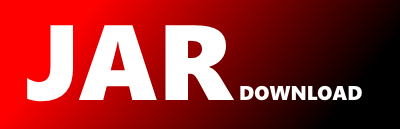
com.adobe.fontengine.inlineformatting.infontformatting.OriyaFormatter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: OriyaFormatter.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is
* obtained from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.inlineformatting.infontformatting;
import com.adobe.fontengine.inlineformatting.AttributedRun;
final class OriyaFormatter extends IndicFormatter {
protected int splitVowelsAndNormalize (AttributedRun run, int start, int limit) {
while (start < limit) {
int usv = run.elementAt (start);
switch (usv) {
case 0x0B48: {
run.replace (start, new int[] {0x0B47, 0x0B56});
limit++;
start += 2;
break; }
case 0x0B4B: {
run.replace (start, new int[] {0x0B47, 0x0B3E});
limit++;
start += 2;
break; }
case 0x0B4C: {
run.replace (start, new int[] {0x0B47, 0x0B57});
limit++;
start += 2;
break; }
default: {
start++; }}}
return limit;
}
/* @see com.adobe.fontengine.inlineformatting.infontformatting.OTIndicFormatter#nukta()
*/
protected int nukta () {
return 0x0B3C;
}
/* @see com.adobe.fontengine.inlineformatting.infontformatting.OTIndicFormatter#virama()
*/
protected int virama () {
return 0x0B4D;
}
/* @see com.adobe.fontengine.inlineformatting.infontformatting.OTIndicFormatter#isConsonant(int)
*/
protected boolean isConsonant (int usv) {
return ( 0xB15 <= usv && usv <= 0xB39
|| 0xB5C <= usv && usv <= 0xB61
|| 0xB71 == usv
|| 0x25cc == usv);
}
/* @see com.adobe.fontengine.inlineformatting.infontformatting.OTIndicFormatter#hasNukta(int)
*/
protected boolean hasNukta (int usv) {
return ( 0xB5C == usv
|| 0xB5D == usv);
}
/* @see com.adobe.fontengine.inlineformatting.infontformatting.OTIndicFormatter#removeNukta(int)
*/
protected int removeNukta (int usv) {
switch (usv) {
case 0xB5C: return 0xB21;
case 0xB5D: return 0xB22;
default: return usv; }
}
/* @see com.adobe.fontengine.inlineformatting.infontformatting.OTIndicFormatter#isMark(int)
*/
protected boolean isMark (int usv) {
return ( 0x0B3E <= usv && usv <= 0x0B4C
|| 0x0B56 <= usv && usv <= 0x0B57
|| 0x0B01 <= usv && usv <= 0xB03);
}
/* @see com.adobe.fontengine.inlineformatting.infontformatting.OTIndicFormatter#isIndependentVowel(int)
*/
protected boolean isIndependentVowel (int usv) {
return ( 0x0B05 <= usv && usv <= 0x0B14
|| 0x0B60 <= usv && usv <= 0x0B61
|| 0x25cc == usv);
}
protected Position getPosition (int usv) {
switch (usv) {
case 0xB47: return Position.left;
case 0xB01: return Position.topOther;
case 0xB41:
case 0xB42:
case 0xB43: return Position.bottom;
case 0xB3E:
case 0xB40:
case 0xB57: return Position.rightMatra;
case 0xB3F:
case 0xB56: return Position.topMatra;
case 0xB02:
case 0xB03: return Position.rightOther;
default: return Position.any; }
}
protected Shape rephLike (int usv) {
if (usv == 0xb30) {
return Shape.rephCons; }
else {
return Shape.any; }
}
protected boolean subjoins (int usv) {
return usv == 0xB23 || usv == 0xB24 || usv == 0xb28 || usv == 0xb2c
|| usv == 0xb2d || usv == 0xb2e || usv == 0xb30 || usv == 0xb32
|| usv == 0xb33 || usv == 0xb35;
}
protected boolean postjoins (int usv) {
return usv == 0xb2f || usv == 0xb5f;
}
protected boolean postjoinsIndependentVowels (int usv) {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy