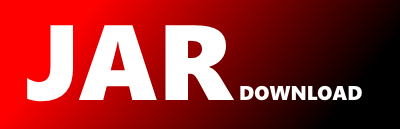
javax.jcr.security.AccessControlList Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* Copyright 2008 Day Management AG, Switzerland. All rights reserved.
*/
package javax.jcr.security;
import javax.jcr.RepositoryException;
import java.security.Principal;
/**
* The AccessControlList
is an AccessControlPolicy
* representing a list of {@link AccessControlEntry access control entries}. It
* is mutable before being {@link AccessControlManager#setPolicy(String,
* AccessControlPolicy) set} to the AccessControlManager and consequently
* defines methods to read and mutate the list i.e. to get, add or remove
* individual entries.
*
* @since JCR 2.0
*/
public interface AccessControlList extends AccessControlPolicy {
/**
* Returns all access control entries present with this policy.
*
* This method is only guaranteed to return an AccessControlEntry
* if that AccessControlEntry
has been assigned through this
* API.
*
* @return all access control entries present with this policy.
* @throws RepositoryException if an error occurs.
*/
public AccessControlEntry[] getAccessControlEntries() throws RepositoryException;
/**
* Adds an access control entry to this policy consisting of the specified
* principal
and the specified privileges
.
*
* This method returns true
if this policy was modified,
* false
otherwise.
*
* How the entries are grouped within the list is an implementation detail.
* An implementation may e.g. combine the specified privileges with those
* added by a previous call to addAccessControlEntry
for the
* same Principal
. However, a call to addAccessControlEntry
* for a given Principal
can never remove a
* Privilege
added by a previous call.
*
* The modification does not take effect until this policy has been set to a
* node by calling {@link AccessControlManager#setPolicy(String,
* AccessControlPolicy)} and save
is performed.
*
* @param principal a Principal
.
* @param privileges an array of Privilege
s.
* @return true
if this policy was modify; false
* otherwise.
* @throws AccessControlException if the specified principal or any of the
* privileges does not exist or if some other access control related
* exception occurs.
* @throws RepositoryException if another error occurs.
*/
public boolean addAccessControlEntry(
Principal principal,
Privilege[] privileges)
throws AccessControlException, RepositoryException;
/**
* Removes the specified AccessControlEntry
from this policy.
*
* Only exactly those entries obtained through getAccessControlEntries
* can be removed. This method does not take effect until this policy has
* been re-set to a node by calling {@link AccessControlManager#setPolicy(String,
* AccessControlPolicy)} and save
is performed.
*
* @param ace the access control entry to be removed.
* @throws AccessControlException if the specified entry is not present on
* the specified node.
* @throws RepositoryException if another error occurs.
*/
public void removeAccessControlEntry(AccessControlEntry ace)
throws AccessControlException, RepositoryException;
}