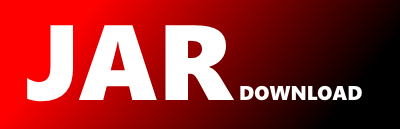
com.adobe.xfa.gfx.GFXMapping Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
package com.adobe.xfa.gfx;
/**
* This class represents one character/glyph mapping.
*
* Logically, a mapping is represented by a set of (possibly disjoint)
* character indexes and a set of (possibly disjoint) glyph indexes. In
* other words, a mapping can be thought of as containing a pair of
* arrays. The implementation may choose a more efficient
* representation for common cases.
*
* @exclude from published api.
*/
public class GFXMapping {
private static class IndexSet {
private int mnStart;
private int mnLength;
private int[] mpoIndexes;
IndexSet () {
}
IndexSet (IndexSet oSource) {
copyFrom (oSource);
}
IndexSet (int nIndex, int nLength) {
mnStart = nIndex;
mnLength = nLength;
}
void add (int nIndex) {
if (mpoIndexes == null) {
int nStart = mnStart;
int nLimit = nStart + mnLength;
if (nLimit == nStart) {
mnStart = nIndex;
mnLength = 1;
} else if ((nIndex + 1) == nStart) {
mnStart--;
} else if (nIndex == nLimit) {
mnLength++;
} else if ((nIndex < nStart) || (nIndex >= nLimit)) {
forceArray();
}
}
if (mpoIndexes != null) {
int currentLength = mpoIndexes.length;
if (mnLength >= currentLength) {
int[] newIndexes = new int [currentLength * 2];
for (int i = 0; i < mnLength; i++) {
newIndexes[i] = mpoIndexes[i];
}
mpoIndexes = newIndexes;
}
mpoIndexes[mnLength] = nIndex;
mnLength++;
}
}
int getCount () {
return mnLength;
}
int get (int nIndex) {
return (mpoIndexes == null) ? (mnStart + nIndex) : (mpoIndexes)[nIndex];
}
int getLowest () {
return (mpoIndexes == null) ? mnStart : searchLowest();
}
int getHighest () {
return (mpoIndexes == null) ? (mnStart + mnLength - 1) : searchHighest();
}
void copyFrom (IndexSet oSource) {
if (this != oSource) {
mnLength = oSource.mnLength;
if (oSource.mpoIndexes == null) {
mpoIndexes = null;
mnStart = oSource.mnStart;
} else {
if ((mpoIndexes == null) || (mpoIndexes.length < oSource.mnLength)) {
mpoIndexes = new int [oSource.mpoIndexes.length];
}
for (int i = 0; i < oSource.mnLength; i++) {
mpoIndexes[i] = oSource.mpoIndexes[i];
}
}
}
}
private int searchLowest () {
assert (mpoIndexes != null);
int nReturn = Integer.MAX_VALUE;
for (int i = 0; i < mnLength; i++) {
int nIndex = mpoIndexes[i];
if (nIndex < nReturn) {
nReturn = nIndex;
}
}
return nReturn;
}
private int searchHighest () {
assert (mpoIndexes != null);
int nReturn = 0;
for (int i = 0; i < mnLength; i++) {
int nIndex = mpoIndexes[i];
if (nIndex > nReturn) {
nReturn = nIndex;
}
}
return nReturn;
}
private void forceArray () {
assert (mpoIndexes == null);
int size = 4;
while (size < mnLength) {
size = size * 2;
}
mpoIndexes = new int [size];
for (int i = 0; i < mnLength; i++) {
mpoIndexes[i] = mnStart + i;
}
}
}
private final IndexSet moChars;
private final IndexSet moGlyphs;
/**
* Default constructor.
*
* The mapping is initialized to an invalid state. The set of
* characters and the set of glyphs are both empty. Adding such a
* mapping to a mapping list is an error.
*
*/
public GFXMapping () {
moChars = new IndexSet();
moGlyphs = new IndexSet();
}
/**
* Copy constructor.
* The mapping is created with the same content as the given mapping.
* @param oSource - Source mapping object to copy.
*/
public GFXMapping (GFXMapping oSource) {
moChars = new IndexSet (oSource.moChars);
moGlyphs = new IndexSet (oSource.moGlyphs);
}
/**
* Constructor that initializes the mapping to represent a contiguous
* run of characters and a contiguous run of glyphs.
*
* The client specifies the mapping in terms of four parameters
* identifying both a sequential run of characters and a sequential run
* of glyphs. Given the parameter names listed in the method
* declaration, the mapping is interpreted as the nCharLength characters
* in the Unicode text, starting at index nCharIndex, map to the
* nGlyphLength glyphs in the rendering starting at nGlyphIndex.
* @param nCharIndex - Starting character index of the mapping.
* @param nGlyphIndex - Starting glyph index of the mapping.
* @param nCharLength - Number of consecutive Unicode characters in the
* mapping.
* @param nGlyphLength - Number of consecutive glyphs in the mapping.
*/
public GFXMapping (int nCharIndex, int nGlyphIndex, int nCharLength, int nGlyphLength) {
moChars = new IndexSet (nCharIndex, nCharLength);
moGlyphs = new IndexSet (nGlyphIndex, nGlyphLength);
}
public GFXMapping (int nCharIndex, int nGlyphIndex, int nCharLength) {
this (nCharIndex, nGlyphIndex, nCharLength, 1);
}
public GFXMapping (int nCharIndex, int nGlyphIndex) {
this (nCharIndex, nGlyphIndex, 1, 1);
}
/**
* Add another character index to the set of characters represented by
* this mapping.
*
* This method is typically called only if the mapping represents a set
* of non-contiguous characters in the Unicode text.
* @param nCharIndex - Character index to add to the mapping.
*/
public void addCharIndex (int nCharIndex) {
moChars.add (nCharIndex);
}
/**
* Return the number of character indexes currently in the mapping.
* @return Number of character indexes currently in the mapping.
*/
public int getCharCount () {
return moChars.getCount();
}
/**
* Extract one character index from the mapping.
*
* The character indexes themselves are indexed from zero up to one less
* than the value returned by GetCharCount().
* @param nIndex - Index of the desired character index.
* @return Character index value.
*/
public int getCharIndex (int nIndex) {
return moChars.get (nIndex);
}
/**
* Return the lowest character index from the mapping.
*
* The lowest character index may not be the first in the list of
* character index, depending on the order added. It is an error to
* call this method if the mapping contains no character index values.
* @return Lowest character index value.
*/
public int getLowestCharIndex () {
return moChars.getLowest();
}
/**
* Return the highest character index from the mapping.
*
* The highest character index may not be the last in the list of
* character index, depending on the order added. It is an error to
* call this method if the mapping contains no character index values.
* @return Highest character index value.
*/
public int getHighestCharIndex () {
return moChars.getHighest();
}
/**
* Add another glyph index to the set of glyphs represented by this
* mapping.
*
* This method is typically called only if the mapping represents a set
* of non-contiguous glyphs in the Unicode text.
* @param nGlyphIndex - Glyphacter index to add to the mapping.
*/
public void addGlyphIndex (int nGlyphIndex) {
moGlyphs.add (nGlyphIndex);
}
/**
* Return the number of glyph indexes currently in the mapping.
* @return Number of glyph indexes currently in the mapping.
*/
public int getGlyphCount () {
return moGlyphs.getCount();
}
/**
* Extract one glyph index from the mapping.
*
* The glyph indexes themselves are indexed from zero up to one less
* than the value returned by GetGlyphCount().
* @param nIndex - Index of the desired glyph index.
* @return Glyphacter index value.
*/
public int getGlyphIndex (int nIndex) {
return moGlyphs.get (nIndex);
}
/**
* Return the lowest glyph index from the mapping.
*
* The lowest glyph index may not be the first in the list of glyph
* index, depending on the order added. It is an error to call this
* method if the mapping contains no glyph index values.
* @return Lowest glyph index value.
*/
public int getLowestGlyphIndex () {
return moGlyphs.getLowest();
}
/**
* Return the highest glyph index from the mapping.
*
* The highest glyph index may not be the last in the list of glyph
* index, depending on the order added. It is an error to call this
* method if the mapping contains no glyph index values.
* @return Highest glyph index value.
*/
public int getHighestGlyphIndex () {
return moGlyphs.getHighest();
}
/**
* Assignment operator.
*
* This mapping's content is replaced with a copy of the content from
* the given mapping.
* @param oSource - Source mapping object to copy.
*/
public void copyFrom (GFXMapping oSource) {
if (this != oSource) {
moChars.copyFrom (oSource.moChars);
moGlyphs.copyFrom (oSource.moGlyphs);
}
}
int getCount (boolean bGlyph) {
return bGlyph ? getGlyphCount() : getCharCount();
}
int getIndex (boolean bGlyph, int nIndex) {
return bGlyph ? getGlyphIndex (nIndex) : getCharIndex (nIndex);
}
int getLowestIndex (boolean bGlyph) {
return bGlyph ? getLowestGlyphIndex() : getLowestCharIndex();
}
int getHighestIndex (boolean bGlyph) {
return bGlyph ? getHighestGlyphIndex() : getHighestCharIndex();
}
}