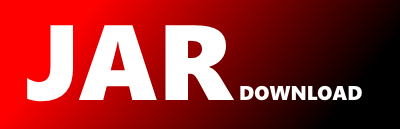
com.adobe.xfa.formcalc.BuiltinScientific Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.formcalc;
/**
* This class defines static methods to implement
* the FormCalc scientific calculations.
*
* S C I E N T I F I C F U N C T I O N S
* acos, asin, atan, cos, deg2rad, exp, log, pi, pow, rad2deg,
* sin, sqrt, tan.
*
* @author Mike P. Tardif
*
* @exclude from published api.
*/
final class BuiltinScientific {
/*
* Disallow instances of this class.
*/
private BuiltinScientific() {
}
/*
* Acos
* This function returns the arc cosine value of a given number.
*/
static void Acos(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 1);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// promote the arg to a double, and take its arc cosine.
//
double nVal = oParser.getNumeric(oArgSym[0]);
if (-1. > nVal || nVal > 1.)
throw new CalcException();
oRetSym = new CalcSymbol(Math.acos(nVal));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Asin
* This function returns the arc sine value of a given number.
*/
static void Asin(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 1);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// promote the arg to a double, and take its arc sine.
//
double nVal = oParser.getNumeric(oArgSym[0]);
if (-1. > nVal || nVal > 1.)
throw new CalcException();
oRetSym = new CalcSymbol(Math.asin(nVal));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Atan
* This function returns the arc tangent value of a given number.
*/
static void Atan(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 1);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// promote the arg to a double, and take its arc tangent.
//
double nVal = oParser.getNumeric(oArgSym[0]);
oRetSym = new CalcSymbol(Math.atan(nVal));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Atan2
* This function returns the arc tangent value of the given numbers.
*/
static void Atan2(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 2);
Builtins.maxArgs(nArgs, 2);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// promote the args to doubles, and take their arc tangent.
//
double nVal1 = oParser.getNumeric(oArgSym[0]);
double nVal2 = oParser.getNumeric(oArgSym[1]);
oRetSym = new CalcSymbol(Math.atan2(nVal1, nVal2));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Cos
* This function returns the cosine value of a given number.
*/
static void Cos(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 1);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// promote the arg to a double, and take its cosine.
//
double nVal = oParser.getNumeric(oArgSym[0]);
oRetSym = new CalcSymbol(Math.cos(nVal));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Deg2Rad
* This function returns the radian value of a given number.
*/
static void Deg2Rad(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 1);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// promote the arg to a double, and take its radian.
//
double nVal = oParser.getNumeric(oArgSym[0]);
oRetSym = new CalcSymbol(nVal * Math.PI / 180.);
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Exp
* This function returns the value of e raised to a given number.
*/
static void Exp(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 1);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// promote the arg to a double, and take its exponential.
//
double nVal = oParser.getNumeric(oArgSym[0]);
oRetSym = new CalcSymbol(Math.exp(nVal));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Log
* This function returns the natural logarithm value of a given number.
*/
static void Log(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 1);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// promote the arg to a double, and take its logarithm.
//
double nVal = oParser.getNumeric(oArgSym[0]);
if (nVal <= 0.)
throw new CalcException();
oRetSym = new CalcSymbol(Math.log(nVal));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Pi
* This function returns the value of pi.
*/
static void Pi(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.maxArgs(nArgs, 0);
//
// make pi.
//
oRetSym = new CalcSymbol(Math.PI);
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Pow
* This function returns the value of a number raised to a given power.
*/
static void Pow(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 2);
Builtins.maxArgs(nArgs, 2);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// promote the args to doubles, and take the exponential.
//
double nVal1 = oParser.getNumeric(oArgSym[0]);
double nVal2 = oParser.getNumeric(oArgSym[1]);
if (nVal2 == 0.)
oRetSym = new CalcSymbol(1.);
else
oRetSym = new CalcSymbol(Math.pow(nVal1, nVal2));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Rad2Deg
* This function returns the degree value of a given number.
*/
static void Rad2Deg(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 1);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// promote the arg to a double, and take its degree.
//
double nVal = oParser.getNumeric(oArgSym[0]);
oRetSym = new CalcSymbol(nVal * 180. / Math.PI);
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Sin
* This function returns the sine value of a given number.
*/
static void Sin(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 1);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// promote the arg to a double, and take its sine.
//
double nVal = oParser.getNumeric(oArgSym[0]);
oRetSym = new CalcSymbol(Math.sin(nVal));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Sqrt
* This function returns the square root value of a given number.
*/
static void Sqrt(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 1);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// promote the arg to a double, and take its square root.
//
double nVal = oParser.getNumeric(oArgSym[0]);
if (nVal < 0.)
throw new CalcException();
oRetSym = new CalcSymbol(Math.sqrt(nVal));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
/*
* Tan
* This function returns the tangent value of a given number.
*/
static void Tan(CalcParser oParser, CalcSymbol[] oArgSym) {
final int nArgs = oArgSym.length;
CalcSymbol oRetSym = null;
try {
//
// check the number of args vs the number required.
//
Builtins.minArgs(nArgs, 1);
Builtins.maxArgs(nArgs, 1);
//
// check for error-valued, return-valued and null-valued args.
//
Builtins.limitExceptionArgs(oArgSym);
Builtins.limitNullArgs(oParser, nArgs, oArgSym);
//
// promote the arg to a double, and take its tangent.
//
double nVal = oParser.getNumeric(oArgSym[0]);
oRetSym = new CalcSymbol(Math.tan(nVal));
} catch (CalcException e) {
oRetSym = e.getSymbol();
if (oRetSym.getType() != CalcSymbol.TypeNull)
oParser.mbInThrow = true;
}
//
// push the result on the stack.
//
oParser.mStack.push(oRetSym);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy