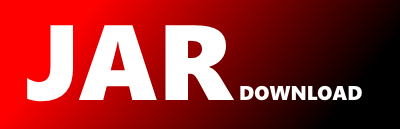
org.apache.jackrabbit.oak.commons.io.BurnOnCloseFileIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.jackrabbit.oak.commons.io;
import static org.apache.commons.io.FileUtils.forceDelete;
import static org.apache.jackrabbit.oak.commons.IOUtils.closeQuietly;
import java.io.BufferedWriter;
import java.io.Closeable;
import java.io.File;
import java.io.IOException;
import java.util.Iterator;
import java.util.function.Function;
import org.apache.commons.io.LineIterator;
import org.apache.jackrabbit.guava.common.collect.AbstractIterator;
import org.apache.jackrabbit.oak.commons.FileIOUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Implements a {@link java.io.Closeable} wrapper over a {@link LineIterator}.
* Also has a transformer to transform the output. If the underlying file is
* provided then it deletes the file on {@link #close()}.
*
* If there is a scope for lines in the file containing line break characters it
* should be ensured that the files is written with
* {@link FileIOUtils#writeAsLine(BufferedWriter, String, boolean)} with true to escape
* line break characters and should be properly unescaped on read. A custom
* transformer can also be provided to unescape.
*
* @param
* the type of elements in the iterator
*/
public class BurnOnCloseFileIterator implements Closeable, Iterator {
private static final Logger LOG = LoggerFactory.getLogger(BurnOnCloseFileIterator.class);
private final Impl delegate;
public BurnOnCloseFileIterator(Iterator iterator, Function transformer) {
this.delegate = new Impl(iterator, null, transformer);
}
public BurnOnCloseFileIterator(Iterator iterator, File backingFile, Function transformer) {
this.delegate = new Impl(iterator, backingFile, transformer);
}
@Override
public boolean hasNext() {
return this.delegate.hasNext();
}
@Override
public T next() {
return this.delegate.next();
}
@Override
public void close() throws IOException {
this.delegate.close();
}
public static BurnOnCloseFileIterator wrap(Iterator iter) {
return new BurnOnCloseFileIterator(iter, new Function() {
public String apply(String s) {
return s;
}
});
}
public static BurnOnCloseFileIterator wrap(Iterator iter, File backingFile) {
return new BurnOnCloseFileIterator(iter, backingFile, new Function() {
public String apply(String s) {
return s;
}
});
}
private static class Impl extends AbstractIterator implements Closeable {
private final Iterator iterator;
private final Function transformer;
private final File backingFile;
public Impl(Iterator iterator, File backingFile, Function transformer) {
this.iterator = iterator;
this.transformer = transformer;
this.backingFile = backingFile;
}
@Override
protected T computeNext() {
if (iterator.hasNext()) {
return transformer.apply(iterator.next());
}
try {
close();
} catch (IOException e) {
LOG.warn("Error closing iterator", e);
}
return endOfData();
}
@Override
public void close() throws IOException {
if (iterator instanceof Closeable) {
closeQuietly((Closeable) iterator);
}
if (backingFile != null && backingFile.exists()) {
forceDelete(backingFile);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy