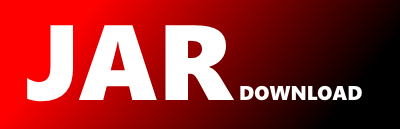
com.adobe.fontengine.math.F16Dot16 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: F16Dot16.java
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is
* obtained from Adobe Systems Incorporated.
*
*/
package com.adobe.fontengine.math;
import com.adobe.agl.text.DecimalFormat;
/** Constants and operations on fixed 16.16 numbers.
*
* The value v is represented by the int
value
* 2^16 * v .
*/
public class F16Dot16 {
public static final int /*16.16*/ ZERO = 0;
public static final int /*16.16*/ ONE = 1 << 16;
public static final int /*16.16*/ ONE_HALF = ONE / 2;
/** Clamp a long value to the int range.*/
static private int clamp (long x) {
if (x > Integer.MAX_VALUE) {
return Integer.MAX_VALUE; }
else if (x < Integer.MIN_VALUE) {
return Integer.MIN_VALUE; }
else {
return (int) x; }
}
/** Truncate the decimal part of a 16.16, result is a 16.16. */
public static int /*16.16*/ truncate (int /*16.16*/ v) {
return v & 0xffff0000;
}
/** Multiply two 16.16, result is a 16.16. */
public static int /*16.16*/ multiply (int /*16.16*/ v1, int /*16.16*/ v2) {
return clamp (((long) v1 * (long) v2) >> 16);
}
/** Square of a 16.16, result is a 16.16. */
public static int /*16.16*/ square (int /*16.16*/ v) {
return multiply (v, v);
}
/** Multiply a 16.16 by a 2.14, result is 16.16. */
public static int /*16.16*/ multiplyByF2Dot14 (int /*16.16*/ v1, int /*2.14*/ v2) {
return clamp (((((long) v1 * (long) v2) >> 13) + 1) >> 1);
}
/** Multiply a 16.16 by a 16.16 and divive by a 16.16, result is a 16.16. */
public static int /*16.16*/ multiplyDivide (int /*16.16*/ v1, int /*16.16*/ v2, int /*16.16*/ v3) {
long numerator = ((long) v1) * ((long) v2);
long denominator = v3;
boolean negate = false;
if (numerator < 0) {
numerator = - numerator;
negate = ! negate; }
if (denominator < 0) {
denominator = - denominator;
negate = ! negate; }
long v = (numerator + denominator / 2) / denominator;
if (negate) {
return clamp (-v); }
else {
return clamp (v); }
}
/** Divide a 16.16 by a 16.16, result is a 16.16. */
public static int /*16.16*/ divide (int /*16.16*/ v1, int /*16.16*/ v2) {
return clamp ((((long) v1 << 16) / v2));
}
/** Round a 16.16, half-up , result is a 16.16. */
public static int /*16.16*/ round (int /*16.16*/ v) {
return truncate (v + ONE_HALF);
}
/** Convert a 16.16 to a double. */
public static double toDouble (int /*16.16*/ v) {
return ((double) v) / 0x10000;
}
/** Convert a double to a 16.16. */
public static int /*16.16*/ fromDouble (double v) {
return (int) (v * ONE);
}
/** Convert an int to a 16.16. */
public static int /*16.16*/ fromInt (int v) {
return v << 16;
}
private final static DecimalFormat df = new DecimalFormat ("0.###");
/** Convert a 16.16 to a decimal representation. */
public static String toString (int /*16.16*/ v) {
return df.format (toDouble (v));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy