPos, int charCnt, WordCheckResult result) {
this.startPos = startPos;
this.charCnt = charCnt;
this.result = result;
}
public int getStartPos() {
return this.startPos;
}
public int getCharCnt() {
return this.charCnt;
}
public WordCheckResult getResult() {
return this.result;
}
}
/**
* The spellchecked text
*/
private final String text;
/**
* Flag that determines if the text contains HTML code
*/
private final boolean isHtml;
/**
* Language used for spellchecking
*/
private final String language;
/**
* Spellchecking results on a per-word base
*/
private final List results;
public TextCheckResult(String text, boolean isHtml, String language) {
this.text = text;
this.isHtml = isHtml;
this.language = language;
this.results = new ArrayList(10);
}
public void addWordResult(int startPos, int charCnt, WordCheckResult wordResult) {
this.results.add(new WordResult(startPos, charCnt, wordResult));
}
public WordResult getWordResult(int pos) {
for (WordResult wordResult : this.results) {
int startPos = wordResult.getStartPos();
int endPos = startPos + wordResult.getCharCnt();
if ((pos >= startPos) && (pos < endPos)) {
return wordResult;
}
}
return null;
}
public String getText() {
return this.text;
}
public boolean isHtml() {
return this.isHtml;
}
public String getLanguage() {
return this.language;
}
public String toJsonString() throws SpellCheckException {
return this.toJsonString(false);
}
public String toJsonString(boolean sparse) throws SpellCheckException {
try {
JSONStringer json = new JSONStringer();
json.object();
json.key("language");
json.value(this.language);
json.key("words");
json.array();
for (WordResult wordResult : this.results) {
if (!sparse || !wordResult.getResult().isCorrect()) {
json.object();
json.key("start");
json.value(wordResult.getStartPos());
json.key("chars");
json.value(wordResult.getCharCnt());
json.key("result");
wordResult.getResult().addToJsonStringer(json);
json.endObject();
}
}
json.endArray();
json.endObject();
return json.toString();
} catch (JSONException je) {
throw new SpellCheckException("Could not JSON-ify spellcheck result.", je);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy