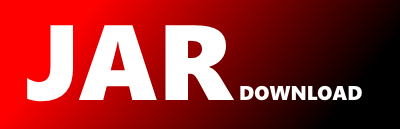
com.adobe.internal.io.DataBufferByteWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
package com.adobe.internal.io;
import java.awt.image.DataBuffer;
import java.io.IOException;
/**
* An implementation of the DataBuffer abstract class which wraps a ByteWriter inside it.
* All the data written to the DataBuffer will actually be read from and written to the byteWriter.
* This class is used for the BufferedImages which has the raster data in the DataBuffers.
*/
public class DataBufferByteWriter extends DataBuffer
{
private ByteWriter byteWriter;
/**
* @param byteWriter
* @param dataType
* @param size
*/
public DataBufferByteWriter (ByteWriter byteWriter, int dataType, int size)
{
super(dataType,size);
this.byteWriter = byteWriter;
}
/**
* @see java.awt.image.DataBuffer#getElem(int, int)
*/
public int getElem(int bank, int i)
{
try {
return byteWriter.read(i);
} catch (IOException e) {
//Ignoring exception
}
return 0;
}
/**
* @see java.awt.image.DataBuffer#setElem(int, int, int)
*/
public void setElem(int bank, int i, int val)
{
try {
byteWriter.write(i,val);
} catch (IOException e) {
//Ignoring exception
}
}
/**
* @see com.adobe.internal.io.ByteReader#length()
*/
public long length()
throws IOException
{
return byteWriter.length();
}
/**
* @see com.adobe.internal.io.ByteReader#close()
*/
public void close()
throws IOException
{
byteWriter.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy