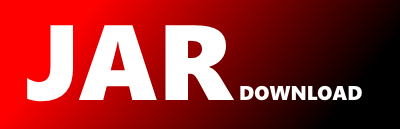
com.day.cq.wcm.api.msm.RolloutManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2009 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.wcm.api.msm;
import javax.jcr.Node;
import javax.jcr.RepositoryException;
import org.apache.sling.api.resource.ResourceResolver;
import com.day.cq.wcm.api.Page;
import com.day.cq.wcm.api.WCMException;
/**
* Provides a service for managing MSM rollouts.
* @deprecated since 5.3 use {@link com.day.cq.wcm.msm.api.RolloutManager} instead
*/
@Deprecated
public interface RolloutManager {
/**
* Trigger type that defines when a rollout should happen.
*/
public static enum Trigger {
/**
* never trigger a rollout (probbly only used for pull scenarios)
*/
NEVER("never"),
/**
* auto rollout on modification
*/
MODIFICATION("modification"),
/**
* auto rollout on activation
*/
PUBLICATION("publish"),
/**
* rollout on explicit user rollouts
*/
ROLLOUT("rollout");
private final String name;
Trigger(String name) {
this.name = name;
}
public String toString() {
return this.name;
}
public static Trigger fromName(String n) {
for (Trigger m : Trigger.values()) {
if (m.toString().equals(n)) {
return m;
}
}
throw new IllegalArgumentException("Unknown trigger type: " + n);
}
}
/**
* Execute a rollout on all the found live copies of the master
page.
*
* @param master master page to rollout
* @param trigger rollout trigger
* @param targets paths of live copies to update. null
for all.
* @param isDeep if true
all child pages are updated, too.
* @throws WCMException if an error during this operation occurs.
*/
void rollout(Page master, boolean isDeep, Trigger trigger, String[] targets)
throws WCMException;
/**
* Execute a rollout on all the found live copies of the master
page.
*
* @param master master page to rollout
* @param trigger rollout trigger
* @param reset if true
rollout is run in reset mode, Live Copy is completely reset
* @param targets paths of live copies to update. null
for all.
* @param isDeep if true
all child pages are updated, too.
* @throws WCMException if an error during this operation occurs.
*/
void rollout(Page master, boolean isDeep, Trigger trigger, boolean reset, String[] targets)
throws WCMException;
/**
* Execute a rollout of the paragraph
to all live copies of
* the of master
page.
*
* @param master Master page to rollout
* @param paragraphs (absolute) paths of paragraphs
* @param trigger rollout trigger
* @param targets paths of live copies to update. null
for all.
* @throws WCMException if an error during this operation occurs.
*/
void rollout(Page master, Trigger trigger, String[] targets,
String[] paragraphs)
throws WCMException;
/**
* Execute a rollout of the paragraph
to all live copies of
* the of master
page.
*
* @param master Master page to rollout
* @param trigger rollout trigger
* @param reset if true
rollout is run in reset mode, Live Copy is completely reset
* @param targets paths of live copies to update. null
for all.
* @param paragraphs (absolute) paths of paragraphs
* @throws WCMException if an error during this operation occurs.
*/
void rollout(Page master, Trigger trigger, boolean reset, String[] targets,
String[] paragraphs)
throws WCMException;
/**
* Execute a rollout of the paragraph
to all live copies of
* the of master
page. If delete
is true
,
* the paragraph is deleted and then rolledout.
*
* @param master Master page to rollout
* @param paragraphs (absolute) paths of paragraphs
* @param trigger rollout trigger
* @param targets paths of live copies to update. null
for all.
* @param delete true
if paragraph should be deleted
* @throws WCMException if an error during this operation occurs.
*/
void rollout(Page master, Trigger trigger, String[] targets,
String[] paragraphs, boolean delete)
throws WCMException;
/**
* Execute a rollout of the paragraph
to all live copies of
* the of master
page. If delete
is true
,
* the paragraph is deleted and then rolledout.
*
* @param master Master page to rollout
* @param paragraphs (absolute) paths of paragraphs
* @param trigger rollout trigger
* @param reset if true
rollout is run in reset mode, Live Copy is completely reset
* @param targets paths of live copies to update. null
for all.
* @param delete true
if paragraph should be deleted
* @throws WCMException if an error during this operation occurs.
*/
void rollout(Page master, Trigger trigger, boolean reset, String[] targets,
String[] paragraphs, boolean delete)
throws WCMException;
/**
* Rollout the content for one relation ship.
* @param resolver resource resolver
* @param relation relation to rollout
* @throws WCMException if an error during this operation occurs.
*/
void rollout(ResourceResolver resolver, LiveRelationship relation)
throws WCMException;
/**
* Rollout the content for one relation ship.
* @param resolver resource resolver
* @param relation relation to rollout
* @param reset if true
rollout is run in reset mode, Live Copy is completely reset
* @throws WCMException if an error during this operation occurs.
*/
void rollout(ResourceResolver resolver, LiveRelationship relation, boolean reset)
throws WCMException;
/**
* Update rollout info on the node
. To use after a rollout operation.
*
* @param node Node to update
* @param deepUpdate Children of the node can be updated by setting
* deepUpdate
to true.
* @param autoSave Save modifications
* @throws WCMException if an error during this operation occurs.
*/
void updateRolloutInfo(Node node, boolean deepUpdate, boolean autoSave) throws WCMException;
/**
* Returns if a property is defined as excluded in the RolloutManager
configuration.
* Excluded properties include reserved properties.
* @param propertyName repository property name.
* @return true if excluded. False otherwise.
* @deprecated Use #isExcludedPageProperty instead.
*/
boolean isExcludedProperty(String propertyName);
/**
* Returns if a property is defined as excluded in the RolloutManager
configuration.
* If isPage
is true, checks in page exclusion list. Otherwise, check in paragraph exclusion list
* Excluded properties include reserved properties.
* @param isPage Page property
* @param propertyName repository property name.
* @return true if excluded. False otherwise.
*/
boolean isExcludedProperty(boolean isPage, String propertyName);
/**
* Returns if a property is defined as excluded in the RolloutManager
configuration for a page.
* Excluded properties include reserved properties.
* @param propertyName repository property name.
* @return true if excluded. False otherwise.
*/
boolean isExcludedPageProperty(String propertyName);
/**
* Returns if a property is defined as excluded in the RolloutManager
configuration for a paragraph.
* Excluded properties include reserved properties.
* @param propertyName repository property name.
* @return true if excluded. False otherwise.
*/
boolean isExcludedParagraphProperty(String propertyName);
/**
* Returns if a node type is defined as excluded in the RolloutManager
configuration
* @param nodeType repository node type.
* @return true if excluded. False otherwise.
*/
boolean isExcludedNodeType(String nodeType);
/**
* Returns if one of the node types or mixins is defined as excluded in the RolloutManager
configuration
* @param node node to check.
* @return true if excluded. False otherwise.
@throws RepositoryException if an read/write error during this operation occurs.
*/
boolean isExcludedNode(Node node) throws RepositoryException;
/**
* Returns if a property is a MSM reserved property
* @param propertyName repository property name.
* @return true if reserved. False otherwise.
*/
boolean isReservedProperty(String propertyName);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy