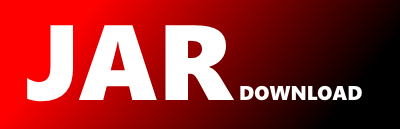
org.apache.jackrabbit.spi.commons.query.QueryNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.jackrabbit.spi.commons.query;
import javax.jcr.RepositoryException;
/**
* Implements an abstract base class for nodes of a query tree that represents
* a query. The query tree is independent from the query syntax which is used
* to search the repository.
*/
public abstract class QueryNode {
/** Type value for {@link QueryRootNode} */
public static final int TYPE_ROOT = 1;
/** Type value for {@link RelationQueryNode} */
public static final int TYPE_RELATION = 2;
/** Type value for {@link OrderQueryNode} */
public static final int TYPE_ORDER = 3;
/** Type value for {@link TextsearchQueryNode} */
public static final int TYPE_TEXTSEARCH = 4;
/** Type value for {@link ExactQueryNode} */
public static final int TYPE_EXACT = 5;
/** Type value for {@link NodeTypeQueryNode} */
public static final int TYPE_NODETYPE = 6;
/** Type value for {@link AndQueryNode} */
public static final int TYPE_AND = 7;
/** Type value for {@link OrQueryNode} */
public static final int TYPE_OR = 8;
/** Type value for {@link NotQueryNode} */
public static final int TYPE_NOT = 9;
/** Type value for {@link LocationStepQueryNode} */
public static final int TYPE_LOCATION = 10;
/** Type value for {@link PathQueryNode} */
public static final int TYPE_PATH = 11;
/** Type value for {@link DerefQueryNode} */
public static final int TYPE_DEREF = 12;
/** Type value for {@link PropertyFunctionQueryNode} */
public static final int TYPE_PROP_FUNCTION = 13;
/**
* References the parent of this QueryNode
. If this is the root
* of a query tree, then parent
is null
.
*/
private final QueryNode parent;
/**
* Constructs a new QueryNode
with a reference to it's parent.
*
* @param parent the parent node, or null
if this is the root
* node of a query tree.
*/
public QueryNode(QueryNode parent) {
this.parent = parent;
}
/**
* Returns the parent QueryNode
or null
if this is
* the root node of a query tree.
*
* @return the parent QueryNode
or null
if this is
* the root node of a query tree.
*/
public QueryNode getParent() {
return parent;
}
/**
* Dumps this QueryNode and its child nodes to a String.
* @return the query tree as a String.
* @throws RepositoryException
*/
public String dump() throws RepositoryException {
StringBuffer tmp = new StringBuffer();
QueryTreeDump.dump(this, tmp);
return tmp.toString();
}
/**
* Accepts a {@link QueryNodeVisitor} and calls the appropriate visit
* method on the visitor depending on the concrete implementation of
* this QueryNode
.
*
* @param visitor the visitor to call back.
* @param data arbitrary data for the visitor.
* @return the return value of the visitor.visit()
call.
* @throws RepositoryException
*/
public abstract Object accept(QueryNodeVisitor visitor, Object data) throws RepositoryException;
/**
* Returns the type of this query node.
* @return the type of this query node.
*/
public abstract int getType();
/**
* Returns true
if obj
is the same type of
* QueryNode
as this
node and is equal to
* this
node.
* @param obj the reference object with which to compare.
* @return true
if obj
is equal to
* this
; false
otherwise.
*/
public abstract boolean equals(Object obj);
/**
* Returns true
if this query node needs items under
* /jcr:system to be queried.
*
* @return true
if this query node needs content under
* /jcr:system to be queried; false
otherwise.
*/
public abstract boolean needsSystemTree();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy