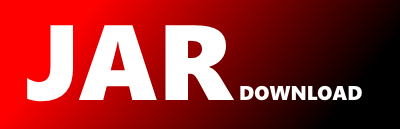
com.adobe.xfa.formcalc.BuiltinDate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.formcalc;
import com.adobe.xfa.ut.ISODate;
import com.adobe.xfa.ut.ISOTime;
import com.adobe.xfa.ut.LcData;
import com.adobe.xfa.ut.LcDate;
import com.adobe.xfa.ut.LcTime;
/**
*
* This class defines static methods to implement
* the FormCalc date and time calculations.
*
* D A T E F U N C T I O N S
* date, date2num, isodate2num, num2date, datefmt, localdatefmt.
*
* @author Mike P. Tardif
*
* @exclude from published api.
*/
final class BuiltinDate {
/*
* Disallow instances of this class.
*/
private BuiltinDate() {
}
/*
* Date
* This function returns the number of days from the epoch
* for today's date.
*/
static void Date(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.maxArgs(nArgs, 0);
//
// get the number of days for today's date.
//
LcDate date = new LcDate("", LcDate.DEFAULT_CENTURY_SPLIT);
oRetSym = new CalcSymbol(date.getDays());
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Date2Num
* This function returns the number of days from the epoch
* for a given date string.
*
*/
static void Date2Num(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 3);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// retrieve and normalize arguments.
//
String s = oParser.getString(oArgSym[0]);
String f = (nArgs > 1) ? oParser.getString(oArgSym[1])
: LcDate.DEFAULT_DATE_FMT;
String l = (nArgs > 2) ? oParser.getString(oArgSym[2])
: oParser.msLocaleName;
//
// compute the number of days for the given date string.
//
LcDate date = new LcDate(s, f, l, LcDate.DEFAULT_CENTURY_SPLIT);
oRetSym = new CalcSymbol(date.getDays());
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Num2Date
* This function returns a date string given the number of days
* from the epoch.
*
*/
static void Num2Date(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 3);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// retrieve and normalize arguments.
//
int nDays = (int) oParser.getNumeric(oArgSym[0]);
String f = (nArgs > 1) ? oParser.getString(oArgSym[1])
: LcDate.DEFAULT_DATE_FMT;
String l = (nArgs > 2) ? oParser.getString(oArgSym[2])
: oParser.msLocaleName;
//
// compute the date string for the given number of days.
//
LcDate date = new LcDate(nDays, l, LcDate.DEFAULT_CENTURY_SPLIT);
oRetSym = new CalcSymbol(date.format(f));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* DateFmt
* This function returns a format date string,
* given a date format style.
*
*/
static void DateFmt(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 0);
Builtins.maxArgs(nArgs, 2);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// retrieve and normalize arguments.
//
int n = (nArgs > 0) ? (int) oParser.getNumeric(oArgSym[0]) : 0;
String l = (nArgs > 1) ? oParser.getString(oArgSym[1])
: oParser.msLocaleName;
//
// get the locale's date format style.
//
oRetSym = new CalcSymbol(LcDate.getDateFormat(n, l));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* DateTimeFmt
* This function returns a format datetime string,
* given a datetime format style.
*
*/
static void DateTimeFmt(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 0);
Builtins.maxArgs(nArgs, 2);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// retrieve and normalize arguments.
//
int n = (nArgs > 0) ? (int) oParser.getNumeric(oArgSym[0]) : 0;
String l = (nArgs > 1) ? oParser.getString(oArgSym[1])
: oParser.msLocaleName;
//
// get the locale's date format style.
//
LcData data = new LcData(l);
oRetSym = new CalcSymbol(data.getDateTimeFormat(n, 1));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* LocalDateFmt
* This function returns a localized format date string,
* given a date format style.
* Note: this is implementable only on a Java platform. Pity!
*
*/
static void LocalDateFmt(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 0);
Builtins.maxArgs(nArgs, 2);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// retrieve and normalize arguments.
//
int n = (nArgs > 0) ? (int) oParser.getNumeric(oArgSym[0]) : 0;
String l = (nArgs > 1) ? oParser.getString(oArgSym[1])
: oParser.msLocaleName;
//
// get the locale's localized date format style.
//
oRetSym = new CalcSymbol(LcDate.getLocalDateFormat(n, l));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* IsoDate2Num
* This function returns the number of days from the epoch
* for a given ISO date string.
*
*/
static void IsoDate2Num(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 1);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// compute the number of days for the given iso date string.
//
String s = oParser.getString(oArgSym[0]);
ISODate isoDate = new ISODate(s, "");
oRetSym = new CalcSymbol(isoDate.getDays());
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
////////////////////////////////////////////////////////////////////////////////
//
// T I M E F U N C T I O N S
// time, time2num, isotime2num, num2time, num2gmtime, timefmt,
// localtimefmt.
//
////////////////////////////////////////////////////////////////////////////////
static void Time(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.maxArgs(nArgs, 0);
//
// get the number of days for today's time.
//
LcTime time = new LcTime("");
oRetSym = new CalcSymbol(time.getMillis());
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
static void Time2Num(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 3);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// retrieve and normalize arguments.
//
String s = oParser.getString(oArgSym[0]);
String f = (nArgs > 1) ? oParser.getString(oArgSym[1])
: LcTime.DEFAULT_TIME_FMT;
String l = (nArgs > 2) ? oParser.getString(oArgSym[2])
: oParser.msLocaleName;
//
// compute the number of milliseconds for the given time string.
//
LcTime time = new LcTime(s, f, l);
oRetSym = new CalcSymbol(time.getMillis());
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
static void IsoTime2Num(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 1);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// compute the number of milliseconds for the given iso time string.
//
String s = oParser.getString(oArgSym[0]);
ISOTime isoTime = new ISOTime(s, "");
oRetSym = new CalcSymbol(isoTime.getMillis());
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
static void Num2Time(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 3);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// retrieve and normalize arguments.
//
int nMillis = (int) oParser.getNumeric(oArgSym[0]);
String f = (nArgs > 1) ? oParser.getString(oArgSym[1])
: LcTime.DEFAULT_TIME_FMT;
String l = (nArgs > 2) ? oParser.getString(oArgSym[2])
: oParser.msLocaleName;
//
// compute the time string for the given number of milliseconds
// adjusted for local time.
//
LcTime time = new LcTime(nMillis, l);
time.setLocalTime();
oRetSym = new CalcSymbol(time.format(f));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
static void Num2GmTime(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 3);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// retrieve and normalize arguments.
//
int nMillis = (int) oParser.getNumeric(oArgSym[0]);
String f = (nArgs > 1) ? oParser.getString(oArgSym[1])
: LcTime.DEFAULT_TIME_FMT;
String l = (nArgs > 2) ? oParser.getString(oArgSym[2])
: oParser.msLocaleName;
//
// compute the time string for the given number of milliseconds.
//
LcTime time = new LcTime(nMillis, l);
oRetSym = new CalcSymbol(time.format(f));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
static void TimeFmt(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 0);
Builtins.maxArgs(nArgs, 2);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// retrieve and normalize arguments.
//
int n = (nArgs > 0) ? (int) oParser.getNumeric(oArgSym[0]) : 0;
String l = (nArgs > 1) ? oParser.getString(oArgSym[1])
: oParser.msLocaleName;
//
// get the locale's time format style.
//
oRetSym = new CalcSymbol(LcTime.getTimeFormat(n, l));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
static void LocalTimeFmt(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 0);
Builtins.maxArgs(nArgs, 2);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// retrieve and normalize arguments.
//
int n = (nArgs > 0) ? (int) oParser.getNumeric(oArgSym[0]) : 0;
String l = (nArgs > 1) ? oParser.getString(oArgSym[1])
: oParser.msLocaleName;
//
// get the locale's localized time format style.
//
oRetSym = new CalcSymbol(LcTime.getLocalTimeFormat(n, l));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy