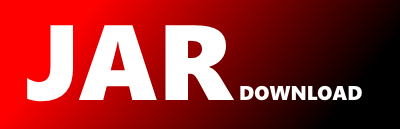
com.day.cq.wcm.api.components.EditContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2008 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.wcm.api.components;
import java.io.IOException;
import javax.servlet.ServletException;
import org.apache.sling.api.SlingHttpServletRequest;
import org.apache.sling.api.SlingHttpServletResponse;
import com.day.cq.wcm.api.WCMMode;
/**
* The edit context is used to define the edit behaviour of components. There is
* a context opened for every included component that can be edited (or designed).
* A component can be edited if it provides a {@link EditConfig}.
*/
public interface EditContext {
/**
* Checks if this is the root edit context.
* @return true
if this is the root edit context
*/
boolean isRoot();
/**
* Returns the parent edit context or null
if this context
* is the root context.
* @return the parent context or null
*/
EditContext getParent();
/**
* Returns the root edit context.
* @return the root edit context.
*/
EditContext getRoot();
/**
* Returns the primary component context that is bound to this edit context.
* Note that en edit context can span several component context if components
* are included that do not need editing.
*
* @return the component context.
*/
ComponentContext getComponentContext();
/**
* Returns the primary edit component for this context.
* @return the edit component.
*/
Component getComponent();
/**
* Retrieves the edit config for the current component respecting the
* context hierarchy. This might not be the same as the one returned by
* {@link Component#getEditConfig() getComponent().getEditConfig()} since it
* can be merged with the child configs of its parents.
*
* @return the edit config
*/
EditConfig getEditConfig();
/**
* Returns the context attribute for the given name
* @param name the name of the attribute
* @return the attribute or null
if not defined.
*/
Object getAttribute(String name);
/**
* Sets the content path that is used when including the java script call
* for the edit bars. defaults to the path of the resource in edit mode or
* to the path of the style in design mode.
*
* @param path content path
*/
void setContentPath(String path);
/**
* Sets a context attribute with the given name. If the given attribute is
* null
it is removed from the attribute map.
* @param name the name of the attribute
* @param value the attribute value
* @return the old attribute or null
*/
Object setAttribute(String name, Object value);
/**
* Includes the html prolog needed for editing
*
* @param req the request
* @param resp the response
* @param mode the wcm mode
* @throws IOException if an I/O error occurs
* @throws ServletException if a servlet error occurs
*/
void includeProlog(SlingHttpServletRequest req,
SlingHttpServletResponse resp,
WCMMode mode)
throws IOException, ServletException;
/**
* Includes the html epilog of this context into the response.
*
* @param req the request
* @param resp the response
* @param mode the wcm mode
* @throws IOException if an I/O error occurs
* @throws ServletException if a servlet error occurs
*/
void includeEpilog(SlingHttpServletRequest req,
SlingHttpServletResponse resp,
WCMMode mode)
throws IOException, ServletException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy