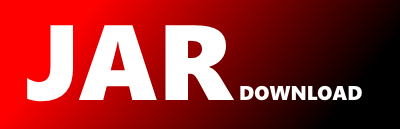
com.day.cq.workflow.WorkflowService Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* Copyright 1997-2008 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.workflow;
import java.util.Dictionary;
import javax.jcr.Session;
import com.day.cq.workflow.exec.Workflow;
import com.day.cq.workflow.model.WorkflowModel;
/**
* The WorkflowService
is the main entry point for accessing the
* workflow engine. It defines life cycle methods for the workflow engine (eg to
* {@link #start()}/{@link #stop()} it).
*
*
* It also provides functionality for getting a
* {@link WorkflowSession}
per user based on the users JCR
* session and according credentials.
*
* Instances of the {@link WorkflowSession}
interface are used
* for all workflow operations like deploying a new {@link WorkflowModel} or
* starting a new {@link Workflow} instance.
*
*/
public interface WorkflowService {
/**
* The start method will initialize and start the
* WorkflowService
properly.
*
* @throws WorkflowException
* Thrown in case that the initialization procedure fails.
*/
void start() throws WorkflowException;
/**
* This methods will shutdown the WorkflowService
properly.
* All active workflows will be suspended and all running processes will be
* terminated.
*/
void stop();
/**
* Creates a new {@link WorkflowSession}
based on the given
* JCR session. The credentials of the JCR session define what the user can
* access and which operations he is allowed to do.
*
* @param session
* The users JCR session to be used for initializing the
* {@link WorkflowSession}
.
* @return A new instance of the {@link WorkflowSession}
* based on the given JCR session.
*/
WorkflowSession getWorkflowSession(Session session);
/**
* Allows users of the WorkflowService
to retrieve settings
* and parameters related to the WorkflowService
* implementation. In common these are provided by the environment (eg. a
* OSGi compliant container or a servlet engine.
*
* @return Returns the map of configuration parameters for the
* WorkflowService
.
*/
Dictionary getConfig();
}