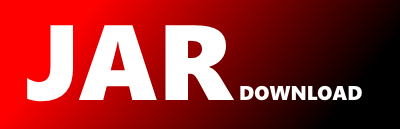
com.adobe.fontengine.font.Matrix Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: Matrix.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font;
/**
* A 2x3 "Postscript style" coordinate matrix (a 2D transformation and shift).
*
* Synchronization
*
* These objects are immutable.
*/
final public class Matrix {
/** a constant Matrix representing the identity transformation */
public final static Matrix IDENTITY_MATRIX = new Matrix(1,0,0,1,0,0);
public final double a;
public final double b;
public final double c;
public final double d;
public final double tx;
public final double ty;
/**
* Creates a matrix from either a 4 or 6 element array representing a matrix.
* If there are 4 elements, tx and ty are set to 0.
*
* @param matrix contains the matrix elements in the following order: a, b, c, d, tx, ty
*/
public Matrix(double[] matrix)
{
a = matrix[0];
b = matrix[1];
c = matrix[2];
d = matrix[3];
tx = matrix.length > 4 ? matrix[4]:0;
ty = matrix.length > 5 ? matrix[5]:0;
}
public Matrix(double a, double b, double c, double d, double tx, double ty)
{
this.a = a;
this.b = b;
this.c = c;
this.d = d;
this.tx = tx;
this.ty = ty;
}
/**
* Returns true iff this is very close to representing the identity transformation.
*/
public boolean isIdentity()
{
return (Math.abs(a - 1) < 0.001 && Math.abs(d - 1) < 0.001
&& Math.abs(b) < 0.001 && Math.abs(c) < 0.001
&& Math.abs(tx) < 0.001 && Math.abs(ty) < 0.001);
}
/**
* Checks whether 'inverse' is the inverse of this matrix.
* @param inverse the matrix to be compared
* @return true iff this * inverse ~= IDENTITY_MATRIX
*/
public boolean isInverse(Matrix inverse)
{
Matrix res = multiply(inverse);
return res.isIdentity();
}
/** Computes this * multiplier and returns the resulting matrix. */
public Matrix multiply(Matrix multiplier)
{
double aRes,bRes,cRes,dRes,txRes,tyRes;
aRes = this.a * multiplier.a + this.b * multiplier.c;
bRes = this.a * multiplier.b + this.b * multiplier.d;
cRes = this.c * multiplier.a + this.d * multiplier.c;
dRes = this.c * multiplier.b + this.d * multiplier.d;
txRes = this.tx * multiplier.a + this.ty * multiplier.c + multiplier.tx;
tyRes = this.tx * multiplier.b + this.ty * multiplier.d + multiplier.ty;
return new Matrix(aRes,bRes,cRes,dRes,txRes,tyRes);
}
/** Computes this * multiplier and returns the resulting matrix. */
public Matrix multiply (double multiplier) {
return new Matrix (a * multiplier, b * multiplier, c * multiplier,
d * multiplier, tx * multiplier, ty * multiplier);
}
/** computes p * this and returns the result in p */
public void applyToPoint(Point p)
{
// shortcut the process if we are looking at the identity matrix.
if (this.equals(IDENTITY_MATRIX))
return;
double origX = p.x;
double origY = p.y;
p.x = origX * a + origY * c + tx;
p.y = origX * b + origY * d + ty;
}
/** computes [x y] * this and returns the resulting x value */
public double applyToXYGetX(double x, double y)
{
return x*a + y*c + tx;
}
/** computes [x y] * this and returns the resulting y value */
public double applyToXYGetY(double x, double y)
{
return x*b + y*d + ty;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy