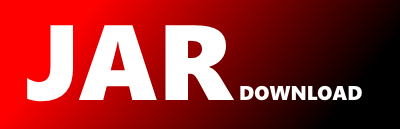
com.day.util.diff.DiffWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2017 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*/
package com.day.util.diff;
import java.io.Writer;
import java.io.IOException;
import java.io.PrintWriter;
/**
* Implements a writer that provides an additional method {@link #writeNewLine()}
* that can be used for writing line separators which can be defined. A
* {@link PrintWriter} would actually be better, but it does not support
* defining the line separator to use.
*/
public class DiffWriter extends Writer {
/**
* native line separator
*/
public static final String LS_NATIVE = System.getProperty("line.separator");
/**
* unix line separator
*/
public static final String LS_UNIX = "\n";
/**
* windows line separator
*/
public static final String LS_WINDOWS = "\r\n";
/**
* the wrapped writer
*/
private final Writer out;
/**
* the line seperator to use for {@link #writeNewLine()}
*/
private String lineSeparator = LS_NATIVE;
/**
* {@inheritDoc}
*/
public DiffWriter(Writer out) {
this.out = out;
}
/**
* {@inheritDoc}
*
* @param lineSeparator the line seperator to use for {@link #writeNewLine()}
*/
public DiffWriter(Writer out, String lineSeparator) {
this.out = out;
this.lineSeparator = lineSeparator;
}
/**
* Writes a new line according to the defined line separator
* @throws IOException if an I/O error occurs
*/
public void writeNewLine() throws IOException {
write(lineSeparator);
}
/**
* {@inheritDoc}
*/
public void write(int c) throws IOException {
out.write(c);
}
/**
* {@inheritDoc}
*/
public void write(char[] cbuf) throws IOException {
out.write(cbuf);
}
/**
* {@inheritDoc}
*/
public void write(char[] cbuf, int off, int len) throws IOException {
out.write(cbuf, off, len);
}
/**
* {@inheritDoc}
*/
public void write(String str) throws IOException {
out.write(str);
}
/**
* {@inheritDoc}
*/
public void write(String str, int off, int len) throws IOException {
out.write(str, off, len);
}
/**
* {@inheritDoc}
*/
public void flush() throws IOException {
out.flush();
}
/**
* {@inheritDoc}
*/
public void close() throws IOException {
out.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy