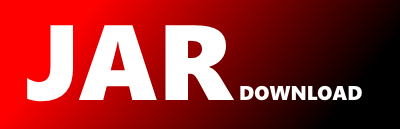
com.day.cq.wcm.msm.api.RolloutManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 1997-2011 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.wcm.msm.api;
import java.util.Arrays;
import javax.jcr.Node;
import javax.jcr.RepositoryException;
import org.apache.sling.api.resource.Resource;
import org.apache.sling.api.resource.ResourceResolver;
import com.day.cq.wcm.api.Page;
import com.day.cq.wcm.api.WCMException;
/**
* Provides a service for managing MSM rollouts.
*/
public interface RolloutManager {
/**
* Trigger type that defines when a rollout should happen.
*/
public static enum Trigger {
/**
* never trigger a rollout (probbly only used for pull scenarios)
*/
NEVER("never"),
/**
* auto rollout on modification
*/
MODIFICATION("modification"),
/**
* auto rollout on activation
*/
PUBLICATION("publish"),
/**
* auto rollout on deactivation
*/
DEACTIVATION("deactivate"),
/**
* rollout on explicit user rollouts
*/
ROLLOUT("rollout");
private final String name;
Trigger(String name) {
this.name = name;
}
public String toString() {
return this.name;
}
public static Trigger fromName(String name) {
for (Trigger m : Trigger.values()) {
if (m.toString().equals(name)
|| m.name().equals(name)) { // fall-back to match name in order to allow (fromName(trigger.name());
return m;
}
}
throw new IllegalArgumentException("Unknown trigger type: " + name);
}
}
/** Parameters for rollouts, with default values */
static public class RolloutParams {
/** main page to rollout */
public Page main; // rollout page parameter
public Page master; // backwards compatibility rollout page parameter
/**
* source resource to rollout if main is null.
* If main value is not null, then main will be used for rollout.
*/
public Resource source;
/** if true, all child pages are updated too */
public boolean isDeep;
/** rollout trigger */
public Trigger trigger;
/** path of lives copies to update, null to update all */
public String [] targets;
/** if not null, (absolute) paths of paragraphs to roll out */
public String [] paragraphs;
/** if not null, (absolute) paths of fields to roll out */
public String[] fields;
/** if true, Live Copy is completely reset */
public boolean reset;
/** deprecated: since 5.8 delete before roll-out */
@Deprecated
public boolean delete;
/** if not null, used to report progress */
public RolloutProgress rolloutProgress;
@Override
public String toString() {
final StringBuilder sb = new StringBuilder();
sb.append(getClass().getSimpleName());
if(main != null) {
sb.append(", Main path=");
sb.append(main.getPath());
} else if(master != null) { // backwards compatibility
sb.append(", Main path=");
sb.append(master.getPath());
} else {
sb.append(", Source path=");
sb.append(source.getPath());
}
if(trigger != null) {
sb.append(", Trigger=");
sb.append(trigger);
}
if(targets != null) {
sb.append(", Targets=");
sb.append(Arrays.asList(targets));
}
if(paragraphs != null) {
sb.append(", Paragraphs=");
sb.append(Arrays.asList(paragraphs));
}
sb.append(isDeep ? " isDeep" : "");
sb.append(reset ? " reset" : "");
return sb.toString();
}
}
/** Used to report ongoing progress of a rollout */
static public interface RolloutProgress {
void reportProgress(String path, String operation);
}
/**
* Execute a rollout on all live copies of the main
page.
* @param params combined rollout parameters
* @throws com.day.cq.wcm.api.WCMException when rollout fails
*/
void rollout(RolloutParams params) throws WCMException;
/**
* Rollout the content for one relation ship.
* @param resolver resource resolver
* @param relation relation to rollout
* @param reset if true
rollout is run in reset mode, Live Copy is completely reset
* @throws WCMException if an error during this operation occurs.
*/
void rollout(ResourceResolver resolver, LiveRelationship relation, boolean reset)
throws WCMException;
/**
* Rollout the content for one relation ship.
* @param resolver resource resolver
* @param relation relation to rollout
* @param reset if true
rollout is run in reset mode, Live Copy is completely reset
* @param autoSave if true
session is saved once the rollout is finished
* @throws WCMException if an error during this operation occurs.
*/
void rollout(ResourceResolver resolver, LiveRelationship relation, boolean reset, boolean autoSave)
throws WCMException;
/**
* Update rollout info on the node
. To use after a rollout operation.
*
* @param node Node to update
* @param deepUpdate Children of the node can be updated by setting
* deepUpdate
to true.
* @param autoSave Save modifications
* @throws WCMException if an error during this operation occurs.
*/
void updateRolloutInfo(Node node, boolean deepUpdate, boolean autoSave) throws WCMException;
/**
* Returns if a property is defined as excluded in the RolloutManager
configuration.
* Excluded properties include reserved properties.
* @param propertyName repository property name.
* @return true if excluded. False otherwise.
* @deprecated Use #isExcludedPageProperty instead.
*/
@SuppressWarnings({"UnusedDeclaration"}) // desired as deprecated
boolean isExcludedProperty(String propertyName);
/**
* Returns if a property is defined as excluded in the RolloutManager
configuration.
* If isPage
is true, checks in page exclusion list. Otherwise, check in paragraph exclusion list
* Excluded properties include reserved properties.
* @param isPage Page property
* @param propertyName repository property name.
* @return true if excluded. False otherwise.
*/
boolean isExcludedProperty(boolean isPage, String propertyName);
/**
* Returns if a property is defined as excluded in the RolloutManager
configuration for a page.
* Excluded properties include reserved properties.
* @param propertyName repository property name.
* @return true if excluded. False otherwise.
*/
boolean isExcludedPageProperty(String propertyName);
/**
* Returns if a property is defined as excluded in the RolloutManager
configuration for a paragraph.
* Excluded properties include reserved properties.
* @param propertyName repository property name.
* @return true if excluded. False otherwise.
*/
boolean isExcludedParagraphProperty(String propertyName);
/**
* Returns true if a {@link javax.jcr.nodetype.NodeType NodeType}
* is configured as excluded in the RolloutManager
configuration
* @param nodeType {@link javax.jcr.nodetype.NodeType#getName() name} of
* the Repository NodeType.
* @return true if excluded.
*/
boolean isExcludedNodeType(String nodeType);
/**
* If true the given {@link javax.jcr.Node Node} will not take part in a
* roll out.
* If one of the {@link javax.jcr.Node Node's} PrimaryNodeType or one of
* its mixin NodeTypes is {@link #isExcludedNodeType(String) excluded},
* this method must return true.
* Implementations can extend rules to exclude Nodes from Inheritance for
* example to exclude
* {@link javax.jcr.nodetype.NodeDefinition#isProtected() protected} Nodes
*
* @param node Node to check.
* @return true if excluded
* @throws RepositoryException if an read/write error during this operation occurs.
* @see #isExcludedNodeType(String)
*/
boolean isExcludedNode(Node node) throws RepositoryException;
/**
* Returns if a property is a MSM reserved property
* @param propertyName repository property name.
* @return true if reserved. False otherwise.
*/
boolean isReservedProperty(String propertyName);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy