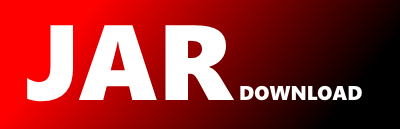
com.adobe.xfa.pmp.adobepdf417pmp.PDF417ImageBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*************************************************************************
*
* File: PDF417ImageBuilder.java
*
**************************************************************************
*
* ADOBE CONFIDENTIAL
* ___________________
*
* Copyright 2011 Adobe Systems Incorporated
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains
* the property of Adobe Systems Incorporated and its suppliers,
* if any. The intellectual and technical concepts contained
* herein are proprietary to Adobe Systems Incorporated and its
* suppliers and are protected by trade secret or copyright law.
* Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained
* from Adobe Systems Incorporated.
**************************************************************************/
package com.adobe.xfa.pmp.adobepdf417pmp;
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
/**
* Ported from PDF417ImageBuilder.cpp
*/
class PDF417ImageBuilder {
private static Color getColor(int color) {
if (color == 0)
return Color.BLACK;
return Color.WHITE;
}
// ////////////////////////////////////////////////////////////////////
/**
* Returns a image build from the X-sequence that is centered in a specific
* sized image.
*/
// ////////////////////////////////////////////////////////////////////
public static BufferedImage buildCentered(String xseq, int x, int y,
int xShave, int yShave, int dataCols, int dataRows, int imageCols,
int imageRows) {
// Calculate the offset to center the symbol in the image
int offsetCols = (imageCols - (((dataCols * 17) + 17 + 17 + 17 + 18 + 4) * x)) / 2;
int offsetRows = (imageRows - (dataRows * y) - (4 * x)) / 2;
// Create the image
BufferedImage pImage = new BufferedImage(imageCols, imageRows,
BufferedImage.TYPE_BYTE_GRAY);
Graphics2D graphicsBarcodeImage = pImage.createGraphics();
graphicsBarcodeImage.setColor(Color.WHITE);
graphicsBarcodeImage.fillRect(0, 0, Integer.MAX_VALUE, Integer.MAX_VALUE);
graphicsBarcodeImage.clipRect(0, 0, imageCols - offsetCols - 2 * x,
imageRows - offsetRows - 2 * x);
// Convert the X-sequence to rectangles in the image.
int col = 2 * x + offsetCols;
int row = 2 * x + offsetRows;
int w;
int color = 0;
int size = xseq.length();
char c;
int idx;
for (idx = 0; idx < size; idx++) {
c = xseq.charAt(idx);
if (c != '\n') {
w = x * (c - 48);
Color currentColor = getColor(color);
graphicsBarcodeImage.setColor(currentColor);
graphicsBarcodeImage.fillRect(col + xShave, row + yShave, col
+ w - xShave, row + y - yShave);
col += w;
color ^= 0xff;
} else {
row = row + y;
col = 2 * x + offsetCols;
color = 0;
}
}
return (pImage);
}
// ////////////////////////////////////////////////////////////////////
/**
* Returns the image size in imageCols and imageRows
*/
// ////////////////////////////////////////////////////////////////////
/*private int[] getImageSize(String xseq, int x, int y) {
int[] imageSize = new int[2];
// Find the number of cols in the image
int idx = 0;
char c;
while ((c = xseq.charAt(idx++)) != '\n')
imageSize[0] += (c - 48);
imageSize[0] = imageSize[0] * x + 4 * x; // The 4*x is the quiet zone
// Find the number of rows in the image.
int size = xseq.length();
for (idx = 0; idx < size; idx++) {
c = xseq.charAt(idx);
if (c == '\n')
imageSize[1]++;
}
imageSize[1] = imageSize[1] * y + 4 * x; // The 4*x is the quiet zone
return imageSize;
}*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy