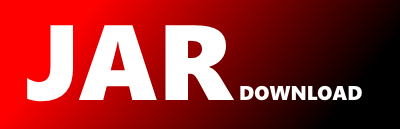
com.adobe.xfa.content.ImageValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.content;
import java.util.ArrayList;
import java.util.List;
import com.adobe.xfa.AppModel;
import com.adobe.xfa.Attribute;
import com.adobe.xfa.Element;
import com.adobe.xfa.EnumAttr;
import com.adobe.xfa.Node;
import com.adobe.xfa.ProtoableNode;
import com.adobe.xfa.STRS;
import com.adobe.xfa.ScriptTable;
import com.adobe.xfa.StringAttr;
import com.adobe.xfa.XFA;
import com.adobe.xfa.ut.Base64;
import com.adobe.xfa.ut.StringUtils;
/**
* A class to represent content
*
* @exclude from published api.
*/
public final class ImageValue extends Content {
private List mpoImageSearchPath;
public ImageValue(Element parent, Node prevSibling) {
super(parent, prevSibling, null, XFA.IMAGE, XFA.IMAGE, null,
XFA.IMAGETAG, XFA.IMAGE);
}
public Attribute defaultAttribute(int eTag) {
// If we're an unspecified property, the default value of contentType is image/*.
if (eTag == XFA.CONTENTTYPETAG)
return new StringAttr(XFA.CONTENTTYPE, "image/*");
// Not a special case, so let the base class handle it
return super.defaultAttribute(eTag);
}
/** @exclude from published api. */
public ProtoableNode createProto(Element parent, boolean bFull) {
ProtoableNode proto = super.createProto(parent, bFull);
if (proto instanceof ImageValue && getAppModel().getIsFragmentDoc()) {
ImageValue imageProto = (ImageValue)proto;
// Fetch the search path from the AppModel (which is populated during fragment load)
// and copy it to this ImageValue. Watson 1490120: also inherit the search path
// from the prototype ImageValue, i.e. "this".
AppModel appModel = getAppModel();
List thisSearchPath = getImageSearchPath();
// JavaPort: modified from C++ to avoid NPE.
List fragmentSearchPath = appModel.getFragmentSearchPath();
if (fragmentSearchPath != null || thisSearchPath != null) {
List concatenated = new ArrayList();
// First inherit the search path from the prototype ImageValue, i.e. "this".
if (thisSearchPath != null) {
concatenated.addAll(thisSearchPath);
}
// Secondly copy the search path from the AppModel.
if (fragmentSearchPath != null && fragmentSearchPath.size() > 0)
concatenated.addAll(fragmentSearchPath);
imageProto.mpoImageSearchPath = concatenated;
}
}
return proto;
}
/**
* @see Content#equals(Object)
* @exclude
*/
public boolean equals(Object object) {
if (this == object)
return true;
return super.equals(object) &&
getValue().equals(((ImageValue)object).getValue());
}
public int hashCode() {
return super.hashCode() ^ getValue().hashCode();
}
/**
* Returns a pointer to the search path for images. This search path will be
* set according to image resolution rules, which depend on whether the
* image tag came from a fragment. This method may return NULL if the image
* tag is not in a fragment.
*
* @exclude from published api.
*/
private List getImageSearchPath() {
return mpoImageSearchPath;
}
public boolean getIsNull() {
if (isPropertySpecified(XFA.HREFTAG, true, 0)) {
String sSource = "";
sSource = getAttribute(XFA.HREFTAG).toString();
if (!StringUtils.isEmpty(sSource)) {
Attribute attr = getXsiNilAttribute();
// check the xsi:nil attr
if (attr == null || !attr.getAttrValue().equals(STRS.TRUE))
return false;
}
}
return super.getIsNull();
}
public ScriptTable getScriptTable() {
return ImageScript.getScriptTable();
}
public String getValue() {
String sSource = "";
boolean bImageIsALink = isPropertySpecified(XFA.HREFTAG, true, 0);
if (bImageIsALink) {
sSource = getAttribute(XFA.HREFTAG).toString();
}
if (!bImageIsALink || StringUtils.isEmpty(sSource)) {
sSource = getStrValue();
}
return sSource;
}
/**
* @see Element#setAttribute(Attribute, int)
* @exclude
*/
public void setAttribute(Attribute oAttr, int eTag) {
super.setAttribute(oAttr, eTag);
if (eTag == XFA.HREFTAG && oAttr != null) {
// Clear the value of the image element. If this image
// was embedded, this will clear the base64 encoded data
// if it exists.
setStrValue("", true, false);
}
}
/**
* Set the value for this image
* This function will set the value of the #text child to the base64 encoded
* value of the image. It will also set the contentType and tranferEncoding attributes
* on the image element
*
* @param pImageBuffer - the raw image value.
* @param sContentType - the content type of the image
*/
public void setValue(byte[] pImageBuffer, String sContentType) {
// clear href attribute
setAttribute(new StringAttr(XFA.HREF, ""), XFA.HREFTAG);
// set content type
setAttribute(new StringAttr(XFA.CONTENTTYPE, sContentType), XFA.CONTENTTYPETAG);
// set transferencoding
setAttribute(EnumAttr.TRANSFER_BASE64, XFA.TRANSFERENCODINGTAG);
// set the value of the image element
String sBase64 = Base64.encode(pImageBuffer, true);
setStrValue(sBase64, true, false);
}
/**
* Set the base64 encoded value for this image
* This function will set the value of the #text child to the base64 encoded
* value of the image. It will also set the contentType and tranferEncoding attributes
* on the image element
*
* @param sB64 - base 64 encoded data
* @param sContentType - the content type of the image
*/
public void setValue(String sB64, String sContentType/* ="" */) {
// clear href attribute
setAttribute(new StringAttr(XFA.HREF, ""), XFA.HREFTAG);
// set content type
if (!StringUtils.isEmpty(sContentType))
setAttribute(new StringAttr(XFA.CONTENTTYPE, sContentType), XFA.CONTENTTYPETAG);
// set transferencoding
setAttribute(EnumAttr.TRANSFER_BASE64, XFA.TRANSFERENCODINGTAG);
// set the value of the image element
setStrValue(sB64, true, false);
}
/**
* Return the value of the content as a string
* @return the string representation of the value.
*/
public String toString() {
return getValue();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy