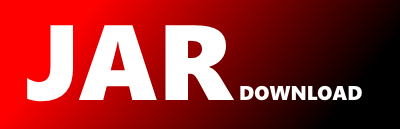
com.adobe.granite.ui.components.ExpressionHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2013 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.adobe.granite.ui.components;
import java.util.Locale;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import javax.servlet.ServletRequest;
import javax.servlet.ServletRequestWrapper;
import javax.servlet.jsp.PageContext;
import org.apache.sling.api.SlingHttpServletRequest;
/**
* A helper to deal with EL easier.
*/
public class ExpressionHelper {
private ExpressionResolver resolver;
@Nonnull
private SlingHttpServletRequest request;
@SuppressWarnings("null")
public ExpressionHelper(@Nonnull ExpressionResolver resolver, @Nonnull PageContext pageContext) {
this(resolver, toSlingRequest(pageContext.getRequest()));
}
public ExpressionHelper(@Nonnull ExpressionResolver resolver, @Nonnull SlingHttpServletRequest servletRequest) {
this.resolver = resolver;
this.request = servletRequest;
}
/**
* Resolves the given expression as string. Request's locale will be used.
*
* @param expression
* the expression to be resolved
* @return the resolved expression as a string, or {@code null} when expression
* is {@code}
*/
public String getString(@CheckForNull String expression) {
return get(expression, String.class);
}
/**
* Resolves the given expression as string, with the given locale.
*
* @param expression
* the expression to be resolved
* @param locale
* the locale
* @return the resolved expression as a string, or {@code null} when expression
* is {@code}
*/
public String getString(@CheckForNull String expression, @Nonnull Locale locale) {
return get(expression, locale, String.class);
}
/**
* Resolves the given expression as boolean. Request's locale will be used.
*
* @param expression
* the expression to be resolved
* @return the resolved expression as a boolean, or {@code null} when expression
* is {@code}
*/
public boolean getBoolean(@CheckForNull String expression) {
Boolean result = get(expression, Boolean.class);
if (result == null) {
return false;
} else {
return result;
}
}
/**
* Resolves the given expression as boolean, with the given locale.
*
* @param expression
* the expression to be resolved
* @param locale
* the locale
* @return the resolved expression as a boolean, or {@code null} when expression
* is {@code}
*/
public boolean getBoolean(@CheckForNull String expression, @Nonnull Locale locale) {
Boolean result = get(expression, locale, Boolean.class);
if (result == null) {
return false;
} else {
return result;
}
}
/**
* Resolves the given expression. Request's locale will be used.
*
* @param expression
* the expression to be resolved
* @param expectedType
* the expected type of the resolved expression
* @param
* the type of the resolved expression
* @return the resolved expression, or {@code null} when expression is {@code}
*/
@SuppressWarnings("null")
public T get(@CheckForNull String expression, @Nonnull Class expectedType) {
return get(expression, request.getLocale(), expectedType);
}
/**
* Resolves the given expression, with the given locale.
*
* @param expression
* the expression to be resolved
* @param locale
* the locale
* @param expectedType
* the expected type of the resolved expression
* @param
* the type of the resolved expression
* @return the resolved expression, or {@code null} when expression is {@code}
*/
public T get(@CheckForNull String expression, @Nonnull Locale locale, @Nonnull Class expectedType) {
if (expression == null) {
return null;
}
return resolver.resolve(expression, locale, expectedType, request);
}
@SuppressWarnings("null")
@Nonnull
private static SlingHttpServletRequest toSlingRequest(@Nonnull ServletRequest req) {
while (!(req instanceof SlingHttpServletRequest)) {
if (req instanceof ServletRequestWrapper) {
req = ((ServletRequestWrapper) req).getRequest();
} else {
throw new IllegalStateException("request wrong class");
}
}
return (SlingHttpServletRequest) req;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy