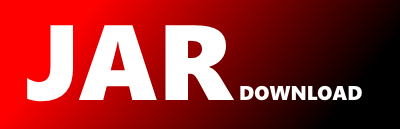
com.adobe.fontengine.font.cff.FontFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
*
* File: FontFactory.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.font.cff;
import java.io.IOException;
import com.adobe.fontengine.font.FontByteArray;
import com.adobe.fontengine.font.InvalidFontException;
import com.adobe.fontengine.font.UnsupportedFontException;
/** Creates font objects for naked cff fonts */
final public class FontFactory {
/**
* Given buffer of font data, creates cff FontData objects that represent it.
*
* Note that fonts are parsed at this point.
*
* @return An array of cff fonts
* @throws IOException Thrown if the stream cannot be read
* @throws InvalidFontException Thrown if the stream does not represent a valid cff font.
* @throws UnsupportedFontException Thrown if the stream represents an unsupported cff font.
*/
public static CFFFont[] load (FontByteArray buffer)
throws IOException, InvalidFontException, UnsupportedFontException {
return new FontSet (buffer).fonts;
}
/**
* @return the minimum number of bytes needed to try to identify
* whether a font stream contains cff
*/
public static int getNumBytesNeededToIdentify() {
return 4;
}
/**
* This is a very loose check to see if the font looks like
* CFF. Since these fonts don't have as solid of a way of identifying
* them as other fonts do, this check should only be used when checks
* for other fonttypes have failed.
*
* @param startingBytes bytes from the start of the font stream. Must
* contain at least getNumBytesNeededToIdentify bytes.
*
* @return true of the font appears to be cff. false otherwise.
*/
public static boolean isCFF(byte[] startingBytes) {
// This is hokey, but there isn't much we can
// do to identify these fonts. We look at the major version
// and the offset size. If both look reasonable, we will assume
// it is cff. The parse should fail later if we give a false positive.
if ((startingBytes[0] == 1)
&& (startingBytes[3] > 0 && startingBytes[3] <= 4)) {
return true; }
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy