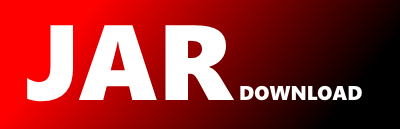
com.adobe.granite.ui.components.PagingIterator Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*************************************************************************
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2014 Adobe
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe
* and its suppliers and are protected by all applicable intellectual
* property laws, including trade secret and copyright laws.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe.
**************************************************************************/
package com.adobe.granite.ui.components;
import java.util.Iterator;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
/**
* A wrapper iterator to supports paging.
*
* If you have access to Collection 4.1, it is recommended to use
* {@code IteratorUtils#boundedIterator(Iterator, long, long)} instead.
*/
public class PagingIterator implements Iterator {
private Iterator it;
private Integer offset;
private Integer limit;
private int limitCounter = 0;
/**
* Instantiates a new paging wrapper of the given iterator.
*
* The iterator is iterated immediately as per {@code offset}.
*
* @param it
* The iterator to wrap
* @param offset
* The offset of the paging; {@code null} to do paging without
* offset.
* @param limit
* The limit of the paging; {@code null} to do paging without limit.
* @throws IllegalArgumentException
* When either offset or limit is negative
*/
public PagingIterator(@Nonnull Iterator it, @CheckForNull Integer offset, @CheckForNull Integer limit)
throws IllegalArgumentException {
this.it = it;
this.offset = offset;
this.limit = limit;
if (offset != null && offset < 0) {
throw new IllegalArgumentException("offset is negative");
}
if (limit != null && limit < 0) {
throw new IllegalArgumentException("limit is negative");
}
doOffset();
}
private void doOffset() {
if (offset == null) {
return;
}
for (int i = 0; i < offset && it.hasNext(); i++) {
it.next();
}
}
@Override
public boolean hasNext() {
if (limit == null) {
return it.hasNext();
}
return limitCounter < limit && it.hasNext();
}
@Override
public E next() {
E next = it.next();
limitCounter++;
return next;
}
@Override
public void remove() {
it.remove();
}
}