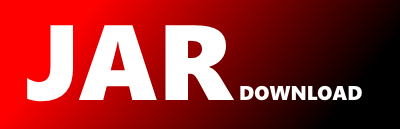
com.day.cq.wcm.api.PageModification Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2009 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.wcm.api;
import java.io.Serializable;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import org.osgi.service.event.Event;
/**
* This class encapsulates the information coming along with a page
* modification.
*/
public final class PageModification implements Serializable {
private static final String PROPERTY_CHANGES = "changes";
private static final String PROPERTY_VERSION_ID = "versionId";
private static final String PROPERTY_USER_ID = "userId";
private static final String PROPERTY_PATH = "path";
private static final String PROPERTY_DESTINATION = "destination";
private static final String PROPERTY_ABOVE = "above";
private static final String PROPERTY_MODIFIED_DATE = "modifiedDate";
private static final String PROPERTY_TYPE = "type";
/**
* The modification type.
*/
public static enum ModificationType {
/**
* page was created
*/
CREATED("PageCreated"),
/**
* page was modified
*/
MODIFIED("PageModified"),
/**
* page was moved
*/
MOVED("PageMoved"),
/**
* page was deleted
*/
DELETED("PageDeleted"),
/**
* page was versioned
*/
VERSION_CREATED("VersionCreated"),
/**
* page was restored
*/
RESTORED("PageRestored"),
/**
* page rolled out
*/
ROLLEDOUT("PageRolledOut"),
/**
* page became valid (on time reached)
*/
VALID("PageValid"),
/**
* page became invalid (onff time reached)
*/
INVALID("PageInvalid");
private final String name;
ModificationType(String name) {
this.name = name;
}
/**
* @see java.lang.Enum#toString()
*/
public String toString() {
return name;
}
public static ModificationType fromName(String n) {
for (ModificationType m : ModificationType.values()) {
if (m.toString().equals(n)) {
return m;
}
}
throw new IllegalArgumentException("Unknown modification type: " + n);
}
}
/**
* Path of page that changed
*/
private final String path;
/**
* Destination hande for move, may be null
if this was a
* simple reordering inside the same hierarchy
*/
private final String destination;
/**
* above path (used in create/move messages)
*/
private final String above;
/**
* Modification type.
*/
private final ModificationType type;
/**
* The version of the page that this modification belongs to.
*/
private final String versionId;
/**
* The user object of this page modification.
*/
private final String userId;
/**
* The modification date.
*/
private final Date modificationDate;
/**
* A list of changes.
*/
private final Set changes;
/**
* Create a new PageModification
that represents
* a page creation.
*
* @param path path to new page
* @param above label of successor sibling
* @param userId the id of the user that caused this modification.
* @return modification
*/
public static PageModification created(String path, String above, String userId) {
return new PageModification(ModificationType.CREATED,
path, null, null, above, userId, null, null);
}
/**
* Create a new PageModification
that represents
* a page modification.
*
* @param path path of page
* @param vid the version name
* @param userId the id of the user that caused this modification.
* @param changes A set of changes (paths) - optional
* @return modification
*/
public static PageModification modified(String path,
String vid,
String userId,
Set changes) {
return new PageModification(ModificationType.MODIFIED,
path, vid, null, null, userId, null, changes);
}
/**
* Create a new PageModification
that represents
* a page moval.
*
* @param path path of page
* @param destination new page destination, may be null
* @param above label of successor sibling, may be null
* @param userId the id of the user that caused this modification.
* @return modification
*/
public static PageModification moved(String path,
String destination, String above, String userId) {
return new PageModification(ModificationType.MOVED,
path, null, destination, above, userId, null, null);
}
/**
* Create a new PageModification
that represents
* a page deletion.
*
* @param path path of page
* @param userId the id of the user that caused this modification.
* @return modification
*/
public static PageModification deleted(String path,
String userId) {
return new PageModification(ModificationType.DELETED,
path, null, null, null, userId, null, null);
}
/**
* Create a new PageModification
that represents
* a version created.
*
* @param path path of page
* @param vid the versio name
* @param userId the id of the user that caused this modification.
* @return modification
*/
public static PageModification versionCreated(String path,
String vid, String userId) {
return new PageModification(ModificationType.VERSION_CREATED,
path, vid, null, null, userId, null, null);
}
/**
* Create a new PageModification
that represents
* a version created.
*
* @param path path of page
* @param vid the vesion name
* @param userId the id of the user that caused this modification.
* @return modification
*/
public static PageModification pageRestored(String path,
String vid, String userId) {
return new PageModification(ModificationType.RESTORED,
path, vid, null, null, userId, null, null);
}
/**
* Create a new PageModification
that represents
* that the page became valid due to an on-time reached.
*
* @param path path of page
* @param time the on-time
* @return modification
*/
public static PageModification pageValid(String path, long time) {
return new PageModification(ModificationType.VALID,
path, null, null, null, null, new Date(time), null);
}
/**
* Create a new PageModification
that represents
* that the page became invalid due to an off-time reached.
*
* @param path path of page
* @param time the off-time
* @return modification
*/
public static PageModification pageInvalid(String path, long time) {
return new PageModification(ModificationType.INVALID,
path, null, null, null, null, new Date(time), null);
}
/**
* Create a new PageModification
that represents
* a version deleted.
*
* @param path path of page
* @param userId the id of the user that caused this modification.
* @return modification
*/
public static PageModification rolledout(String path,
String userId) {
return new PageModification(ModificationType.ROLLEDOUT,
path, null, null, null, userId, null, null);
}
/**
* Create a new PageModification
. Internal method called
* by one of the static factory methods {@link #created},
* {@link #modified}, {@link #moved}, {@link #deleted} or
* {@link #versionCreated}
*
* @param type modification type
* @param path path of the page; must not be null
* @param versionid The versionid for the modification
* @param destination destination in case of move operations, otherwise
* null
* @param above path above in case of create/move operations, otherwise
* null
* @param userId the id of the user that caused this modification.
* @param md the modification date. if null
the current date is used
* @param changes A set of changes (paths) - optional
*/
private PageModification(ModificationType type,
String path,
String versionid,
String destination,
String above,
String userId,
Date md,
Set changes) {
this.type = type;
this.path = path;
this.versionId = versionid;
this.destination = destination;
this.above = above;
this.userId = userId == null ? "admin" : userId;
this.modificationDate = (md == null ? new Date() : md);
this.changes = changes;
}
/**
* The path of the page.
*
* @return The path of the page.
*/
public String getPath() {
return path;
}
public String getDestination() {
return destination;
}
public String getAbove() {
return above;
}
public ModificationType getType() {
return type;
}
public String getVersionId() {
return versionId;
}
public String getUserId() {
return userId;
}
public Date getModificationDate() {
return modificationDate;
}
/**
* Get the list of changes for a modification of the page.
*
* @return A set of relative paths or null.
*/
public Set getModificationPaths() {
return changes;
}
/**
* Overridden to alter the preconditions when two modifications are
* considered equal.
*
* @param o object to compare this object against
* @return true
if this object is considered equal to the
* other object, otherwise false
*/
public final boolean equals(Object o) {
if (o instanceof PageModification) {
PageModification other = (PageModification) o;
return other.path.equals(path) &&
other.destination.equals(destination) &&
other.type == type;
}
return false;
}
/**
* Returns a hash code value for the object. Replaced in order to
* return the same hashcode for two objects that are considered
* equal according to the {@link #equals} method implemented above.
*
* @return a hash code value for this object.
* @see java.lang.Object#equals(java.lang.Object)
* @see java.util.Hashtable
*/
public int hashCode() {
int hashCode = path.hashCode() ^ type.hashCode();
if (destination != null) {
hashCode ^= destination.hashCode();
}
return hashCode;
}
/**
* Return a string representation of this object
*
* @return string representation
*/
public String toString() {
StringBuffer b = new StringBuffer();
b.append("page ");
switch (type) {
case CREATED:
b.append("created");
break;
case MODIFIED:
b.append("modified");
break;
case MOVED:
b.append("moved");
break;
case DELETED:
b.append("deleted");
break;
case VERSION_CREATED:
b.append("version created");
break;
case RESTORED:
b.append("version restored");
break;
case ROLLEDOUT:
b.append("rolled out");
break;
case VALID:
b.append("valid");
break;
case INVALID:
b.append("invalid");
break;
}
b.append(": ");
b.append(path);
if (destination != null) {
b.append(" --> ");
b.append(destination);
}
if (above != null) {
b.append(", above: ");
b.append(above);
}
if (versionId != null) {
b.append(", vid: ");
b.append(versionId);
}
if (userId != null) {
b.append(", userId: ");
b.append(userId);
}
return b.toString();
}
/**
* Get a dictionary with all event properties.
*
* @return an map of event properties
*/
public Map getEventProperties() {
final Map properties = new HashMap();
properties.put(PROPERTY_TYPE, type.toString());
properties.put(PROPERTY_MODIFIED_DATE, modificationDate);
if (above != null) {
properties.put(PROPERTY_ABOVE, above);
}
if (destination != null) {
properties.put(PROPERTY_DESTINATION, destination);
}
if (path != null) {
properties.put(PROPERTY_PATH, path);
}
if (userId != null) {
properties.put(PROPERTY_USER_ID, userId);
}
if (versionId != null) {
properties.put(PROPERTY_VERSION_ID, versionId);
}
if (changes != null) {
properties.put(PROPERTY_CHANGES, changes);
}
return properties;
}
/**
* Create a page modification from properties.
*
* @param props the event properties
* @return a page modification object
*/
@SuppressWarnings("unchecked")
public static PageModification fromEventProperties(Map props) {
return new PageModification(
ModificationType.fromName((String) props.get(PROPERTY_TYPE)),
(String) props.get(PROPERTY_PATH),
(String) props.get(PROPERTY_VERSION_ID),
(String) props.get(PROPERTY_DESTINATION),
(String) props.get(PROPERTY_ABOVE),
(String) props.get(PROPERTY_USER_ID),
(Date) props.get(PROPERTY_MODIFIED_DATE),
(Set) props.get(PROPERTY_CHANGES));
}
/**
* Convert a single modification to an event.
*
* @return a page event
*/
public Event toEvent() {
return new PageEvent(this).toEvent();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy