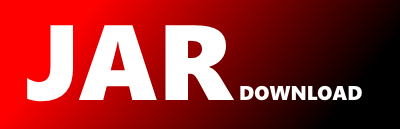
com.day.cq.wcm.foundation.model.responsivegrid.ResponsiveGridUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2020 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.wcm.foundation.model.responsivegrid;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Function;
import java.util.stream.Collectors;
import static com.day.cq.wcm.foundation.model.responsivegrid.ResponsiveConstants.BREAKPOINT_VARIANT_NAME_DEFAULT;
import static com.day.cq.wcm.foundation.model.responsivegrid.ResponsiveConstants.DEFAULT_COLUMNS;
import static com.day.cq.wcm.foundation.model.responsivegrid.ResponsiveConstants.SEPARATOR;
public class ResponsiveGridUtils {
static String createClassname(List classNameIngredients) {
return String.join(SEPARATOR, classNameIngredients);
}
/**
* Generates the class names to be applied to the column
*/
static String createClassNames(String initial,
Map breakpoints,
Function> generator) {
if (breakpoints == null) {
breakpoints = Collections.emptyMap();
}
String cssClass = initial;
String cssClasses = breakpoints.values().stream()
.map(generator)
.flatMap(List::stream)
.collect(Collectors.joining(" "));
cssClass += " " + cssClasses;
return cssClass.replaceAll("\\s{2,}", " ").trim();
}
static Map getMissingBreakpoints(Map breakpoints, Set missingBreakpointNames, Map initialDefaultWidths) {
missingBreakpointNames.removeAll(breakpoints.keySet());
Map missingBreakpoints = new HashMap<>();
Breakpoint defaultBreakpoint = breakpoints.get(BREAKPOINT_VARIANT_NAME_DEFAULT);
int width = 0;
int offset = 0;
if (defaultBreakpoint != null) {
width = defaultBreakpoint.getWidth();
offset = defaultBreakpoint.getOffset();
}
for (String missingBreakpoint : missingBreakpointNames) {
int effectiveWidth = width;
if(initialDefaultWidths.containsKey(missingBreakpoint)) {
effectiveWidth = initialDefaultWidths.get(missingBreakpoint);
}
missingBreakpoints.put(missingBreakpoint, new Breakpoint(missingBreakpoint, effectiveWidth, offset, null));
}
return missingBreakpoints;
}
static Map getMissingBreakpoints(Map breakpoints, Map referenceBreakpoints) {
if (referenceBreakpoints == null || referenceBreakpoints.isEmpty()) {
return Collections.emptyMap();
}
Map missingBreakpoints = new HashMap<>();
List missingGridBreakpointNames = new ArrayList<>(referenceBreakpoints.keySet());
missingGridBreakpointNames.removeAll(breakpoints.keySet());
Breakpoint defaultBreakpoint = breakpoints
.get(BREAKPOINT_VARIANT_NAME_DEFAULT);
for (String missingGridBreakpointName : missingGridBreakpointNames) {
int width = 0;
// special value, if there is no offset saved in the database we do not want to render it
int offset = 0;
int gridWidth = 0;
if (defaultBreakpoint != null) {
width = defaultBreakpoint.getWidth();
offset = defaultBreakpoint.getOffset();
}
Breakpoint gridBreakPointVariant = referenceBreakpoints.get(missingGridBreakpointName);
if (gridBreakPointVariant != null) {
gridWidth = gridBreakPointVariant.getWidth();
}
if (width == 0 || width + offset > gridWidth) {
width = gridWidth;
offset = 0;
}
if (width == 0 || width > DEFAULT_COLUMNS) {
width = DEFAULT_COLUMNS;
}
if(offset == 0) {
// workaround to prevent offset to appear
offset = -1;
}
missingBreakpoints.put(missingGridBreakpointName, new Breakpoint(missingGridBreakpointName, width, offset, null));
}
return missingBreakpoints;
}
/**
*
* @param width Width to constrain
* @param offset Offset to constrain
* @param maxWidth Maximum available width
* @return [0] constrained width, [1] constrained offset
*/
static int[] getConstrainedWidthAndOffset(int width, int offset, int maxWidth) {
// width + offset are greater than maximum width allowed
if (width + offset > maxWidth) {
if (width > maxWidth) {
// The width is bigger than the parent
// We take the full size
width = maxWidth;
offset = 0;
} else if (offset < maxWidth) {
// There is room for the offset and a width
width = maxWidth - offset;
} else {
// There is no room for an offset
offset = 0;
}
}
return new int[]{ width, offset };
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy