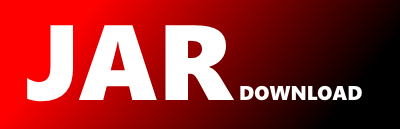
com.adobe.granite.taskmanagement.Condition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* ADOBE CONFIDENTIAL
* __________________
*
* Copyright 2012 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.granite.taskmanagement;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
/**
* Used to specify conditions in a {@link Filter} for a TaskManagement query
*/
public class Condition implements Cloneable {
/**
* Used to define the task property names of the condition
*/
private List propertyNames = new ArrayList();
/**
* Used to define the ComparisonOperator of the condition
*/
private ComparisonOperator comparisonOperator;
/**
* Used to define the comparison value of the condition
*/
private Object comparisonValue;
/**
* Built-in task properties
*/
private List taskProperties = new ArrayList();
/**
* Construct a new Condition instance with null property,
* comparisonOperator and comparisonValue fields.
*/
public Condition() {
}
/**
* Get the name of the task property for this condition.
*
* @return the task property name.
*/
public String getPropertyName() {
if (taskProperties.size() == 1) {
return taskProperties.get(0).getPropertyName();
} else if (propertyNames.size() == 1) {
return propertyNames.get(0);
}
return null;
}
/**
* Set the name of the task property for this condition.
* you may either set a taskProperty or a #setTaskProperty not both
*
* @param aPropertyName the task property name.
*/
public void setPropertyName(String aPropertyName) {
propertyNames.clear();
propertyNames.add(aPropertyName);
}
/**
* Gets the names of the task properties for this condition
*
* @return the task property names or {@code null}
*/
public String[] getPropertyNames() {
if (!taskProperties.isEmpty()) {
List names = new ArrayList();
for (TaskProperty taskProperty : taskProperties) {
names.add(taskProperty.getPropertyName());
}
return names.toArray(new String[]{});
} else if (!propertyNames.isEmpty()) {
return propertyNames.toArray(new String[]{});
}
return null;
}
/**
* Set the names of the task properties for this condition. You may either
* use {@link #setPropertyNames(String...)} or
* {@link #setTaskProperties(TaskProperty...)} but not both.
*
* @param propertyNames
* the task property names
*/
public void setPropertyNames(String... propertyNames) {
this.propertyNames.clear();
this.propertyNames.addAll(Arrays.asList(propertyNames));
}
/**
* gets the taskProperty set for this condition
* @return the taskProperty
*/
public TaskProperty getTaskProperty() {
if (taskProperties.size() == 1) {
return taskProperties.get(0);
}
return null;
}
/**
* sets a built-in task property for this condition.
* you may either set a taskProperty or a #setPropertyName not both
* @param taskProperty the built in task property for this condition
*/
public void setTaskProperty(TaskProperty taskProperty) {
taskProperties.clear();
taskProperties.add(taskProperty);
}
/**
* Gets the task properties set for this condition.
*
* @return the task properties
*/
public TaskProperty[] getTaskProperties() {
return taskProperties.toArray(new TaskProperty[]{});
}
/**
* Sets built-in task properties for this condition. You may either use
* {@link #setTaskProperties(TaskProperty...)} or
* {@link #setPropertyNames(String...)} but not both.
*
* @param taskProperties
* the built-in task properties for this condition
*/
public void setTaskProperties(TaskProperty... taskProperties ) {
this.taskProperties.clear();
this.taskProperties.addAll(Arrays.asList(taskProperties));
}
/**
* Get the ComparisonOperator object for this condition.
*
* @return The ComparisonOperator for this condition or null if no ComparisonOperator
* has been set.
*/
public ComparisonOperator getComparisonOperator() {
return this.comparisonOperator;
}
/**
* Set the ComparisonOperator object for this condition
*
* @param aComparisonOperator The ComparisonOperator object to set
*/
public void setComparisonOperator(ComparisonOperator aComparisonOperator) {
this.comparisonOperator = aComparisonOperator;
}
/**
* Get the comparison value Object for this condition.
*
* @return The comparison value as an Object for this condition or null if no comparison value
* has been set.
*/
public Object getComparisonValue() {
return this.comparisonValue;
}
/**
* Set the comparison value Object for this condition
*
* @param aComparisonValue The comparison value Object to set
*/
public void setComparisonValue(Object aComparisonValue) {
this.comparisonValue = aComparisonValue;
}
@Override
public Object clone() throws CloneNotSupportedException {
return super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy