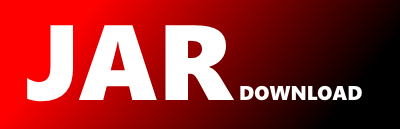
com.adobe.fontengine.fontmanagement.FontProxy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: FontProxy.java
*
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2004-2006 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.fontmanagement;
import java.io.Serializable;
import com.adobe.fontengine.font.Font;
import com.adobe.fontengine.font.FontDescription;
/**
* FontProxy provides the class used for storing a FontDescription
and
* a Font
together in a database for retrieval. This is the standard
* way that CAFE databases will store fonts.
*/
final public class FontProxy implements Serializable
{
/* Serialization signature is explicitly set and should be
* incremented on each release to prevent compatibility.
*/
static final long serialVersionUID = 1;
FontDescription fontDesc;
Font font;
public FontProxy(FontDescription fontDesc, Font font)
{
this.fontDesc = fontDesc;
this.font = font;
}
/**
* @return the Font
*/
public Font getFont()
{
return this.font;
}
/**
* @return the FontDescription
*/
public FontDescription getFontDescription()
{
return this.fontDesc;
}
public boolean equals(Object obj)
{
if (obj != null)
{
if (this == obj)
{
return true;
}
if (obj instanceof FontProxy)
{
if ( (this.fontDesc.equals(((FontProxy)obj).fontDesc))
&& (this.font.equals(((FontProxy)obj).font)))
{
return true;
}
}
}
return false;
}
public int hashCode()
{
return this.font.hashCode() ^ this.fontDesc.hashCode();
}
public String toString () {
return fontDesc.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy