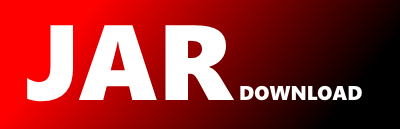
javax.jcr.security.AccessControlManager Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* Copyright 2008 Day Management AG, Switzerland. All rights reserved.
*/
package javax.jcr.security;
import javax.jcr.AccessDeniedException;
import javax.jcr.PathNotFoundException;
import javax.jcr.RepositoryException;
import javax.jcr.lock.LockException;
import javax.jcr.version.VersionException;
/**
* The AccessControlManager
object is accessed via {@link
* javax.jcr.Session#getAccessControlManager()}. It provides methods for:
* - Access control discovery
- Assigning access control policies
*
*
* @since JCR 2.0
*/
public interface AccessControlManager {
/**
* Returns the privileges supported for absolute path absPath
,
* which must be an existing node.
*
* This method does not return the privileges held by the session. Instead,
* it returns the privileges that the repository supports.
*
* @param absPath an absolute path.
* @return an array of Privilege
s.
* @throws PathNotFoundException if no node at absPath
exists
* or the session does not have sufficient access to retrieve a node at that
* location.
* @throws RepositoryException if another error occurs.
*/
public Privilege[] getSupportedPrivileges(String absPath)
throws PathNotFoundException, RepositoryException;
/**
* Returns the privilege with the specified privilegeName
.
* Since the privilege name is a JCR name, it may be passed in either
* qualified or expanded form (see specification for details on JCR names).
*
* @param privilegeName the name of an existing privilege.
* @return the Privilege
with the specified
* privilegeName
.
* @throws AccessControlException if no privilege with the specified name
* exists.
* @throws RepositoryException if another error occurs.
*/
public Privilege privilegeFromName(String privilegeName)
throws AccessControlException, RepositoryException;
/**
* Returns whether the session has the specified privileges for absolute
* path absPath
, which must be an existing node.
*
* Testing an aggregate privilege is equivalent to testing each non
* aggregate privilege among the set returned by calling
* Privilege.getAggregatePrivileges()
for that privilege.
*
* The results reported by the this method reflect the net effect of
* the currently applied control mechanisms. It does not reflect unsaved
* access control policies or unsaved access control entries. Changes to
* access control status caused by these mechanisms only take effect on
* Session.save()
and are only then reflected in the results of
* the privilege test methods.
*
* @param absPath an absolute path.
* @param privileges an array of Privilege
s.
* @return true
if the session has the specified privileges;
* false
otherwise.
* @throws PathNotFoundException if no node at absPath
exists
* or the session does not have sufficent access to retrieve a node at that
* location.
* @throws RepositoryException if another error occurs.
*/
public boolean hasPrivileges(String absPath, Privilege[] privileges)
throws PathNotFoundException, RepositoryException;
/**
* Returns the privileges the session has for absolute path absPath, which
* must be an existing node.
*
* The returned privileges are those for which {@link #hasPrivileges} would
* return true
.
*
* The results reported by the this method reflect the net effect of
* the currently applied control mechanisms. It does not reflect unsaved
* access control policies or unsaved access control entries. Changes to
* access control status caused by these mechanisms only take effect on
* Session.save()
and are only then reflected in the results of
* the privilege test methods.
*
* @param absPath an absolute path.
* @return an array of Privilege
s.
* @throws PathNotFoundException if no node at absPath
exists
* or the session does not have sufficient access to retrieve a node at that
* location.
* @throws RepositoryException if another error occurs.
*/
public Privilege[] getPrivileges(String absPath)
throws PathNotFoundException, RepositoryException;
/**
* Returns the AccessControlPolicy
objects that have been set
* to the node at absPath
or an empty array if no policy has
* been set. This method reflects the binding state, including transient
* policy modifications.
*
* Use {@link #getEffectivePolicies(String)} in order to determine the
* policy that effectively applies at absPath
.
*
* @param absPath an absolute path.
* @return an array of AccessControlPolicy
objects or an empty
* array if no policy has been set.
* @throws PathNotFoundException if no node at absPath
exists
* or the session does not have sufficient access to retrieve a node at that
* location.
* @throws AccessDeniedException if the session lacks
* READ_ACCESS_CONTROL
privilege for the absPath
* node.
* @throws RepositoryException if another error occurs.
*/
public AccessControlPolicy[] getPolicies(String absPath)
throws PathNotFoundException, AccessDeniedException, RepositoryException;
/**
* Returns the AccessControlPolicy
objects that currently are
* in effect at the node at absPath
. This may be policies set
* through this API or some implementation specific (default) policies.
*
* @param absPath an absolute path.
* @return an array of AccessControlPolicy
objects.
* @throws PathNotFoundException if no node at absPath
exists
* or the session does not have sufficient access to retrieve a node at that
* location.
* @throws AccessDeniedException if the session lacks
* READ_ACCESS_CONTROL
privilege for the absPath
* node.
* @throws RepositoryException if another error occurs.
*/
public AccessControlPolicy[] getEffectivePolicies(String absPath)
throws PathNotFoundException, AccessDeniedException, RepositoryException;
/**
* Returns the access control policies that are capable of being applied to
* the node at absPath
.
*
* @param absPath an absolute path.
* @return an AccessControlPolicyIterator
over the applicable
* access control policies or an empty iterator if no policies are
* applicable.
* @throws PathNotFoundException if no node at absPath
exists
* or the session does not have sufficient access to retrieve a node at that
* location.
* @throws AccessDeniedException if the session lacks
* READ_ACCESS_CONTROL
privilege for the absPath
* node.
* @throws RepositoryException if another error occurs.
*/
public AccessControlPolicyIterator getApplicablePolicies(String absPath)
throws PathNotFoundException, AccessDeniedException, RepositoryException;
/**
* Binds the policy
to the node at absPath
.
*
* The behavior of the call acm.setPolicy(absPath, policy)
* differs depending on how the policy
object was originally
* acquired.
*
* If policy
was acquired through {@link #getApplicablePolicies
* acm.getApplicablePolicies(absPath)} then that policy
object
* is added to the node at absPath
.
*
* On the other hand, if policy
was acquired through {@link
* #getPolicies acm.getPolicies(absPath)} then that policy
* object (usually after being altered) replaces its former version on the
* node at absPath
.
*
* This is session-write method and therefore the access control policy is
* only dispatched on save
and will only take effect upon
* persist.
*
* A VersionException
will be thrown either immediately, on
* dispatch or on persists, if the node at absPath
is read-only
* due to a checked-in node. Implementations may differ on when this
* validation is performed.
*
* A LockException
will be thrown either immediately, on
* dispatch or on persists, if a lock prevents the operation.
* Implementations may differ on when this validation is performed.
*
* @param absPath an absolute path.
* @param policy the AccessControlPolicy
to be applied.
* @throws PathNotFoundException if no node at absPath
exists
* or the session does not have sufficient access to retrieve a node at that
* location.
* @throws AccessControlException if the policy is not applicable.
* @throws AccessDeniedException if the session lacks
* MODIFY_ACCESS_CONTROL
privilege for the absPath
* node.
* @throws LockException if a lock applies at the node at
* absPath
and this implementation performs this validation
* immediately.
* @throws VersionException if the node at absPath
is read-only
* due to a checked-in node and this implementation performs this validation
* immediately.
* @throws RepositoryException if another error occurs.
*/
public void setPolicy(String absPath, AccessControlPolicy policy)
throws PathNotFoundException, AccessControlException,
AccessDeniedException, LockException, VersionException, RepositoryException;
/**
* Removes the specified AccessControlPolicy
from the node at
* absPath
.
*
* An AccessControlPolicy
can only be removed if it was bound
* to the specified node through this API before. The effect of the removal
* only takes place upon Session.save()
. Note, that an
* implementation default or any other effective AccessControlPolicy
* that has not been applied to the node before may never be removed using
* this method.
*
* A PathNotFoundException
is thrown if no node at
* absPath
exists or the session does not have privilege to
* retrieve the node.
*
* An AccessControlException
is thrown if the policy to remove
* does not exist at the node at absPath
.
*
* An AccessDeniedException
is thrown if the session lacks
* MODIFY_ACCESS_CONTROL
privilege for the absPath
* node.
*
* An LockException
is thrown either immediately, on dispatch
* or on persists, if the node at absPath
is locked.
* Implementations may differ on when this validation is performed.
*
* An VersionException
is thrown either immediately, on
* dispatch or on persists, if the node at absPath
is read-only
* due to a checked-in node.Implementations may differ on when this
* validation is performed.
*
* A RepositoryException
is thrown if another error occurs.
*
* @param absPath an absolute path.
* @param policy the policy to be removed.
* @throws PathNotFoundException if no node at absPath
exists
* or the session does not have sufficient access to retrieve a node at that
* location.
* @throws AccessControlException if no policy exists.
* @throws AccessDeniedException if the session lacks
* MODIFY_ACCESS_CONTROL
privilege for the absPath
* node.
* @throws LockException if a lock applies at the node at
* absPath
and this implementation performs this validation
* immediately instead of waiting until save
.
* @throws VersionException if the node at absPath
is
* versionable and checked-in or is non-versionable but its nearest
* versionable ancestor is checked-in and this implementation performs this
* validation immediately instead of waiting until save
.
* @throws RepositoryException if another error occurs.
*/
public void removePolicy(String absPath, AccessControlPolicy policy)
throws PathNotFoundException, AccessControlException,
AccessDeniedException, LockException, VersionException, RepositoryException;
}