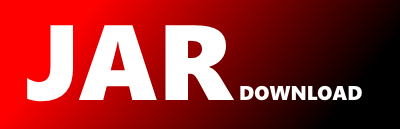
com.adobe.internal.io.stream.BitInputStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* File: BitInputStream.java
*
*************************************************************************
** ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2012 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.internal.io.stream;
import java.io.IOException;
import java.io.InputStream;
import java.security.InvalidParameterException;
/**
* This class reads bit by bit data from a back-end stream which is passed in constructor.
* @author hkansal
*
*/
public class BitInputStream{
private static final int BUFFER_SIZE = 512;
private InputStream stream = null;
private int currentBitPos = 0;
private byte[] buffer = null;
private int currentBytePos = 0;
private int maxBytesLimit = BUFFER_SIZE;
/**
* Reads chunk of data on demand which is cached for further
* bit by bit reading.
* @throws IOException
*/
private void fillBuffer() throws IOException{
if(stream.available() > 0)
maxBytesLimit = stream.read(buffer);
else
maxBytesLimit = -1;
currentBytePos = 0;
}
/**
* Constructor for this stream.
* @param stream This stream is used as back-end stream to read bit by bit data.
* @throws IOException
*/
public BitInputStream(InputStream stream) throws IOException{
this.stream = stream;
}
private int readData(int nob) throws IOException{
if(maxBytesLimit == -1)
throw new IOException("End of stream has been reached so no data can be read now.");
if(currentBytePos == maxBytesLimit)
fillBuffer();// buffer more data to perform this read operation.
int value = (255&(buffer[currentBytePos]<> (Byte.SIZE-nob);
currentBitPos+=nob;
if(currentBitPos >=Byte.SIZE)
currentBytePos++;
currentBitPos%=Byte.SIZE;
return value;
}
/**
* Reads nob number of bits from available data and returns an integer value.
* Throws {@link IOException} if end of stream has been reached.
* @param nob
* @return int
* @throws IOException
*/
public int read(int nob) throws IOException {
if(buffer == null){
buffer = new byte[BUFFER_SIZE];
fillBuffer();
}
int value = 0;
int bitsToRead = 0;
while(nob > 0){
bitsToRead = (nob <= (Byte.SIZE-currentBitPos)?nob:(Byte.SIZE-currentBitPos));
nob-=bitsToRead;
value = (value | (readData(bitsToRead)< b.length){
throw new InvalidParameterException("Byte array passed is either null or insufficient to read the number of bytes actually asked to read.");
}
if(bitsPerValue > Byte.SIZE)
throw new InvalidParameterException("BitsPerValue is " + bitsPerValue + " which is greater than 8 and cann't be fit into byte.");
int i=0;
try{
for(i=0;i b.length){
throw new InvalidParameterException("Byte array passed is either null or insufficient to read the number of bytes actually asked to read.");
}
int i=0;
try{
for(i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy