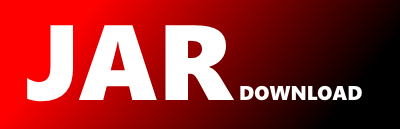
com.day.cq.wcm.command.api.CopyMoveCommandBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2017 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
* **************************************************************************/
package com.day.cq.wcm.command.api;
import com.adobe.cq.dam.cfm.extensions.ContentFragmentReferenceResolver;
import com.adobe.cq.wcm.launches.cf.ContentFragmentLaunchManager;
import com.day.cq.dam.api.AssetReferenceResolver;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import org.apache.sling.api.resource.ResourceResolver;
import org.osgi.annotation.versioning.ProviderType;
/**
* builder interface for the copy/move command
*/
@ProviderType
public interface CopyMoveCommandBuilder extends CommandBuilder {
/**
* initializes the {@code ResourceResolver} for the command builder
*
* @param resourceResolver the resource resolver for the command builder
* @return this {@code CopyMoveCommandBuilder}
*/
@Nonnull
CopyMoveCommandBuilder withResourceResolver(@Nonnull ResourceResolver resourceResolver);
/**
* sets the reference resolver to access references
*
* @param assetRefResolver the {@code AssetReferenceResolver} to get references
* @return this {@code CopyMoveCommandBuilder}
*/
@Nonnull
CopyMoveCommandBuilder withAssetReferenceResolver(@Nonnull AssetReferenceResolver assetRefResolver);
/**
* sets the content fragment reference resolver to access references
*
* @param contentFragmentReferenceResolver the {@code ContentFragmentReferenceResolver} to get references
* @return this {@code CopyMoveCommandBuilder}
*/
@Nonnull
CopyMoveCommandBuilder withContentFragmentReferenceResolver(@Nonnull ContentFragmentReferenceResolver contentFragmentReferenceResolver);
@Nonnull
CopyMoveCommandBuilder withContentFragmentLaunchManager(@Nonnull ContentFragmentLaunchManager cfLaunchManager);
/**
* sets if the command is copy command or move command
*
* @param copy true if copy command, false if move command
* @return this {@code CopyMoveCommandBuilder}
*/
@Nonnull
CopyMoveCommandBuilder withCopy(boolean copy);
/**
* sets name of the next resource
*
* @param beforeName the name of the next resource. if null the resource is ordered at the end.
* @return this {@code CopyMoveCommandBuilder}
*/
@Nonnull
CopyMoveCommandBuilder withBeforeName(@Nullable String beforeName);
/**
* sets whether to perform a shallow operation (this is currently only supported for pages)
*
* @param shallow if true only the resource content is moved.
* @return this {@code CopyMoveCommandBuilder}
*/
@Nonnull
CopyMoveCommandBuilder withShallow(boolean shallow);
/**
* sets whether to do to integrity checking while performing the command
*
* @param integrity if true it is checked if the pages on the src paths can
* be moved to the destinations without conflicting the
* integrity. the integrity is conflicted if
*
* - the source page (or any child pages) are 'activated'
* - any reference to the page (or any child page) is 'activated'
*
* @return this {@code CopyMoveCommandBuilder}
*/
@Nonnull
CopyMoveCommandBuilder withCheckIntegrity(boolean integrity);
/**
* sets whether to fetch references while performing the command
*
* @param retrieveAll if true, fetch references on server ideally when the number of items is very large.
* @return this {@code CopyMoveCommandBuilder}
*/
@Nonnull
CopyMoveCommandBuilder withRetrieveAllRefs(boolean retrieveAll);
/**
* @return a new path argument builder
*/
@Nonnull
CopyMoveCommandPathArgumentBuilder createPathArgumentBuilder();
/**
* append a path argument
*
* @param pathArgument the path argument instance
* @return this {@code CopyMoveCommandBuilder}
*/
@Nonnull
CopyMoveCommandBuilder withPathArgument(@Nonnull CopyMoveCommandPathArgument pathArgument);
/**
* updates the title (if provided) of the moved resource
*
* @param destTitle new title of the resource at destination
* @return this {@code CopyMoveCommandBuilder}
*/
@Nonnull
CopyMoveCommandBuilder withTitleUpdate(String destTitle);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy