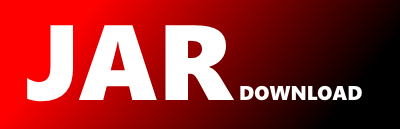
com.adobe.internal.util.ArrayListStack Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/* ****************************************************************************
*
* File: ArrayListStack
*
* ****************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2005 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual
* and technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or
* copyright law. Dissemination of this information or reproduction of this
* material is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
*
* ***************************************************************************/
package com.adobe.internal.util;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.EmptyStackException;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
/**
* @author speri
*
* This class implements the functionality of the Stack.
* default stack implmentation provided in java.util is vector based , and carries a overhead of synchronization.
*/
public class ArrayListStack implements Stack, List, Serializable
{
private ArrayList items;
/**
*
*/
private static final long serialVersionUID = 1L;
/**
* Constructs an empty stack.
*/
public ArrayListStack()
{
items = new ArrayList();
}
/**
* Constructs an empty stack of the size given.
*/
public ArrayListStack(int size)
{
this.items = new ArrayList(size);
}
/**
* Constructs the stack from the ArrayList provided.
*/
public ArrayListStack(ArrayList list)
{
items = list;
}
/**
* returns the underlying ArrayList
*/
public ArrayList getArrayList()
{
return items;
}
/**
* Adds an item to the top of the stack.
* @param elem the item to add.
* @return the item added.
*/
public T push(T elem)
{
items.add(elem);
return elem;
}
/**
* Removes and returns item from the top of the stack.
* @return the former top item.
* @throws EmptyStackException if stack is empty.
*/
public T pop() throws EmptyStackException
{
T obj;
int len = size();
obj = peek();
items.remove(len - 1);
return obj;
}
/**
* Returns item from the top of the stack.
* @return the top item.
* @throws EmptyStackException if stack is empty.
*/
public T peek() throws EmptyStackException
{
int len = size();
if ( len == 0)
{
throw new EmptyStackException();
}
return items.get(len - 1);
}
/**
* Tests if stack is empty.
* @return true if the stack is empty; false otherwise.
*/
public boolean empty()
{
return this.items.isEmpty();
}
/**
* Returns the size of the stack.
* @return the size of the stack.
*/
public int size()
{
return items.size();
}
public void clear()
{
items.clear();
}
public String toString()
{
StringBuilder result = new StringBuilder();
for(int i = size( ) - 1; i >= 0; i--)
{
result.append(items.get( i )).append('\n');
}
return result.toString();
}
/**
* Compares the specified object with this list for equality. Returns
* true if and only if the specified object is also a list, both
* lists have the same size, and all corresponding pairs of elements in
* the two lists are equal. (Two elements e1 and
* e2 are equal if (e1==null ? e2==null :
* e1.equals(e2)).) In other words, two lists are defined to be
* equal if they contain the same elements in the same order.
*
* This implementation first checks if the specified object is this
* list. If so, it returns true; if not, it checks if the
* specified object is a list. If not, it returns false; if so,
* it iterates over both lists, comparing corresponding pairs of elements.
* If any comparison returns false, this method returns
* false. If either iterator runs out of elements before the
* other it returns false (as the lists are of unequal length);
* otherwise it returns true when the iterations complete.
*
* @param obj the object to be compared for equality with this list.
*
* @return true if the specified object is equal to this list.
*/
public boolean equals(Object obj)
{
if (obj == this)
{
return true;
}
if (!(obj instanceof ArrayListStack))
return false;
ArrayListStack oStack = (ArrayListStack)obj;
if(items.size() != oStack.size())
{
return false;
}
ListIterator e1 = items.listIterator();
ListIterator e2 = oStack.getArrayList().listIterator();
while(e1.hasNext() && e2.hasNext())
{
T o1 = e1.next();
T o2 = e2.next();
if (!(o1==null ? o2==null : o1.equals(o2)))
{
return false;
}
}
return !(e1.hasNext() || e2.hasNext());
}
/**
* Returns the hash code value for this list.
*
* This implementation uses exactly the code that is used to define the
* list hash function in the documentation for the List.hashCode
* method.
*
* @return the hash code value for this list.
*/
public int hashCode()
{
int hashCode = 1;
Iterator i = items.iterator();
while (i.hasNext())
{
T obj = i.next();
hashCode = 31*hashCode + (obj==null ? 0 : obj.hashCode());
}
return hashCode;
}
/**
* Returns the 1-based position where an object is on this stack.
* If the object o occurs as an item in this stack, this
* method returns the distance from the top of the stack of the
* occurrence nearest the top of the stack; the topmost item on the
* stack is considered to be at distance 1. The equals
* method is used to compare o to the
* items in this stack.
*
* @param o the desired object.
* @return the 1-based position from the top of the stack where
* the object is located; the return value -1
* indicates that the object is not on the stack.
*/
public int search(T o)
{
int i = items.lastIndexOf(o);
if (i >= 0) {
return size() - i;
}
return -1;
}
/* (non-Javadoc)
* @see java.util.List#add(int, java.lang.Object)
*/
public void add(int index, T element)
{
this.items.add(index, element);
}
/* (non-Javadoc)
* @see java.util.List#add(java.lang.Object)
*/
public boolean add(T element)
{
return this.items.add(element);
}
/* (non-Javadoc)
* @see java.util.List#addAll(java.util.Collection)
*/
public boolean addAll(Collection extends T> collection)
{
return this.items.addAll(collection);
}
/* (non-Javadoc)
* @see java.util.List#addAll(int, java.util.Collection)
*/
public boolean addAll(int index, Collection extends T> collection)
{
return this.items.addAll(index, collection);
}
/* (non-Javadoc)
* @see java.util.List#contains(java.lang.Object)
*/
public boolean contains(Object o)
{
return this.items.contains(o);
}
/* (non-Javadoc)
* @see java.util.List#containsAll(java.util.Collection)
*/
public boolean containsAll(Collection> collection)
{
return this.items.containsAll(collection);
}
/* (non-Javadoc)
* @see java.util.List#get(int)
*/
public T get(int index)
{
return this.items.get(index);
}
/* (non-Javadoc)
* @see java.util.List#indexOf(java.lang.Object)
*/
public int indexOf(Object o)
{
return this.items.indexOf(o);
}
/* (non-Javadoc)
* @see java.util.List#isEmpty()
*/
public boolean isEmpty()
{
return this.items.isEmpty();
}
/* (non-Javadoc)
* @see java.util.List#iterator()
*/
public Iterator iterator()
{
return this.items.iterator();
}
/* (non-Javadoc)
* @see java.util.List#lastIndexOf(java.lang.Object)
*/
public int lastIndexOf(Object o)
{
return this.items.lastIndexOf(o);
}
/* (non-Javadoc)
* @see java.util.List#listIterator()
*/
public ListIterator listIterator()
{
return this.items.listIterator();
}
/* (non-Javadoc)
* @see java.util.List#listIterator(int)
*/
public ListIterator listIterator(int index)
{
return this.items.listIterator(index);
}
/* (non-Javadoc)
* @see java.util.List#remove(int)
*/
public T remove(int index)
{
return this.items.remove(index);
}
/* (non-Javadoc)
* @see java.util.List#remove(java.lang.Object)
*/
public boolean remove(Object o)
{
return this.items.remove(o);
}
/* (non-Javadoc)
* @see java.util.List#removeAll(java.util.Collection)
*/
public boolean removeAll(Collection> collection)
{
return this.items.removeAll(collection);
}
/* (non-Javadoc)
* @see java.util.List#retainAll(java.util.Collection)
*/
public boolean retainAll(Collection> collection)
{
return this.items.retainAll(collection);
}
/* (non-Javadoc)
* @see java.util.List#set(int, java.lang.Object)
*/
public T set(int index, T element)
{
return this.items.set(index, element);
}
/* (non-Javadoc)
* @see java.util.List#subList(int, int)
*/
public List subList(int fromIndex, int toIndex)
{
return this.items.subList(fromIndex, toIndex);
}
/* (non-Javadoc)
* @see java.util.List#toArray()
*/
public Object[] toArray()
{
return this.items.toArray();
}
/* (non-Javadoc)
* @see java.util.List#toArray(java.lang.Object[])
*/
public Object[] toArray(Object[] a)
{
return this.items.toArray(a);
}
}