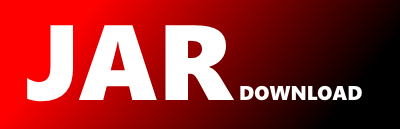
com.adobe.cq.dam.cfm.ContentElement Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2015 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and may be covered by U.S. and Foreign Patents,
* patents in process, and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*/
package com.adobe.cq.dam.cfm;
import aQute.bnd.annotation.ProviderType;
import org.apache.sling.api.adapter.Adaptable;
import org.jetbrains.annotations.Nullable;
import java.util.Iterator;
/**
* Implementations of this interface allow accessing elements of a content fragment in a
* way agnostic to the underlying data structures.
*
* Each content element consists of a set of 0..n variations, the element's content
* being kind of a "master" variation all other variations derive from.
*
* A content element is defined to consist of textual content. The
* {@link ContentFragment} interface is agnostic to a specific MIME type, so it's up to
* the implementation to decide when and how to transform the text to a format that's
* suitable for output.
*
* Transactional behavior: The caller is responsible for committing the respective
* {@link org.apache.sling.api.resource.ResourceResolver} after calling one or more
* methods that change a content element unless specified otherwise.
*/
@ProviderType
public interface ContentElement extends Adaptable, Versionable {
/**
* Gets an iterator on the currently available variations of the element.
*
* @return iterator on variations
*/
Iterator getVariations();
/**
* Gets the variation of the given name.
*
* @param variationName The name of the variation
* @return The variation; null
if no variation of the given name exists
*/
@Nullable
ContentVariation getVariation(String variationName);
/**
* Creates a new variation of the element from the specified template.
*
* The initial content of the new variation must be a copy of the current element
* content.
*
* If the method is called multiple times with the same template, two different
* variations have to created (with different names/identifiers).
*
* The specified template should be part of a {@link FragmentTemplate}.
*
* @param template The template
* @return The variation created
* @throws ContentFragmentException if the variation could not be created
*/
ContentVariation createVariation(VariationTemplate template)
throws ContentFragmentException;
/**
* Removes the specified variation.
*
* If the specified variation is not a member of the element, a
* {@link IllegalArgumentException} has to be thrown.
*
* @param variation The variation to remove
* @throws ContentFragmentException if the variation could not be removed on the
* persistence level
* @throws IllegalArgumentException if the specified variation is not part of this
* element
*/
void removeVariation(ContentVariation variation) throws ContentFragmentException;
/**
* Gets the resolved variation for the given name.
*
* The method must be able to handle names that do not refer to actually existing
* variations. Instead, it has to handle this case in a well defined manner - usually
* by falling back to another, actually existing variation or to the element's
* default content.
*
* From a client's perspective, this method should be used to determine content
* in any kind of "publishing context" - be it in the page authoring context or some
* other context that prepares the final content to be presented to the user.
*
* How the resolution is eventually done is an implementation detail.
*
* @param variationName The name of the variation; may be null
(which
* will return null
to signal that default content
* has to be used)
* @return The resolved variation; null
if there's no suitable variation
* and the element's default content has to be used
*/
ContentVariation getResolvedVariation(String variationName);
/**
* Gets the (technical) name of the content element.
*
* @return The name of the content element
*/
String getName();
/**
* Gets the (human-readable) title of the content element.
*
* @return The title of the content element
*/
String getTitle();
/**
* Gets the current value of the element in a suitable object representation.
*
* Note that if you change the returned {@link FragmentData}, you will have to
* call {@link #setValue(FragmentData)} explicitly after manipulating the value.
*
* {@link DataType} provides additional information about how values are handled
* by the system.
*
* @return The value
* @since 1.1
*/
FragmentData getValue();
/**
* Sets the current value of the element in the provided object representation.
*
* Please see {@link DataType} for more information about what "object
* representation" means.
*
* @param object The value
* @throws ContentFragmentException if the provided value couldn't be persisted or
* doesn't match the data type of the element
* @since 1.1
*/
void setValue(FragmentData object) throws ContentFragmentException;
/**
* Gets the content of the content element in a suitable text representation.
*
* @return The content of the content element in a text representation
*/
String getContent();
/**
* Gets the MIME type of the content element.
*
* @return The MIME type; refers to some text format
*/
String getContentType();
/**
* Sets the content of the content element from the provided text value.
*
* The specified content & its MIME type must be textual. What MIME types are
* eventually supported is left to the implementation.
*
* For non-textual data types (added in 1.1), a MIME type of text/plain
* should be specified. The implementation is then responsible for translating the
* provided text value into a suitable object representation (or throw a
* {@link ContentFragmentException} if this is not possible). How the text value is
* converted into a suitable object value is left to the implementation. But it is
* required that a value returned by {@link #getContent()} can successfully be
* converted by {@link #setContent(String, String)}. In other words,
* {@code el.setContent(el.getContent(), el.getContentType()} must not throw a
* {@code ContentFragmentException} because of type conversion problems.
*
* @param content The new content of the content element
* @param contentType The new content type
* @throws ContentFragmentException if the content could not be written or the provided
* text could not be converted to the data type of the
* element correctly
* @throws IllegalArgumentException if the specified MIME type is not supported or not
* a textual MIME type
*/
void setContent(String content, String contentType) throws ContentFragmentException;
}