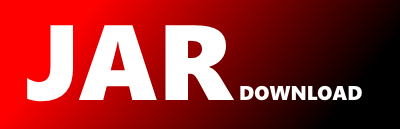
com.day.cq.wcm.foundation.forms.FormsHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2010 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.wcm.foundation.forms;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.UnsupportedEncodingException;
import java.io.Writer;
import java.net.URLDecoder;
import java.net.URLEncoder;
import java.security.AccessControlException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.ResourceBundle;
import javax.jcr.Node;
import javax.jcr.NodeIterator;
import javax.jcr.RepositoryException;
import javax.jcr.Session;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.jsp.JspWriter;
import javax.servlet.jsp.PageContext;
import org.apache.commons.lang.StringUtils;
import org.apache.commons.lang3.StringEscapeUtils;
import org.apache.sling.api.SlingHttpServletRequest;
import org.apache.sling.api.SlingHttpServletResponse;
import org.apache.sling.api.request.RequestDispatcherOptions;
import org.apache.sling.api.request.RequestParameter;
import org.apache.sling.api.request.RequestParameterMap;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ResourceResolver;
import org.apache.sling.api.resource.ResourceUtil;
import org.apache.sling.api.resource.ValueMap;
import org.apache.sling.api.scripting.SlingBindings;
import org.apache.sling.api.scripting.SlingScriptHelper;
import org.apache.sling.commons.json.JSONException;
import org.apache.sling.commons.json.jcr.JsonItemWriter;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.adobe.granite.xss.XSSAPI;
import com.day.cq.commons.jcr.JcrConstants;
import com.day.cq.wcm.api.LanguageManager;
import com.day.cq.wcm.api.WCMMode;
import com.day.cq.wcm.api.components.ComponentContext;
import com.day.cq.wcm.foundation.ELEvaluator;
import com.day.cq.wcm.foundation.forms.impl.FormChooserServlet;
import com.day.cq.wcm.foundation.forms.impl.FormStructureHelperImpl;
import com.day.cq.wcm.foundation.forms.impl.FormsUtil;
import com.day.cq.wcm.foundation.forms.impl.JspSlingHttpServletResponseWrapper;
import com.day.cq.wcm.foundation.forms.impl.ResourceWrapper;
/**
* Helper class for the forms components.
*/
public class FormsHelper {
/** The logger. */
private static final Logger LOGGER = LoggerFactory.getLogger(FormsHelper.class.getName());
private FormsHelper() {
// no instances
}
public static final String REQ_ATTR_GLOBAL_LOAD_MAP = "cq.form.loadmap";
public static final String REQ_ATTR_GLOBAL_LOAD_RESOURCE = "cq.form.loadresource";
public static final String REQ_ATTR_EDIT_RESOURCES = FormResourceEdit.RESOURCES_ATTRIBUTE;
public static final String REQ_ATTR_CLIENT_VALIDATION = "cq.form.clientvalidation";
public static final String REQ_ATTR_FORMID = "cq.form.id";
public static final String REQ_ATTR_WRITTEN_JAVASCRIPT = "cq.form.javascript";
public static final String REQ_ATTR_ACTION_SUFFIX = "cq.form.action.suffix";
public static final String REQ_ATTR_FORWARD_PATH = "cq.form.forward.path";
public static final String REQ_ATTR_FORWARD_OPTIONS = "cq.form.forward.options";
public static final String REQ_ATTR_IS_INIT = "cq.form.init";
public static final String REQ_ATTR_READ_ONLY = "cq.form.readonly";
public static final String REQ_ATTR_REDIRECT = "cq.form.redirect";
public static final String REQ_ATTR_REDIRECT_TO_REFERRER = FormsConstants.REQUEST_ATTR_REDIRECT_TO_REFERRER;
public static final String REQ_ATTR_PROP_WHITELIST = "cq.form.prop.whitelist";
public static final String REQ_ATTR_EXPRESSIONS_ENABLED = "cq.form.expressions.enabled";
public static final String REQ_ATTR_FORM_STRUCTURE_HELPER = "cq.form.formstructurehelper";
private static final FormStructureHelper defaultFormStructureHelper = new FormStructureHelperImpl();
/**
* Signal the start of the form.
* Prepare the request object, write out the client javascript (if the form
* is configured accordingly) and write out the start form tag and hidden fields:
* {@link FormsConstants#REQUEST_PROPERTY_FORMID} with the value of the form id.
* {@link FormsConstants#REQUEST_PROPERTY_FORM_START} with the relative path to the form start par
* and _charset_
with the value UTF-8
*
* @param request The current request.
* @param response The current response.
* @param out The jsp writer.
* @deprecated Use {@link #startForm(SlingHttpServletRequest, SlingHttpServletResponse)}
* @throws IOException if form generation caused an error
* @throws ServletException if form generation caused an error
*/
@Deprecated
public static void startForm(final SlingHttpServletRequest request,
final SlingHttpServletResponse response,
final JspWriter out)
throws IOException, ServletException {
startForm(request, new JspSlingHttpServletResponseWrapper(response, out));
}
/**
* Signal the start of the form.
* Prepare the request object, write out the client javascript (if the form
* is configured accordingly) and write out the start form tag and hidden fields:
* {@link FormsConstants#REQUEST_PROPERTY_FORMID} with the value of the form id.
* {@link FormsConstants#REQUEST_PROPERTY_FORM_START} with the relative path to the form start par
* and _charset_
with the value UTF-8
*
* @param request The current request.
* @param response The current response.
* @since 5.3
* @throws IOException if form generation caused an error
* @throws ServletException if form generation caused an error
*/
public static void startForm(final SlingHttpServletRequest request,
final SlingHttpServletResponse response)
throws IOException, ServletException {
// get resource and properties
final Resource formResource = request.getResource();
initialize(request, formResource, response);
final ValueMap properties = ResourceUtil.getValueMap(formResource);
String formId = properties.get("id", "");
if(StringUtils.isEmpty(formId))
formId = getFormId(request);
// write form element, we post to the same url we came from
final PrintWriter out = response.getWriter();
String url = request.getRequestURI();
final String suffix = getActionSuffix(request);
if (StringUtils.isNotBlank(suffix)) {
url += (suffix.startsWith("/")) ? suffix : "/" + suffix;
}
SlingBindings bindings = (SlingBindings) request.getAttribute(SlingBindings.class.getName());
XSSAPI xssAPI = bindings.getSling().getService(XSSAPI.class).getRequestSpecificAPI(request);
String cssClass = properties.get("css", "");
out.print("
© 2015 - 2025 Weber Informatics LLC | Privacy Policy