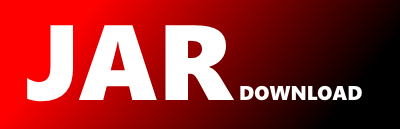
com.github.jknack.handlebars.Options Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/**
* Copyright (c) 2012-2015 Edgar Espina
*
* This file is part of Handlebars.java.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.jknack.handlebars;
import java.io.IOException;
import java.io.Writer;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
/**
* Options available for {@link Helper#apply(Object, Options)}.
* Usage:
*
*
* Options options = new Options.Builder(handlebars, context, fn)
* .build();
*
*
* Optionally you can set parameters and hash table:
*
*
* Options options = new Options.Builder(handlebars, context, fn)
* .setParams(new Object[] {})
* .setHash(hash)
* .build();
*
*
* @author edgar.espina
* @since 0.1.0
*
* @deprecated com.github.jknack.handlebars package is deprecated and marked for removal in subsequent releases which will involve removal of the handlebars dependency in AEM.
*/
@Deprecated(since = "2024-07-10")
public class Options {
/**
* Buffer like use it to increase rendering time while using helpers.
*
* @author edgar
* @since 2.3.2
*
* @deprecated com.github.jknack.handlebars package is deprecated and marked for removal in subsequent releases which will involve removal of the handlebars dependency in AEM.
*/
@Deprecated(since = "2024-07-10")
public interface Buffer extends Appendable, CharSequence {
}
/**
* This buffer will write into the underlying writer. It won't be any visible output and
* {@link #toString()} returns an empty string.
*
* @author edgar
* @since 2.3.2
*
* @deprecated com.github.jknack.handlebars package is deprecated and marked for removal in subsequent releases which will involve removal of the handlebars dependency in AEM.
*/
@Deprecated(since = "2024-07-10")
public static class NativeBuffer implements Buffer {
/**
* Writer.
*/
private Writer writer;
/**
* Creates a new {@link NativeBuffer}.
*
* @param writer A writer. Required.
*/
public NativeBuffer(final Writer writer) {
this.writer = writer;
}
@Override
public Appendable append(final CharSequence csq) throws IOException {
writer.append(csq);
return this;
}
@Override
public Appendable append(final CharSequence csq, final int start, final int end) throws IOException {
writer.append(csq, start, end);
return this;
}
@Override
public Appendable append(final char c) throws IOException {
writer.append(c);
return this;
}
@Override
public int length() {
// no need to merge anything
return 0;
}
@Override
public char charAt(final int index) {
throw new UnsupportedOperationException();
}
@Override
public CharSequence subSequence(final int start, final int end) {
throw new UnsupportedOperationException();
}
@Override
public String toString() {
// no need to merge anything
return "";
}
}
/**
* A {@link StringBuilder} implementation.
*
* @author edgar
* @since 2.3.2
*
* @deprecated com.github.jknack.handlebars package is deprecated and marked for removal in subsequent releases which will involve removal of the handlebars dependency in AEM.
*/
@Deprecated(since = "2024-07-10")
public static class InMemoryBuffer implements Buffer {
/**
* A buffer.
*/
private StringBuilder buffer = new StringBuilder();
@Override
public Appendable append(final CharSequence csq) throws IOException {
buffer.append(csq);
return this;
}
@Override
public Appendable append(final CharSequence csq, final int start, final int end) throws IOException {
buffer.append(csq, start, end);
return this;
}
@Override
public Appendable append(final char c) throws IOException {
buffer.append(c);
return this;
}
@Override
public int length() {
return buffer.length();
}
@Override
public char charAt(final int index) {
return buffer.charAt(index);
}
@Override
public CharSequence subSequence(final int start, final int end) {
return buffer.subSequence(start, end);
}
}
/**
* An {@link Options} builder.
*
* @author edgar.espina
* @since 0.9.0
*
* @deprecated com.github.jknack.handlebars package is deprecated and marked for removal in subsequent releases which will involve removal of the handlebars dependency in AEM.
*/
@Deprecated(since = "2024-07-10")
public static class Builder {
/**
* The {@link Handlebars} object. Not null.
*/
private Handlebars handlebars;
/**
* The current context. Not null.
*/
private Context context;
/**
* The current template. Not null.
*/
private Template fn;
/**
* The current inverse template. Not null.
*/
private Template inverse = Template.EMPTY;
/**
* Empty params.
*/
private static Object[] EMPTY_PARAMS = {};
/**
* The parameters. Not null.
*/
private Object[] params = EMPTY_PARAMS;
/**
* The hash options. Not null.
*/
private Map hash = Collections.emptyMap();
/**
* The {@link TagType} from where the helper was called.
*/
private TagType tagType;
/**
* The name of the helper.
*/
private String helperName;
/**
* Output writer.
*/
private Writer writer;
/**
* Block params.
*/
private List blockParams = Collections.emptyList();
/**
* Creates a new {@link Builder}.
*
* @param handlebars A handlebars object. Required.
* @param helperName The name of the helper. Required.
* @param tagType The {@link TagType} from where the helper was called.
* @param context A context object. Required.
* @param fn A template object. Required.
*/
public Builder(final Handlebars handlebars, final String helperName, final TagType tagType, final Context context, final Template fn) {
this.handlebars = handlebars;
this.helperName = helperName;
this.tagType = tagType;
this.context = context;
this.fn = fn;
}
/**
* Build a new {@link Options} object.
*
* @return A new {@link Options} object.
*/
public Options build() {
Options options = new Options(handlebars, helperName, tagType, context, fn, inverse, params, hash, blockParams);
options.writer = writer;
// clear out references
handlebars = null;
tagType = null;
context = null;
fn = null;
inverse = null;
params = null;
hash = null;
writer = null;
return options;
}
/**
* Set the options hash.
*
* @param hash A hash table. Required.
* @return This builder.
*/
public Builder setHash(final Map hash) {
this.hash = hash;
return this;
}
/**
* Set the options block params.
*
* @param blockParams A block params. Required.
* @return This builder.
*/
public Builder setBlockParams(final List blockParams) {
this.blockParams = blockParams;
return this;
}
/**
* Set the inverse template.
*
* @param inverse Inverse template. Required.
* @return This builder.
*/
public Builder setInverse(final Template inverse) {
this.inverse = inverse;
return this;
}
/**
* Set the options parameters.
*
* @param params A parameters list. Required.
* @return This builder.
*/
public Builder setParams(final Object[] params) {
this.params = params;
return this;
}
/**
* Set a writer, useful to improve performance.
*
* @param writer A writer. Required.
* @return This builder.
*/
public Builder setWriter(final Writer writer) {
this.writer = writer;
return this;
}
}
/**
* The {@link Handlebars} object. Not null.
*/
public final Handlebars handlebars;
/**
* The current context. Not null.
*/
public final Context context;
/**
* The current template. Not null.
*/
public final Template fn;
/**
* The current inverse template. Not null.
*/
public final Template inverse;
/**
* The parameters. Not null.
*/
public final Object[] params;
/**
* The hash options. Not null.
*/
public final Map hash;
/**
* The {@link TagType} from where the helper was called.
*/
public final TagType tagType;
/**
* The name of the helper.
*/
public final String helperName;
/**
* Output writer.
*/
private Writer writer;
/**
* Block param names.
*/
public final List blockParams;
/**
* True, if there is any block param.
*/
private boolean hasBlockParams;
/**
* Creates a new Handlebars {@link Options}.
*
* @param handlebars The handlebars instance. Required.
* @param helperName The name of the helper. Required.
* @param tagType The {@link TagType} from where the helper was called.
* @param context The current context. Required.
* @param fn The template function. Required.
* @param inverse The inverse template function. Required.
* @param params The parameters. Required.
* @param hash The optional hash. Required.
* @param blockParams The block param names. Required.
*/
public Options(final Handlebars handlebars, final String helperName, final TagType tagType, final Context context, final Template fn, final Template inverse, final Object[] params, final Map hash, final List blockParams) {
this.handlebars = handlebars;
this.helperName = helperName;
this.tagType = tagType;
this.context = context;
this.fn = fn;
this.inverse = inverse;
this.params = params;
this.hash = hash;
this.blockParams = blockParams;
hasBlockParams = this.blockParams.size() > 0;
}
/**
* Creates a new Handlebars {@link Options}.
*
* @param handlebars The handlebars instance. Required.
* @param helperName The name of the helper. Required.
* @param tagType The {@link TagType} from where the helper was called.
* @param context The current context. Required.
* @param fn The template function. Required.
* @param inverse The inverse template function. Required.
* @param params The parameters. Required.
* @param hash The optional hash. Required.
* @param blockParams The block param names. Required.
* @param writer A writer. Optional.
*/
public Options(final Handlebars handlebars, final String helperName, final TagType tagType, final Context context, final Template fn, final Template inverse, final Object[] params, final Map hash, final List blockParams, final Writer writer) {
this.handlebars = handlebars;
this.helperName = helperName;
this.tagType = tagType;
this.context = context;
this.fn = fn;
this.inverse = inverse;
this.params = params;
this.hash = hash;
this.blockParams = blockParams;
this.writer = writer;
hasBlockParams = this.blockParams.size() > 0;
}
/**
* Apply the {@link #fn} template using the default context.
*
* @return The resulting text.
* @throws IOException If a resource cannot be loaded.
*/
public CharSequence fn() throws IOException {
return apply(fn, context, blockParams(context.model));
}
/**
* Apply the {@link #fn} template using the provided context.
*
* @param context The context to use.
* @return The resulting text.
* @throws IOException If a resource cannot be loaded.
*/
public CharSequence fn(final Object context) throws IOException {
Context ctx = wrap(context);
return apply(fn, ctx, blockParams(ctx.model));
}
/**
* Apply the {@link #fn} template using the provided context.
*
* @param context The context to use.
* @return The resulting text.
* @throws IOException If a resource cannot be loaded.
*/
public CharSequence fn(final Context context) throws IOException {
Context ctx = wrap(context);
return apply(fn, ctx, blockParams(ctx.model));
}
/**
* Apply the {@link #inverse} template using the default context.
*
* @return The resulting text.
* @throws IOException If a resource cannot be loaded.
*/
public CharSequence inverse() throws IOException {
return apply(inverse, context, blockParams(context.model));
}
/**
* Apply the {@link #inverse} template using the provided context.
*
* @param context The context to use.
* @return The resulting text.
* @throws IOException If a resource cannot be loaded.
*/
public CharSequence inverse(final Object context) throws IOException {
Context ctx = wrap(context);
return apply(inverse, ctx, blockParams(ctx.model));
}
/**
* Apply the {@link #inverse} template using the provided context.
*
* @param context The context to use.
* @return The resulting text.
* @throws IOException If a resource cannot be loaded.
*/
public CharSequence inverse(final Context context) throws IOException {
Context ctx = wrap(context);
return apply(inverse, ctx, blockParams(ctx.model));
}
/**
* Apply the given template to the provided context. The context stack is
* propagated allowing the access to the whole stack.
*
* @param template The template.
* @param context The context object.
* @return The resulting text.
* @throws IOException If a resource cannot be loaded.
*/
public CharSequence apply(final Template template, final Object context) throws IOException {
Context ctx = wrap(context);
return apply(template, ctx, blockParams(ctx.model));
}
/**
* Apply the given template to the provided context. The context stack is
* propagated allowing the access to the whole stack.
*
* @param template The template.
* @param context The context object.
* @return The resulting text.
* @throws IOException If a resource cannot be loaded.
*/
public CharSequence apply(final Template template, final Context context) throws IOException {
Context ctx = wrap(context);
return apply(template, ctx, blockParams(ctx.model));
}
/**
* Apply the given template to the provided context. The context stack is
* propagated allowing the access to the whole stack.
*
* @param template The template.
* @param context The context object.
* @param blockParams The block param values.
* @return The resulting text.
* @throws IOException If a resource cannot be loaded.
*/
public CharSequence apply(final Template template, final Context context, final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy