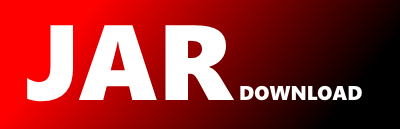
com.day.crx.statistics.loader.Access Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 1997 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.day.crx.statistics.loader;
import java.util.StringTokenizer;
/**
* Access
parses a line of an access.log written by the CQSE.
*
* @author mreutegg
*/
public class Access {
public final String remoteAddr;
public final String username;
public final String dateTime;
public final String timeZone;
public final String requestURI;
public final String status;
public final String contentLength;
public final String referer;
public final String userAgent;
private Access(String remoteAddr,
String username,
String dateTime,
String timeZone,
String requestURI,
String status,
String contentLength,
String referer,
String userAgent) {
this.remoteAddr = remoteAddr;
this.username = username;
this.dateTime = dateTime;
this.timeZone = timeZone;
this.requestURI = requestURI;
this.status = status;
this.contentLength = contentLength;
this.referer = referer;
this.userAgent = userAgent;
}
/**
* @throws IllegalArgumentException on malformed line
*/
public static Access fromString(String line) throws IllegalArgumentException {
StringTokenizer tokenizer = new StringTokenizer(line, " ", false);
try {
String remoteAddr = tokenizer.nextToken();
tokenizer.nextToken(); // dash
String username = tokenizer.nextToken();
String dateTime = tokenizer.nextToken(" [");
String timeZone = tokenizer.nextToken(" ]");
tokenizer.nextToken(" "); // ]
tokenizer.nextToken("\""); // space
tokenizer.nextToken(" \""); // method
String requestURI = tokenizer.nextToken("\"").trim();
// remove http version
requestURI = requestURI.substring(0, requestURI.lastIndexOf(' '));
String status = tokenizer.nextToken("\" ");
String contentLength = tokenizer.nextToken();
tokenizer.nextToken("\""); // space
String referer = tokenizer.nextToken();
tokenizer.nextToken(); // space
String userAgent = tokenizer.nextToken();
return new Access(remoteAddr, username, dateTime, timeZone,
requestURI, status, contentLength, referer, userAgent);
} catch (Exception e) {
throw new IllegalArgumentException();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy