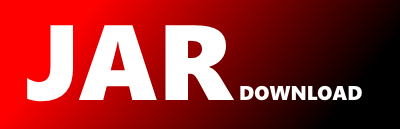
com.adobe.fontengine.font.OutlineConsumer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: OutlineConsumer.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2007 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font;
/** Receives events for unhinted beziers representing glyphs.
*
* There is one event for lines, one event for curves, and one event
* for moves. The path will be closed after the final event. The
* fill will be to the left when walking in the direction of the path
* unless the glyph is malformed.
*
* The coordinates are in the design space of the font.
*/
public interface OutlineConsumer {
/**
* Tells the OutlineConsumer the matrix to be applied to subsequent
* points in order to get to a 1 ppem size. It is possible for setMatrix
* to be called multiple times for a single glyph. Each call replaces the
* previous matrix. It is guaranteed that setMatrix will be called prior to
* any other methods.
*
* @param newMatrix
*/
public void setMatrix (Matrix newMatrix);
/**
* Called to set a new currentpoint. If lineto or curveto was
* previously been called and was the most recent function called,
* an implied lineto should precede this move
* and should close the previous segment by connecting the last point in the
* most recent line/curve with the point that started the last segment.
* @param x the x coordinate of the new currentpoint
* @param y the y coordinate of the new currentpoint
*/
public void moveto (double x, double y);
/** Called for a line.
* The line goes from the current point to
* (x
, y
). After this call, the currentpoint
* should be x,y
*/
public void lineto (double x, double y);
/** Called for a cubic curve.
* The control points are the current point,
* (x2
, y2
),
* (x3
, y3
),
* (x4
, y4
). After this call, the
* currentpoint should be x4, y4.
*/
public void curveto (double x2, double y2,
double x3, double y3, double x4, double y4);
/** Called for a quadratic curve.
* The control points are the current point,
* (x2
, y2
),
* (x3
, y3
). After this call, the
* currentpoint should be x3, y3.
*/
public void curveto (double x2, double y2,
double x3, double y3);
/** Called to signal the end of a glyph's outline.
*
* The last path will already be closed by the time this is called.
*/
public void endchar();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy