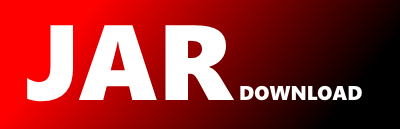
com.day.cq.wcm.webservicesupport.Configuration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2011 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.cq.wcm.webservicesupport;
import java.util.Iterator;
import javax.jcr.Session;
import org.apache.sling.api.resource.Resource;
import aQute.bnd.annotation.ProviderType;
import com.day.cq.commons.inherit.InheritanceValueMap;
import com.day.cq.wcm.api.Template;
/**
* Represents a web service configuration.
*
* @since 5.5
*/
@ProviderType
public interface Configuration {
/**
* Return the jcr:title property of the service.
*
* @return String representation of title
*/
String getTitle();
/**
* Return jcr:description property of the service.
*
* @return String representation of description
*/
String getDescription();
/**
* Returns the name of the configuration. This is the last segment of the
* configuration path.
*
* @return String representation of name
*/
String getName();
/**
* Returns the path of the configuration.
*
* @return String representation of path
*/
String getPath();
/**
* Return the {@link java.util.Date} of last modification of the configuration.
*
* @return UTC milliseconds of the last modification date
*/
Long getLastModified();
/**
* Returns the path to an icon for this service or null
if
* this service does not provide an icon.
*
* @return Path to an icon or null
*/
String getIconPath();
/**
* Returns the path to a thumbnail for this service or null
if
* this service does not provide a thumbnail.
*
* @return Path to an icon or null
*/
String getThumbnailPath();
/**
* Returns the pages {@link Template} or null
if no template
* has been found or the current user does not have read access to the
* template resource specified by the path in the page's template property.
* This is the case on publish instances using default ACL configuration
* (anonymous cannot read templates).
*
* @return Page {@link Template} or null
*/
Template getTemplate();
/**
* Returns the relative parent configuration {@link Resource}.
*
* @return {@link Resource} or null
*/
Resource getParent();
/**
* Returns the configuration {@link Resource}.
*
* @return {@link Resource} or null
*/
Resource getResource();
/**
* Returns the {@link Resource}s content or null
.
*
* @return {@link Resource} or null
*/
Resource getContentResource();
/**
* Returns all properties of this node accessible through the current
* {@link Session}.
*
* @return {@link InheritanceValueMap} with all inherited properties
*/
InheritanceValueMap getProperties();
/**
* Returns a property with provided
* name
of type T or the default value if property was not found.
*
* @param Type of the property
* @param name
* Property name
* @param defaultValue
* Default value
* @return Property value
*/
T get(String name, T defaultValue);
/**
* Returns a property with provided
* name
of type T or the default value if property was not found.
* Takes properties of parent resources / pages into account, if the property is not found locally.
*
* @param Type of the property
* @param name
* Property name
* @param defaultValue
* Default value
* @return Property value
*/
T getInherited(String name, T defaultValue);
/**
* Returns an {@link Iterator} of {@link Configuration} objects loaded from
* the children of the given {@link Resource}
*
* @return {@link Configuration} child {@link Iterator}
*/
Iterator listChildren();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy