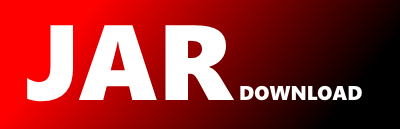
com.day.util.OrderedSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2020 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.day.util;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.Set;
/**
* Helper class that implements a set, but preserves the order of inserting
* the elements when accessing an iterator.
*
* @version $Revision: 1.3 $, $Date: 2004-08-22 02:22:22 +0200 (Sun, 22 Aug 2004) $
* @author tripod
* @since coati, moved to com.day.util for iguana
* Audience core
*/
public class OrderedSet implements Set {
/** the internal map */
private final HashSet set;
/** the list of the order */
private final LinkedList list = new LinkedList();
/**
* Constructs a new, empty set; the backing HashMap instance has
* default initial capacity (16) and load factor (0.75).
*/
public OrderedSet() {
set = new HashSet();
}
/**
* Constructs a new set containing the elements in the specified collection.
* The Set is created with default load factor (0.75) and an
* initial capacity sufficient to contain the elements in the specified
* collection.
*
* @param c the collection whose elements are to be placed into this set.
* @throws NullPointerException if the specified collection is null.
*/
public OrderedSet(Collection c) {
set = new HashSet(java.lang.Math.max((int) (c.size() / .75f) + 1, 16));
addAll(c);
}
/**
* Constructs a new, empty set; the backing Set instance has
* the specified initial capacity and the specified load factor.
*
* @param initialCapacity the initial capacity of the hash map.
* @param loadFactor the load factor of the hash map.
* @throws IllegalArgumentException if the initial capacity is less than
* zero, or if the load factor is nonpositive.
*/
public OrderedSet(int initialCapacity, float loadFactor) {
set = new HashSet(initialCapacity, loadFactor);
}
/**
* Constructs a new, empty set; the backing Set instance has
* the specified initial capacity and default load factor, which is
* 0.75.
*
* @param initialCapacity the initial capacity of the hash table.
* @throws IllegalArgumentException if the initial capacity is less than
* zero.
*/
public OrderedSet(int initialCapacity) {
set = new HashSet(initialCapacity);
}
public int size() {
return set.size();
}
public boolean isEmpty() {
return set.isEmpty();
}
public boolean contains(Object o) {
return set.contains(o);
}
public Iterator iterator() {
return list.iterator();
}
public Object[] toArray() {
return list.toArray();
}
public Object[] toArray(Object a[]) {
return list.toArray(a);
}
public boolean add(Object o) {
if (set.add(o)) {
list.add(o);
return true;
} else {
return false;
}
}
public boolean remove(Object o) {
if (set.remove(o)) {
list.remove(o);
return true;
} else {
return false;
}
}
public boolean containsAll(Collection c) {
return set.containsAll(c);
}
public boolean addAll(Collection c) {
boolean ret=false;
Iterator iter = c.iterator();
while (iter.hasNext()) {
ret |= add(iter.next());
}
return ret;
}
public boolean retainAll(Collection c) {
// not quite correct :-)
set.clear();
list.clear();
return addAll(c);
}
public boolean removeAll(Collection c) {
boolean ret=false;
Iterator iter = c.iterator();
while (iter.hasNext()) {
ret |= remove(iter.next());
}
return ret;
}
public void clear() {
set.clear();
list.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy