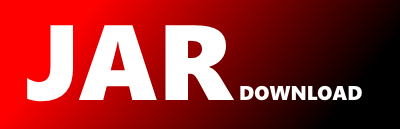
com.day.cq.wcm.foundation.Table Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2008 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.wcm.foundation;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
import java.io.Writer;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.apache.commons.lang3.StringEscapeUtils;
/**
* Implements a very basic table parser.
*/
public class Table {
private LinkedList rows = new LinkedList();
private LinkedList cols = new LinkedList();
private Cell[][] data = new Cell[8][8];
private Tag caption;
private List colTags = new LinkedList();
private Attributes attributes = new Attributes();
public List getColTags() {
return colTags;
}
public Attributes getAttributes() {
return attributes;
}
public Table setAttribute(String name, String value) {
if (value == null) {
attributes.remove(name);
} else {
attributes.put(name, value);
}
return this;
}
public Tag getCaption() {
return caption;
}
public Tag setCaption(String text) {
if (caption == null) {
caption = new Tag("caption");
}
caption.setInnerHtml(text);
return caption;
}
public Cell getCell(int row, int col, boolean create) {
Cell cell = null;
if (row >= rows.size() || col >= cols.size()) {
if (create) {
setSize(Math.max(row + 1, rows.size()), Math.max(col + 1, cols.size()));
cell = data[row][col];
}
} else {
cell = data[row][col];
}
return cell;
}
public int[][] getIntData(int rowStart, int numRows, int colStart, int numCols) {
if (rowStart + numRows > rows.size()) {
numRows = rows.size() - rowStart;
}
if (colStart + numCols > cols.size()) {
numCols = cols.size() - colStart;
}
int[][] ret = new int[numRows][numCols];
for (int r=0; r rows.size()) {
numRows = rows.size() - rowStart;
}
if (colStart + numCols > cols.size()) {
numCols = cols.size() - colStart;
}
double[][] ret = new double[numRows][numCols];
for (int r=0; r= data.length || numCols >= data[0].length) {
Cell[][] newData = new Cell[data.length*2][data[0].length*2];
for (int r=0; r numRows) {
rows.removeLast();
}
while (cols.size() < numCols) {
Column col = new Column(cols.size());
cols.add(col);
for (Row row : rows) {
data[row.nr][col.nr] = new Cell(row, col);
}
}
while (cols.size() > numCols) {
cols.removeLast();
}
}
public List getRows() {
return Collections.unmodifiableList(rows);
}
public List getColumns() {
return Collections.unmodifiableList(cols);
}
public Row getRow(int nr) {
return nr < rows.size() ? null : rows.get(nr);
}
public Column getColumn(int nr) {
return nr < cols.size() ? null : cols.get(nr);
}
public int getNumCols() {
return cols.size();
}
public int getNumRows() {
return rows.size();
}
public void clear() {
rows.clear();
cols.clear();
}
public static Table fromXML(String s) {
try {
return fromXML(new StringReader(s));
} catch (IOException e) {
throw new IllegalStateException(e);
}
}
public static Table fromXML(Reader r) throws IOException {
return new TableXMLBuilder().parse(r);
}
public static Table fromCSV(String s) {
return new TableCSVBuilder().parse(s);
}
public static Table fromCSV(String s, char delim) {
return new TableCSVBuilder(delim).parse(s);
}
public class Row {
private int nr;
private Row(int nr) {
this.nr = nr;
}
public int getNr() {
return nr;
}
public boolean isFirst() {
return nr == 0;
}
public boolean isLast() {
return nr == rows.size() - 1;
}
public Table getTable() {
return Table.this;
}
public List getCells() {
// currently just create a new list. optimize later with own class.
List cells = new ArrayList(cols.size());
for (int c=0; c getCells() {
// currently just create a new list. optimize later with own class.
List cells = new ArrayList(rows.size());
for (int r=0; r 1) {
attributes.put("colspan", String.valueOf(this.colSpan));
} else {
attributes.remove("colspan");
}
return this;
}
public int getRowSpan() {
return rowSpan;
}
public Cell setRowSpan(int rowSpan) {
if (rowSpan == 0) {
rowSpan = 1;
}
internalSetSpans(rowSpan, colSpan);
if (attributes == null) {
attributes = new Attributes();
}
if (rowSpan > 1) {
attributes.put("rowspan", String.valueOf(this.rowSpan));
} else {
attributes.remove("rowspan");
}
return this;
}
private void internalSetSpans(int rSpan, int cSpan) {
Table table = getTable();
int rowNr = row.getNr();
int colNr = col.getNr();
// clear old spans
for (int r = 0; r 0) {
table.getCell(rowNr + r, colNr + c, true).spanSource = this;
}
}
}
}
public Cell setText(CharSequence text) {
this.text = text.toString();
return this;
}
public boolean isHeader() {
return header;
}
public Cell setHeader(boolean header) {
this.header = header;
return this;
}
public String getAttribute(String name) {
if (attributes == null) {
return null;
} else {
return attributes.get(name);
}
}
public Cell setAttribute(String name, String value) {
if (attributes == null) {
attributes = new Attributes();
}
attributes.put(name, value);
if (name.equalsIgnoreCase("colspan")) {
setColSpan(Integer.parseInt(value));
} else if (name.equalsIgnoreCase("rowspan")) {
setRowSpan(Integer.parseInt(value));
}
return this;
}
public Cell clearAttributes() {
attributes = null;
internalSetSpans(1, 1);
return this;
}
public Map getAttributes() {
if (attributes == null) {
return Collections.emptyMap();
} else {
return Collections.unmodifiableMap(attributes);
}
}
public void toHtml(Writer out) throws IOException {
String tag = header ? "th" : "td";
out.write("<");
out.write(tag);
if (attributes != null) {
attributes.toHtml(out);
}
out.write(">");
if (text != null) {
out.write(text);
}
out.write("");
out.write(tag);
out.write(">");
}
}
public static class Tag {
protected final String name;
protected final Attributes attrs = new Attributes();
protected String innerHtml;
public Tag(String name) {
this.name = name;
}
public String getName() {
return name;
}
public String getInnerHtml() {
return innerHtml;
}
public Tag setInnerHtml(String innerHtml) {
this.innerHtml = innerHtml;
return this;
}
public Tag appendInnerHtml(char[] ch, int offset, int len) {
if (innerHtml == null) {
innerHtml = new String(ch, offset, len);
} else {
innerHtml = innerHtml + new String(ch, offset, len);
}
return this;
}
public Attributes getAttributes() {
return attrs;
}
public Tag setAttribute(String name, String value) {
if (value == null) {
attrs.remove(name);
} else {
attrs.put(name, value);
}
return this;
}
public void toHtml(Writer out) throws IOException {
out.write("<");
out.write(name);
if (attrs != null) {
attrs.toHtml(out);
}
out.write(">");
if (innerHtml != null) {
out.write(innerHtml);
out.write("");
out.write(name);
out.write(">");
}
}
}
public static class ColTag extends Tag {
public ColTag() {
super("col");
}
public Tag setInnerHtml(String innerHtml) {
// ignore
return this;
}
}
public static class Attributes extends HashMap {
public void toHtml(Writer out) throws IOException {
for (Map.Entry e: entrySet()) {
out.write(" ");
out.write(e.getKey());
out.write("=\"");
out.write(StringEscapeUtils.escapeHtml4(e.getValue()));
out.write("\"");
}
}
}
public void toHtml(Writer out) throws IOException {
out.write("\n");
if (caption != null) {
caption.toHtml(out);
out.write("\n");
}
for (Tag cg: colTags) {
cg.toHtml(out);
out.write("\n");
}
for (Row row: rows) {
out.write("");
for (Column col: cols) {
Cell cell = data[row.nr][col.nr];
if (cell.getSpanSource() == null) {
data[row.nr][col.nr].toHtml(out);
}
}
out.write(" \n");
}
out.write(" \n");
}
} | | | | |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy