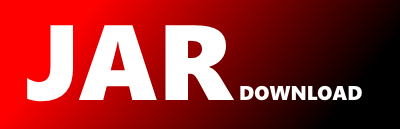
com.scene7.ipsapi.VideoEncoding Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.0
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.06.06 at 01:39:50 PM UTC
//
package com.scene7.ipsapi;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for VideoEncoding complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="VideoEncoding">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="fileSuffix" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="playbackTarget" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="width" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="height" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="videoCodec" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="videoBitrate" type="{http://www.w3.org/2001/XMLSchema}int"/>
* <element name="minBitrate" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="maxBitrate" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="constantBitrate" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="frameRate" type="{http://www.w3.org/2001/XMLSchema}double" minOccurs="0"/>
* <element name="keyframeInterval" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="h264Profile" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="h264Level" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="twoPass" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="audioCodec" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="audioBitrate" type="{http://www.w3.org/2001/XMLSchema}int"/>
* <element name="audioSampleRate" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="advancedSettings" type="{http://www.scene7.com/IpsApi/xsd/2024-05-30-beta}PropertyArray" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "VideoEncoding", namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta", propOrder = {
"name",
"fileSuffix",
"playbackTarget",
"width",
"height",
"videoCodec",
"videoBitrate",
"minBitrate",
"maxBitrate",
"constantBitrate",
"frameRate",
"keyframeInterval",
"h264Profile",
"h264Level",
"twoPass",
"audioCodec",
"audioBitrate",
"audioSampleRate",
"advancedSettings"
})
public class VideoEncoding {
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta", required = true)
protected String name;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta", required = true)
protected String fileSuffix;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected String playbackTarget;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected Integer width;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected Integer height;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected String videoCodec;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected int videoBitrate;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected Integer minBitrate;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected Integer maxBitrate;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected Boolean constantBitrate;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected Double frameRate;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected Integer keyframeInterval;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected String h264Profile;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected String h264Level;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected Boolean twoPass;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected String audioCodec;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected int audioBitrate;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected Integer audioSampleRate;
@XmlElement(namespace = "http://www.scene7.com/IpsApi/xsd/2024-05-30-beta")
protected PropertyArray advancedSettings;
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the fileSuffix property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFileSuffix() {
return fileSuffix;
}
/**
* Sets the value of the fileSuffix property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFileSuffix(String value) {
this.fileSuffix = value;
}
/**
* Gets the value of the playbackTarget property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPlaybackTarget() {
return playbackTarget;
}
/**
* Sets the value of the playbackTarget property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPlaybackTarget(String value) {
this.playbackTarget = value;
}
/**
* Gets the value of the width property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getWidth() {
return width;
}
/**
* Sets the value of the width property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setWidth(Integer value) {
this.width = value;
}
/**
* Gets the value of the height property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getHeight() {
return height;
}
/**
* Sets the value of the height property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setHeight(Integer value) {
this.height = value;
}
/**
* Gets the value of the videoCodec property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVideoCodec() {
return videoCodec;
}
/**
* Sets the value of the videoCodec property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVideoCodec(String value) {
this.videoCodec = value;
}
/**
* Gets the value of the videoBitrate property.
*
*/
public int getVideoBitrate() {
return videoBitrate;
}
/**
* Sets the value of the videoBitrate property.
*
*/
public void setVideoBitrate(int value) {
this.videoBitrate = value;
}
/**
* Gets the value of the minBitrate property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getMinBitrate() {
return minBitrate;
}
/**
* Sets the value of the minBitrate property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setMinBitrate(Integer value) {
this.minBitrate = value;
}
/**
* Gets the value of the maxBitrate property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getMaxBitrate() {
return maxBitrate;
}
/**
* Sets the value of the maxBitrate property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setMaxBitrate(Integer value) {
this.maxBitrate = value;
}
/**
* Gets the value of the constantBitrate property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isConstantBitrate() {
return constantBitrate;
}
/**
* Sets the value of the constantBitrate property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setConstantBitrate(Boolean value) {
this.constantBitrate = value;
}
/**
* Gets the value of the frameRate property.
*
* @return
* possible object is
* {@link Double }
*
*/
public Double getFrameRate() {
return frameRate;
}
/**
* Sets the value of the frameRate property.
*
* @param value
* allowed object is
* {@link Double }
*
*/
public void setFrameRate(Double value) {
this.frameRate = value;
}
/**
* Gets the value of the keyframeInterval property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getKeyframeInterval() {
return keyframeInterval;
}
/**
* Sets the value of the keyframeInterval property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setKeyframeInterval(Integer value) {
this.keyframeInterval = value;
}
/**
* Gets the value of the h264Profile property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getH264Profile() {
return h264Profile;
}
/**
* Sets the value of the h264Profile property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setH264Profile(String value) {
this.h264Profile = value;
}
/**
* Gets the value of the h264Level property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getH264Level() {
return h264Level;
}
/**
* Sets the value of the h264Level property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setH264Level(String value) {
this.h264Level = value;
}
/**
* Gets the value of the twoPass property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isTwoPass() {
return twoPass;
}
/**
* Sets the value of the twoPass property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setTwoPass(Boolean value) {
this.twoPass = value;
}
/**
* Gets the value of the audioCodec property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAudioCodec() {
return audioCodec;
}
/**
* Sets the value of the audioCodec property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAudioCodec(String value) {
this.audioCodec = value;
}
/**
* Gets the value of the audioBitrate property.
*
*/
public int getAudioBitrate() {
return audioBitrate;
}
/**
* Sets the value of the audioBitrate property.
*
*/
public void setAudioBitrate(int value) {
this.audioBitrate = value;
}
/**
* Gets the value of the audioSampleRate property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getAudioSampleRate() {
return audioSampleRate;
}
/**
* Sets the value of the audioSampleRate property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setAudioSampleRate(Integer value) {
this.audioSampleRate = value;
}
/**
* Gets the value of the advancedSettings property.
*
* @return
* possible object is
* {@link PropertyArray }
*
*/
public PropertyArray getAdvancedSettings() {
return advancedSettings;
}
/**
* Sets the value of the advancedSettings property.
*
* @param value
* allowed object is
* {@link PropertyArray }
*
*/
public void setAdvancedSettings(PropertyArray value) {
this.advancedSettings = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy