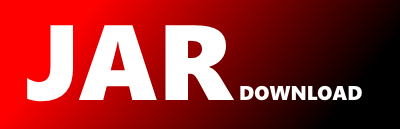
com.day.cq.wcm.api.PageManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2008 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.wcm.api;
import java.util.Calendar;
import java.util.Collection;
import java.util.function.Predicate;
import javax.jcr.Node;
import org.apache.sling.api.resource.Resource;
import org.osgi.annotation.versioning.ProviderType;
import com.day.cq.wcm.api.msm.Blueprint;
/**
* The page manager provides methods for page level operations.
*/
@ProviderType
public interface PageManager {
/**
* Convenience method that returns the page at the given path. If the
* resource at that path does not exist or is not adaptable to Page,
* null
is returned.
* @param path path of the page
* @return page or null
*/
Page getPage(String path);
/**
* Returns the page that contains this resource. If the resource is a page
* the resource is returned. Otherwise it walks up the parent resources
* until a page is found.
* @param resource resource to find the page for
* @return page or null
if not found.
*/
Page getContainingPage(Resource resource);
/**
* Returns the page that contains the resource at the given path. If the path addresses a page, that page is
* returned. Otherwise it walks up the parent resources until a page is found.
* @param path path to find the page for
* @return page or null
if not found.
*/
Page getContainingPage(String path);
/**
* Creates a new page at the given path using the provided template as
* content template. If a no pageName is given but a title, then the title
* is used as hint for the name of the new page. Changes are automatically
* saved.
*
* @param parentPath the path of the parent page
* @param pageName the name of the new page
* @param template the template for the new page
* @param title the title of the new page
* @return the page that was created
* @throws WCMException if an error during this operation occurs.
*/
Page create(String parentPath, String pageName, String template, String title)
throws WCMException;
/**
* Creates a new page at the given path using the provided template as
* content template. If a no pageName is given but a title, then the title
* is used as hint for the name of the new page.
*
* @param parentPath the path of the parent page
* @param pageName the name of the new page
* @param template the template for the new page
* @param title the title of the new page
* @param autoSave if true
saves the modifications.
* @return the page that was created
* @throws WCMException if an error during this operation occurs.
*/
Page create(String parentPath, String pageName, String template, String title,
boolean autoSave) throws WCMException;
/**
* Moves the given page to the new destination and automatically saves the changes.
* If source and destination are equals the page is just ordered.
*
* @param page the page to move
* @param destination the path of the new destination
* @param beforeName the name of the next page. if null
the page
* is ordered at the end.
* @param shallow if true
only the page content is moved
* @param resolveConflict if true
resolves name conflict if
* destination already exists.
* @param adjustRefs list of paths to pages that refer to the moved one.
* those references will be adjusted.
* @return the new page at the new location
* @throws WCMException if an error during this operation occurs.
*/
Page move(Page page, String destination, String beforeName, boolean shallow,
boolean resolveConflict, String[] adjustRefs)
throws WCMException;
/**
* Moves the given page to the new destination and automatically saves the changes.
* If source and destination are equals the page is just ordered.
*
* @param page the page to move
* @param destination the path of the new destination
* @param beforeName the name of the next page. if null
the page
* is ordered at the end.
* @param shallow if true
only the page content is moved
* @param resolveConflict if true
resolves name conflict if
* destination already exists.
* @param adjustRefs list of paths to pages that refer to the moved one.
* those references will be adjusted.
* @param publishRefs list of referencing paths that will be republished.
* @return the new page at the new location
* @throws WCMException if an error during this operation occurs.
*/
Page move(Page page, String destination, String beforeName, boolean shallow,
boolean resolveConflict, String[] adjustRefs, String[] publishRefs)
throws WCMException;
/**
* Moves the given resource to the new destination and automatically saves the changes.
* If source and destination are equals the resource is just ordered.
*
* @param resource the resource to move
* @param destination the path of the new destination
* @param beforeName the name of the next resource. if null
the resource
* is ordered at the end.
* @param shallow if true
only the resource content is moved.
* this is currently only supported for pages.
* @param resolveConflict if true
resolves name conflict if
* destination already exists.
* @param adjustRefs list of paths to pages that refer to the moved one.
* those references will be adjusted.
* @return the new resource at the new location
* @throws WCMException if an error during this operation occurs.
*/
Resource move(Resource resource, String destination, String beforeName,
boolean shallow, boolean resolveConflict, String[] adjustRefs)
throws WCMException;
/**
* Moves the given resource to the new destination and automatically saves the changes.
* If source and destination are equals the resource is just ordered.
*
* @param resource the resource to move
* @param destination the path of the new destination
* @param beforeName the name of the next resource. if null
the resource
* is ordered at the end.
* @param shallow if true
only the resource content is moved.
* this is currently only supported for pages.
* @param resolveConflict if true
resolves name conflict if
* destination already exists.
* @param adjustRefs list of paths to pages that refer to the moved one.
* those references will be adjusted.
* @param publishRefs list of referencing paths that will be republished.
* @return the new resource at the new location
* @throws WCMException if an error during this operation occurs.
*/
Resource move(Resource resource, String destination, String beforeName,
boolean shallow, boolean resolveConflict, String[] adjustRefs, String[] publishRefs)
throws WCMException;
/**
* Options object used for {@link #copy(CopyOptions)}.
*/
public static class CopyOptions {
/**
* The page to copy (if {@link #resource} is not provided).
*/
public Page page;
/**
* The resource to copy
*/
public Resource resource;
/**
* The destination
*/
public String destination;
/**
* The name of the next page. If {@code null} the page is ordered at the end.
*/
public String beforeName;
/**
* If {@code true} a non-recursive copy is performed.
*/
public boolean shallow;
/**
* If {@code true} resolves name conflict if destination already exists.
*/
public boolean resolveConflict;
/**
* If {@code true} saves the modifications.
*/
public boolean autoSave;
/**
* If {@code true} adjusts any content references to the new location.
*/
public boolean adjustReferences;
/**
* If {@code true} the current resource needs to be overridden (mainly because it has a resourceType outofscope)
*/
public boolean overridePage;
}
/**
* Overrides the given page.
*
* @param options the options for this override operation. {@code page} or {@code resource} are required to be
* defined, as well as {@code destination}. Also, it is important to define
* {@code overridePage} in case the page already exists and needs to be overridden
* @return the overridden page
* @throws WCMException if an error during this operation occurs.
*/
Resource override(CopyOptions options) throws WCMException;
/**
* Copies the given page to the new destination.
*
* @param options the options for this copy operation. {@code page} or {@code resource} are required
* defined, as well as {@code destination}.
* @return the copied page
* @throws WCMException if an error during this operation occurs.
*/
Resource copy(CopyOptions options) throws WCMException;
/**
* Copies the given page to the new destination and automatically saves the modifications
*
* @param page the page to copy
* @param destination the destination
* @param beforeName the name of the next page. if null
the page
* is ordered at the end.
* @param shallow if true
a non-recursive copy is performed.
* @param resolveConflict if true
resolves name conflict if
* destination already exists.
* @return the copied page
* @throws WCMException if an error during this operation occurs.
*/
Page copy(Page page, String destination, String beforeName, boolean shallow,
boolean resolveConflict) throws WCMException;
/**
* Copies the given page to the new destination
*
* @param page the page to copy
* @param destination the destination
* @param beforeName the name of the next page. if null
the page
* is ordered at the end.
* @param shallow if true
a non-recursive copy is performed.
* @param resolveConflict if true
resolves name conflict if
* destination already exists.
* @param autoSave if true
saves the modifications.
* @return the copied page
* @throws WCMException if an error during this operation occurs.
*/
Page copy(Page page, String destination, String beforeName, boolean shallow,
boolean resolveConflict, boolean autoSave) throws WCMException;
/**
* Copies the given resource to the new destination and automatically saves the modifications
*
* @param resource the resource to copy
* @param destination the destination
* @param beforeName the name of the next resource. if null
the
* resource is ordered at the end.
* @param shallow if true
a non-recursive copy is performed.
* this is currently only supported for pages.
* @param resolveConflict if true
resolves name conflict if
* destination already exists.
* @return the copied resource
* @throws WCMException if an error during this operation occurs.
*/
Resource copy(Resource resource, String destination, String beforeName,
boolean shallow, boolean resolveConflict) throws WCMException;
/**
* Copies the given resource to the new destination
*
* @param resource the resource to copy
* @param destination the destination
* @param beforeName the name of the next resource. if null
the
* resource is ordered at the end.
* @param shallow if true
a non-recursive copy is performed.
* this is currently only supported for pages.
* @param resolveConflict if true
resolves name conflict if
* destination already exists.
* @param autoSave if true
saves the modifications.
* @return the copied resource
* @throws WCMException if an error during this operation occurs.
*/
Resource copy(Resource resource, String destination, String beforeName,
boolean shallow, boolean resolveConflict, boolean autoSave) throws WCMException;
/**
* Deletes the page and saves the change.
* @param page the page to delete
* @param shallow if true
only the content of the page is deleted
* but not it's child pages
* @throws WCMException if an error during this operation occurs.
*/
void delete(Page page, boolean shallow) throws WCMException;
/**
* Deletes the page.
* @param page the page to delete
* @param shallow if true
only the content of the page is deleted
* but not it's child pages
* @param autoSave if true
saves the modifications.
* @throws WCMException if an error during this operation occurs.
*/
void delete(Page page, boolean shallow, boolean autoSave) throws WCMException;
/**
* Deletes the resource and saves the change.
* @param resource the resource to delete
* @param shallow if true
only the content of the resource is deleted
* but not it's child resources. only supported for pages.
* @throws WCMException if an error during this operation occurs.
*/
void delete(Resource resource, boolean shallow) throws WCMException;
/**
* Deletes the resource.
* @param resource the resource to delete
* @param shallow if true
only the content of the resource is deleted
* but not it's child resources. only supported for pages.
* @param autoSave if true
saves the modifications.
* @throws WCMException if an error during this operation occurs.
*/
void delete(Resource resource, boolean shallow, boolean autoSave) throws WCMException;
/**
* Deletes the resource.
* @param resource the resource to delete
* @param shallow if true
only the content of the resource is deleted
* but not it's child resources. only supported for pages.
* @param autoSave if true
saves the modifications.
* @param archive if true
saves the version for deleted resource.
* @throws WCMException if an error during this operation occurs.
*/
void delete(Resource resource, boolean shallow, boolean autoSave,boolean archive) throws WCMException;
/**
* Orders the given page before the one with the name specified by
* beforeName
if it's null
the page is ordered at
* the end of its siblings. Changes are automatically saved.
*
* @param page the page to order
* @param beforeName the name of the next page
* @throws WCMException if an error during this operation occurs.
*/
void order(Page page, String beforeName) throws WCMException;
/**
* Orders the given page before the one with the name specified by
* beforeName
if it's null
the page is ordered at
* the end of its siblings.
*
* @param page the page to order
* @param beforeName the name of the next page
* @param autoSave if true
saves the modifications.
* @throws WCMException if an error during this operation occurs.
*/
void order(Page page, String beforeName, boolean autoSave) throws WCMException;
/**
* Orders the given resource before the one with the name specified by
* beforeName
if it's null
the resource is ordered
* at the end of its siblings. Changes are automatically saved.
*
* @param resource the resource to order
* @param beforeName the name of the next resource
* @throws WCMException if an error during this operation occurs.
*/
void order(Resource resource, String beforeName) throws WCMException;
/**
* Orders the given resource before the one with the name specified by
* beforeName
if it's null
the resource is ordered
* at the end of its siblings.
*
* @param resource the resource to order
* @param beforeName the name of the next resource
* @param autoSave if true
saves the modifications.
* @throws WCMException if an error during this operation occurs.
*/
void order(Resource resource, String beforeName, boolean autoSave) throws WCMException;
/**
* Returns the template with the given name or null
if the template doesn't exist or
* the current user does not have read access on the template resource identified by the given path.
* This is the case on publish instances using default ACL configuration (anonymous cannot read templates).
* @param templatePath the name of the template
* @return the new template
* @deprecated Use {@link com.day.cq.wcm.api.TemplateManager#getTemplate(String)} instead.
*/
Template getTemplate(String templatePath);
/**
* Returns a collection of all available templates. If the given path
* is not null
only those templates are returned that are
* allowed to be used as page templates below that path.
*
* @param parentPath path of the parent page or null
* @return a collection of templates
* @see Template#isAllowed(String)
* @deprecated Use {@link com.day.cq.wcm.api.TemplateManager#getAllTemplates()} or
* {@link com.day.cq.wcm.api.TemplateManager#getTemplates(Predicate)} instead
*/
Collection getTemplates(String parentPath);
/**
* Returns a collection of all available site templates. If the given path
* is not null
only those templates are returned that are
* allowed to be used as page templates below that path.
*
* @param parentPath path of the parent page or null
* @return a collection of templates
* @see Template#isAllowed(String)
* @deprecated since 5.4 use {@link com.day.cq.wcm.msm.api.BlueprintManager#getBlueprints()}
*/
@SuppressWarnings({"JavadocReference"})
@Deprecated
Collection getBlueprints(String parentPath);
/**
* Create a revision of some page.
*
* @param page the page to create a revision for
* @return page revision or null
if no revision was created
* @throws WCMException if an error during this operation occurs.
*/
Revision createRevision(Page page) throws WCMException;
/**
* Create a revision of some page.
*
* @param page the page to create a revision for
* @param label the optional label for the revision. Note that the version label must be unique across all versions.
* @param comment the optional comment for the revision
* @return page revision or null
if no revision was created
* @throws WCMException if an error during this operation occurs.
* @since 5.2
*/
Revision createRevision(Page page, String label, String comment) throws WCMException;
/**
* Return all revisions of the page at the indicated path. Note that only those revisions are returned
* that have a parent path matching the parent of the indicated page.
*
* @param path the path to return revisions for
* @param cal optional calendar value; if not null
, return
* the revision of a page that has the latest creation date
* below or equal to this value
* @return Collection of revisions
* @throws WCMException if an error during this operation occurs.
*/
Collection getRevisions(String path, Calendar cal) throws WCMException;
/**
* Return all revisions of the page at the indicated path. If includeNoLocal
is true,
* revisions that have a prent path not matching the parent of the indicated page are included as well.
*
* @param path the path to return revisions for
* @param cal optional calendar value; if not null
, return the revision of a page that has the latest
* creation date below or equal to this value
* @param includeNoLocal if true
revisions that have a prent path not matching the parent of the
* indicated page are included as well
* @return Collection of revisions
* @throws WCMException if an error during this operation occurs.
*/
Collection getRevisions(String path, Calendar cal, boolean includeNoLocal) throws WCMException;
/**
* Return all revisions of child pages below the indicated path. Only those revisions are included that have a
* parent path matching the indicated parent path and that don't have an existing node other than below this parent.
*
* @param parentPath the path of the parent page
* @param cal optional calendar value; if not null
, return the revision of a page that has the latest
* creation date below or equal to this value
* @return Collection of revisions
* @throws WCMException if an error during this operation occurs.
*/
Collection getChildRevisions(String parentPath, Calendar cal) throws WCMException;
/**
* Return all revisions of child pages below the indicated path. Only those revisions are included that have a
* parent path matching the indicated parent path and that don't have an existing node other than below this parent.
* If includeNoLocal
is true, also those revisions are included that where not versioned below the
* indicated parent path.
*
* @param parentPath the path of the parent page
* @param cal optional calendar value; if not null
, return the revision of a page that has the latest
* creation date below or equal to this value
* @param includeNoLocal if true
revisions that have a prent path not matching the parent of the
* indicated page are included as well
* @return Collection of revisions
* @throws WCMException if an error during this operation occurs.
*/
Collection getChildRevisions(String parentPath, Calendar cal, boolean includeNoLocal) throws WCMException;
/**
* Return all revisions of child pages below the indicated path. For calculating the best latest revision older than
* cal
all revisions below the specified treeRoot
are respected. But only those revisions
* are returned that match the given parentPath
*
* @param parentPath the path of the parent page
* @param cal optional calendar value; if not null
, return the revision of a page that has the latest
* creation date below or equal to this value
* @param treeRoot root of the tree
* @return Collection of revisions
* @throws WCMException if an error during this operation occurs.
*/
Collection getChildRevisions(String parentPath, String treeRoot, Calendar cal) throws WCMException;
/**
* Restore a revision of a page. If a page content already exists for the specified revision id,
* its content is restored if the path
either addresses that page or its parent page, otherwise
* a WCMException is thrown.
* If the page does not exist then the a new page is created underneath the specified path
* and its content is restored accordingly. If there already exists a page with the same name as
* the one recorded in the version then a non-colliding name is generated.
*
* @param path path to the page or to the parent page
* @param revisionId revision id to restore
* @throws WCMException if an error during this operation occurs.
* @return the page that was restored
*/
public Page restore(String path, String revisionId) throws WCMException;
/**
* Restore a tree. Restores this page and its subtree to the versions of the
* given date.
*
* @param path path to page
* @param date calendar date to restore to
* @throws WCMException if an error during this operation occurs.
* @return the page that was restored
*/
public Page restoreTree(String path, Calendar date) throws WCMException;
/**
* Restore a tree. Restores this page and its subtree to the versions of the
* given date.
*
* @param path path to page
* @param date calendar date to restore to
* @param preserveNV if true
non versionable nodes are preserved
* @throws WCMException if an error during this operation occurs.
* @return the page that was restored
*/
public Page restoreTree(String path, Calendar date, boolean preserveNV) throws WCMException;
/**
* Touches the given page by setting the last modified date and user and
* clears the replication status. if now
is null
* the last modified status is not changed. if clearRepl
is
* false
the replication status is not changed.
*
* @param page the page to touch
* @param shallow if true
not recursion
* @param now current date
* @param clearRepl flag indicates if replication status is to be cleared
* @throws WCMException if an error occurs
*/
public void touch(Node page, boolean shallow, Calendar now, boolean clearRepl) throws WCMException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy