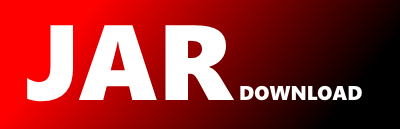
com.day.cq.workflow.event.ExternalProcessPollingEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Copyright 2010 Day Management AG
* Barfuesserplatz 6, 4001 Basel, Switzerland
* All Rights Reserved.
*
* This software is the confidential and proprietary information of
* Day Management AG, ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Day.
*/
package com.day.cq.workflow.event;
import org.apache.sling.event.EventUtil;
import org.osgi.service.event.Event;
import java.io.Serializable;
import java.util.Calendar;
import java.util.Dictionary;
import java.util.Hashtable;
import java.util.Map;
/**
* A helper class to define and create external process polling events, identified using the topic {@link #EVENT_TOPIC}
*/
public class ExternalProcessPollingEvent implements Serializable {
private static final long serialVersionUID = -2349473421221460240L;
/**
* The job topic for adding an entry to the audit log.
*/
public static final String EVENT_TOPIC = "com/day/cq/workflow/external/polling/event";
public static final String PROPERTY_POLLING_TIMEOUT = "com.day.cq.workflow.event.polling.timeout";
public static final String PROPERTY_POLLING_ADVANCE_ON_TIMEOUT = "com.day.cq.workflow.event.polling.advanceOnTimeout";
public static final String PROPERTY_POLLING_START = "com.day.cq.workflow.event.polling.start";
public static final String PROPERTY_POLLING_EVENT = "com.day.cq.workflow.event.polling";
public static final String PROPERTY_POLLING_PROCESS_ID = "com.day.cq.workflow.event.polling.process.id";
private Map workItemMap;
private Serializable externalProcessId;
/**
* Creates a new WorkflowJob.
*
* @param workItemMap {@link Map} item of WorkItem map to be used
*
* @param externalProcessId External process id to be used
*
*/
public ExternalProcessPollingEvent(Map workItemMap, Serializable externalProcessId) {
if (workItemMap == null) {
throw new IllegalArgumentException("work item must not be null.");
}
if (externalProcessId == null) {
throw new IllegalArgumentException("externalProcessId must not be null.");
}
this.workItemMap = workItemMap;
this.externalProcessId = externalProcessId;
}
public Map getWorkItemMap() {
return workItemMap;
}
/**
* Convenience method to create a timed event.
*
* @param period period of time in seconds
*
* @param timeoutSeconds timeout parameter in seconds
*
* @param advanceOnTimeout true or false if it should advance on timeout
*
* @return Event Returns polling {@link Event}
*
*/
public Event createPollingEvent(long period, long timeoutSeconds, boolean advanceOnTimeout) {
final Dictionary props = new Hashtable();
props.put(EventUtil.PROPERTY_TIMED_EVENT_TOPIC, EVENT_TOPIC);
props.put(EventUtil.PROPERTY_TIMED_EVENT_ID, workItemMap.get("id"));
props.put(EventUtil.PROPERTY_TIMED_EVENT_PERIOD, Long.valueOf(period)); //in seconds
//add custom properties
props.put(PROPERTY_POLLING_EVENT, this);
//set timeout
props.put(PROPERTY_POLLING_TIMEOUT, Long.valueOf(timeoutSeconds)); // in seconds
//the start timestamp of the polling event, used to calculate the timeout
props.put(PROPERTY_POLLING_START, Calendar.getInstance().getTime()); // java.util.Date
//the external process id
props.put(PROPERTY_POLLING_PROCESS_ID, externalProcessId);
// whether to advance on timeout or not
props.put(PROPERTY_POLLING_ADVANCE_ON_TIMEOUT, advanceOnTimeout);
return new Event(EventUtil.TOPIC_TIMED_EVENT, props);
}
/**
* Convenience method to create a cancel timed event.
*
* @return Event Returns polling cancel {@link Event}
*
*/
public Event createPollingCancelEvent() {
final Dictionary props = new Hashtable();
props.put(EventUtil.PROPERTY_TIMED_EVENT_TOPIC, EVENT_TOPIC);
props.put(EventUtil.PROPERTY_TIMED_EVENT_ID, workItemMap.get("id"));
return new Event(EventUtil.TOPIC_TIMED_EVENT, props);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy