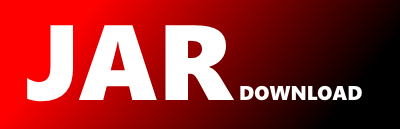
com.day.util.CompoundIterator Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2020 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.day.util;
import java.util.Iterator;
/**
* Implements the Iterator
interface and combines two
* Iterator
objects into one single Iterator
.
*
* The constructor takes two Iterator
arguments: parent
* and child
. Calling {@link #next} on this Iterator
* will first try to return an element from the parent Iterator
* and once the parent Iterator
does not have any more elements
* it will return elements from the child Iterator
.
*
* @version $Revision: 1.2 $, $Date: 2004-08-22 06:56:09 +0200 (Sun, 22 Aug 2004) $
* @author mreutegg
* @since fennec
* Audience wad
*/
public class CompoundIterator implements Iterator {
/**
* The parent Iterator
. Elements from this
* Iterator
are returned first.
*/
private final Iterator parent;
/**
* The child Iterator
. Elements from this
* Iterator
are returned, after the parent
* Iterator
does not have elements any more.
*/
private final Iterator child;
/**
* Creates a CompoundIterator
based on parent
* and child
. This CompountIterator
will first
* return elements from parent
and then elements from
* child
.
* @param parent the Iterator
from where to return the elements
* first.
* @param child the Iterator
from where to return the elements
* after parent
does not have elements any more.
*/
public CompoundIterator(Iterator parent, Iterator child) {
this.parent = parent;
this.child = child;
}
/**
* Returns true
if either parent or child iterator
* has a next element; false
otherwise.
* @return true
if either parent or child iterator
* has a next element; false
otherwise.
*/
public boolean hasNext() {
return parent.hasNext() || child.hasNext();
}
/**
* Returns the next element from the parent or the child
* iterator object.
* @return the next element from the parent or the child
* iterator object.
*/
public Object next() {
return (parent.hasNext()) ? parent.next() : child.next();
}
/**
* Always throws UnsupportedOperationException
* @throws UnsupportedOperationException always!
*/
public void remove() throws UnsupportedOperationException {
throw new UnsupportedOperationException("remove() is not supported in CompoundInterator");
}
}