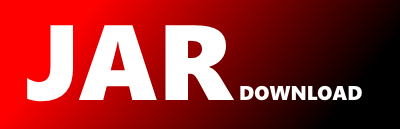
com.adobe.xfa.form.SignDispatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.form;
import com.adobe.xfa.Element;
import com.adobe.xfa.EventManager;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.ResId;
/**
* Manages the node
*
* @exclude from public api.
*/
class SignDispatcher extends com.adobe.xfa.Dispatcher {
//private Element mSignDataElement;
public final static class SignOpResult {
public final static int kSignOpResult_NoSignature = 0;
public final static int kSignOpResult_Successed = 1;
public final static int kSignOpResult_Failed = 2;
}
SignDispatcher(Element signContextNode,
Element signDataElement,
String sEventContext,
int nEventID,
EventManager poEventManager) {
super(signContextNode, sEventContext, nEventID, poEventManager);
//mSignDataElement = signDataElement;
}
public void dispatch() {
throw new ExFull(ResId.UNSUPPORTED_OPERATION, "SignDispatcher#dispatch");
// Node poNodeImpl = getActionContextNode();
// if (poNodeImpl == null)
// return;
//
// SignaturePseudoModel pSignatureModel = getSignatureModel();
// if (pSignatureModel == null)
// return;
//
// switch(getOperation()) {
// case EnumAttr.SIGNOPERATION_CLEAR:
// clear(true);
// break;
// case EnumAttr.SIGNOPERATION_VERIFY:
// verify(true);
// break;
// case EnumAttr.SIGNOPERATION_SIGN:
// sign(true);
// break;
// }
}
// public int /* SignOpResult */ clear(boolean bUI /* = true */) {
// SignaturePseudoModel pSignatureModel = getSignatureModel();
// if (pSignatureModel == null)
// return SignOpResult.kSignOpResult_Failed;
//
// String sTarget = getTarget();
// DSigData oSignature = pSignatureModel.getSignature(sTarget);
//
// // check permissions
// if (! pSignatureModel.clearPermsCheck(oSignature)) {
// MsgFormatPos message = new MsgFormatPos(ResId.PermissionsViolationExceptionMethod);
// message.format("clear");
// throw new ExFull(message);
// }
//
// if (oSignature.isNull())
// return SignOpResult.kSignOpResult_NoSignature;
//
// if (pSignatureModel.clear(oSignature, bUI))
// return SignOpResult.kSignOpResult_Successed;
//
// return SignOpResult.kSignOpResult_Failed;
// }
//
// public int /* SignOpResult */ verify(boolean bUI /* = true */) {
// SignaturePseudoModel pSignatureModel = getSignatureModel();
// if (pSignatureModel == null)
// return SignOpResult.kSignOpResult_Failed;
//
// String sTarget = getTarget();
// DSigData oSignature = pSignatureModel.getSignature(sTarget);
//
// if (oSignature.isNull())
// return SignOpResult.kSignOpResult_NoSignature;
//
// PKI_Base_SeedValues pSeedValues = new PKI_Base_SeedValues;
//
// DomSignatureInfo oSigInfo = getSigInfo(pSeedValues, bUI);
//
// if (pSignatureModel.verify(oSignature, oSigInfo))
// return SignOpResult.kSignOpResult_Successed;
//
// return SignOpResult.kSignOpResult_Failed;
// }
//
// public XFADSigData sign(boolean bUI /* = true */) {
// NodeList oDataNodes;
// getDataNodesFromManifest(oDataNodes);
//
// return sign(oDataNodes, bUI);
// }
//
// public DSigData sign(NodeList oDataNodes, boolean bUI /* = true */) {
// SignaturePseudoModel pSignatureModel = getSignatureModel();
// if (pSignatureModel == null)
// return DSigData();
//
// String sRef = getRef();
//
// // check permissions
// if (! pSignatureModel.signPermsCheck(sRef)) {
// MsgFormatPos message = new MsgFormatPos(ResId.PermissionsViolationExceptionMethod);
// message.format("sign");
// throw new ExFull(message);
// }
//
// PKI_Base_SeedValues oSeedValues;
//
// DomSignatureInfo oSigInfo = getSigInfo(oSeedValues, bUI);
//
// // get $form
// XFAFormModelImpl* poFormModelImpl = NULL;
//
// XFANodeImpl* poActionContextNode = getActionContextNode();
// assert(poActionContextNode);
// if (poActionContextNode)
// {
// poFormModelImpl = (XFAFormModelImpl*)poActionContextNode->getModelImpl();
// }
//
// assert(poFormModelImpl);
// if (poFormModelImpl == null)
// return DSigData();
//
//
// // set up preSign/postSign events
// jfString sPreSign = XFAEnum::getString(XFAEnum::ACTIVITY_PRESIGN);
// jfString sPostSign = XFAEnum::getString(XFAEnum::ACTIVITY_POSTSIGN);
//
// // dispatch preSign event
// if (poFormModelImpl && poActionContextNode && sPreSign.Length())
// poFormModelImpl->eventOccurred(sPreSign, poActionContextNode);
//
// // sign
// XFADSigData oReturnVal;
// XFAEventManager* poEventManager = getEventManager();
// if (poEventManager && ! poEventManager->cancelAction(aXFASTRS_SIGN))
// {
// try
// {
// oReturnVal = pSignatureModel->sign(oDataNodes, sRef, oSigInfo);
// }
// catch (jfExFull& oError)
// {
// // dispatch postSign event even if there is an error
// if (poFormModelImpl && poActionContextNode && sPostSign.Length())
// poFormModelImpl->eventOccurred(sPostSign, poActionContextNode);
//
// throw(oError);
// }
// }
//
// // dispatch postSign event
// if (poFormModelImpl && poActionContextNode && sPostSign.Length())
// poFormModelImpl->eventOccurred(sPostSign, poActionContextNode);
//
// return oReturnVal;
// }
//
// public int /* SignOpResult */ hide(boolean bHide) {
// SignaturePseudoModel pSignatureModel = getSignatureModel();
// if (pSignatureModel == null)
// return SignOpResult.kSignOpResult_Failed;
//
// String sTarget = getTarget();
// DSigData oSignature = pSignatureModel.getSignature(sTarget);
//
// if (oSignature.isNull())
// return SignOpResult.kSignOpResult_NoSignature;
//
// Node poNode = (Node)oSignature;
//
// poNode.isTransient(bHide);
//
// return SignOpResult.kSignOpResult_Successed;
// }
//
// public SignaturePseudoModel getSignatureModel() {
// Node pSignData = moSignDataElement;
//
// if (pSignData == null || pSignData.getModel() == null || !pSignData.isSameClass(XFA.SIGNDATATAG))
// return null; // invalid node
//
// AppModel pAppModel = ((Element)pSignData).getAppModel();
//
// SignaturePseudoModel pSignatureModel =
// (SignaturePseudoModel)pAppModel.lookupPseudoModel("$signature");
//
// if (pSignatureModel == null)
// return null; // no signature support
//
// return pSignatureModel;
// }
//
// public boolean getDataNodesFromManifest(NodeList oDataNodes) {
// Element pSignData = moSignDataElement;
// assert(pSignData != null);
//
// if (pSignData == null || pSignData.getModel() == null)
// return false;
//
// Node poManifest = pSignData.getElement(XFA.MANIFESTTAG, true, 0, false, false);
//
// // no manifest
// if (poManifest == null)
// return false;
//
// // we only support include
// int eAction = poManifest.getEnum(XFA.ACTION).getInt();
// if (eAction != EnumAttr.MANIFESTACTION_INCLUDE) {
// //"%1 is an unsupported operation for the %2 object"
// MsgFormatPos oEx = new MsgFormatPos(ResId.UnsupportedOperationException);
// oEx.format(XFA.ACTION + "=\"" + EnumAttr.getString(eAction) + '"');
// oEx.format(XFA.MANIFEST);
// throw new ExFull(oEx);
// }
//
// for (Node poRef = poManifest.getFirstChild(); poRef != null; poRef = poRef.getNextSibling()) {
// if (poRef.isSameClass(XFA.REFTAG)) {
// String sSom = ((GenericTextContainer)poRef).getValue(); // JavaPort: correct class???
//
// NodeList oSomResult = poRef.getAppModel().resolveNodes(sSom);
//
// if (oSomResult == null || oSomResult.length() == 0) {
// MsgFormatPos oEx = new MsgFormatPos(ResId.EmptySOMResult);
// oEx.format(sSom);
// throw new ExFull(oEx);
// }
//
// int nSomResult = oSomResult.length();
//
// for (int j = 0; j < nSomResult; j++) {
// Node oTargetNode = (Node)oSomResult.item(j);
//
// // transient or some other node.
// if (oTargetNode.isNull() ||
// oTargetNode.getTransientFlag() ||
// oTargetNode.getDefaultFlag() ||
// oTargetNode.getFragmentFlag() ||
// oTargetNode.getModel() instanceof FormModel) {
// MsgFormatPos oEx = new MsgFormatPos(ResId.TransientNodeSignatureException);
// oEx.format("signField");
// throw new ExFull(oEx);
// }
// // accept all other nodes
// oDataNodes.append(oTargetNode);
// }
// }
// }
//
// return true;
// }
//
// public DomSignatureInfo getSigInfo(PKI_Base_SeedValues pSeedValues, boolean bUI /* = true */) {
// DomSignatureInfo oSigInfo;
//
// Element pSignData = moSignDataElement;
// assert(pSignData != null);
//
// if (pSignData == null || pSignData.getModel() == null)
// return DomSignatureInfo();
//
// oSigInfo.sXMLID = getTarget();
//
// // get ui flag
// oSigInfo.bUI = bUI;
//
// // set the seed value object
// oSigInfo.poSeedValue = pSeedValues;
//
// // get seed values
// Node pFilter = pSignData.getElement(XFA.FILTERTAG, true, 0, false, false);
// if (pFilter != null) {
// SignaturePseudoModel.getSeedValues(pFilter, pSeedValues);
// }
//
// return oSigInfo;
// }
//
// public int getOperation() {
// if (moSignDataElement != null)
// return moSignDataElement.getEnum(XFA.OPERATIONTAG);
//
// return EnumAttr.UNDEFINED;
// }
//
// public String getTarget() {
// if (moSignDataElement != null)
// return moSignDataElement.getAttribute(XFA.TARGETTAG).toString();
//
// return "";
// }
//
// public String getRef() {
// if (moSignDataElement != null)
// return moSignDataElement.getAttribute(XFA.REFTAG).toString();
//
// return "";
// }
public String getDispatcherType() {
return "signData";
}
// public String getParameter(int nParam) {
// /*if (nParam == 0)
// return getContentType();
// else if (nParam == 1)
// return getScript();
// */
// return "";
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy