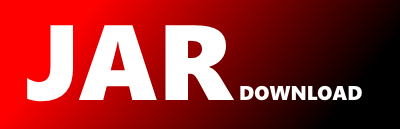
com.day.crx.statistics.StatisticsModule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 1997 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.crx.statistics;
import java.util.Arrays;
import java.util.Iterator;
import java.util.List;
import javax.jcr.RepositoryException;
import javax.jcr.Session;
import com.day.crx.CRXModule;
import com.day.crx.CRXSession;
/**
* StatisticsModule
implements a {@link CRXModule} that writes
* statistical data to a given workspace and runs reports on that data.
*
* @author mreutegg
*/
public class StatisticsModule implements CRXModule {
/**
* The statistics implementation.
*/
private Statistics statistics;
/**
* The name of the statistic workspace. If null
, then the
* default workspace is used.
*/
private String wspName;
//-----------------------------< CRXModule >--------------------------------
/**
* {@inheritDoc}
*/
public String getName() {
return StatisticsModule.class.getName();
}
/**
* {@inheritDoc}
*/
public void start(CRXSession session) throws RepositoryException {
Session s;
if (wspName == null) {
s = session.getSession(session.getWorkspace().getName());
} else {
List wspNames = Arrays.asList(
session.getWorkspace().getAccessibleWorkspaceNames());
if (!wspNames.contains(wspName)) {
// create workspace
session.getWorkspace().createWorkspace(wspName);
}
s = session.getSession(wspName);
}
statistics = new Statistics(s);
}
/**
* {@inheritDoc}
*/
public void stop() {
if (statistics != null) {
statistics.stop();
statistics = null;
}
}
//-------------------------< StatisticsModule >-----------------------------
/**
* Runs a report and returns the result of the report.
*
* @param report the report to run.
* @return the result of the report.
* @throws RepositoryException if an error occurs while reading from the
* workspace.
*/
public Iterator runReport(Report report) throws RepositoryException {
return statistics.runReport(report);
}
/**
* Adds an entry to the statistics workspace.
*
* @param entry the entry to add.
* @throws RepositoryException if an error occurs while writing to the
* workspace.
*/
public void addEntry(Entry entry) throws RepositoryException {
statistics.addEntry(entry);
}
//----------------------------< Properties >--------------------------------
/**
* @return the name of the statistics workspace.
*/
public String getWorkspaceName() {
return wspName;
}
/**
* @param wspName the name of the statistics workspace.
*/
public void setWorkspaceName(String wspName) {
this.wspName = wspName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy