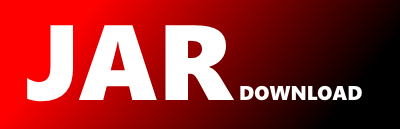
com.adobe.xfa.protocol.Protocol Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.protocol;
import java.io.InputStream;
import java.net.URLStreamHandler;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.adobe.xfa.ut.ExFull;
import com.adobe.xfa.ut.FindBugsSuppress;
/**
* A interface that all Internet protocol objects must implement.
*
* @author Mike Tardif
*/
public interface Protocol {
/**
* Describes an entry in a multipart MIME section in a multipart/form-data media type.
* Multipart MIME sections are described by a sequence of MultiPartDesc
* entries, and the value of each entry is interpreted by a eSectionDataOption
* as described by this enumeration.
*
* A multipart MIME section should contain either a
* SECTION_CONTENT_FILE
or a SECTION_CONTENT_VALUE
entry,
* but not both.
*
* A multipart MIME section is terminated by a a MultiPartDesc with eSectionDataOption of
* SECTION_END.
*
* @see MultiPartDesc#eSectionDataOption
*/
public enum SectionDataOption {
/**
* The value field contains the name of the original field
* that corresponds to this section (i.e., the name
* parameter of the Content-Disposition
header.
*/
SECTION_CONTENT_NAME,
/**
* The value field contains the Content-Type
of this section.
*/
SECTION_CONTENT_TYPE,
/**
* The value field contains the name of a file containing the data
* content to be sent.
* The file name will also be sent in the filename
* parameter of the Content-Disposition
header.
*/
SECTION_CONTENT_FILE,
/**
* The value field contains the data to be sent.
*/
SECTION_CONTENT_VALUE,
/**
* Delimits the end of a multipart MIME section.
* The value field is ignored.
*/
SECTION_END,
}
/**
* An enumeration of trust enforcement rules.
*/
public enum TrustType {
/**
* Trust url.
*/
URL,
/**
* Trust cross domain URLs.
*/
CROSS_DOMAIN,
/**
* Trust URLs coming from data.
*/
DATA_INJECTION,
/**
* Trust URLs coming from script.
*/
SCRIPT_INJECTION
}
/**
* Represents the result of a form data post.
*/
@FindBugsSuppress(code="EI2")
public class PostRsvp {
/**
* Instantiates a post response with the given values.
* @param nCode the response code.
* @param sType the response content type.
* @param data the response data.
* @param exception the response exception.
*/
public PostRsvp(int nCode, String sType, byte[] data, ExFull exception) {
this.nCode = nCode;
this.sType = sType;
this.data = data;
this.exception = exception;
}
/**
* The response status code.
*/
public final int nCode;
/**
* The response content type.
*/
public final String sType;
/**
* The response data.
*/
public final byte[] data;
/**
* The response exception if the post was unsuccessful.
*/
public final ExFull exception;
}
/**
* Represents multipart/form-data in a request.
*/
@FindBugsSuppress(code="EI2")
public class MultiPartDesc {
public MultiPartDesc(SectionDataOption eSectionDataOption, byte[] value) {
this.eSectionDataOption = eSectionDataOption;
this.value = value;
}
/**
* Describes an entry in a multipart MIME section.
*/
public final SectionDataOption eSectionDataOption;
/**
* Contains the content for this entry in a multipart MIME section.
* The contents of value are interpreted according to eSectionDataOption.
*/
public final byte[] value;
}
/**
* Represents simple POST data.
*/
@FindBugsSuppress(code="EI2")
public class SimplePostData {
/**
* Instantiates a simple post data object.
* @param data the data to be posted.
*/
public SimplePostData(byte[] data) {
this.data = data;
}
/**
* The MIME header fields, where each key is the field-name (without the terminating ":")
* and the corresponding value is the field-body-contents.
*/
public final Map headerMap = new HashMap(); // header strings.
/**
* The request data that will be posted (uploaded) to the server.
*/
public final byte[] data;
}
/**
* Gets the protocol's scheme.
* This method is required for all implementations of custom protocols
* and must return a non-null, non-empty value.
* @return this protocol's scheme.
*/
public String scheme();
/**
* Gets (downloads) the data designated by the given URL.
* @param sUrl the URL to be downloaded.
* @return an InputStream that contains the downloaded data.
*/
public InputStream get(String sUrl);
/**
* Puts (uploads) the given file's data to the given URL.
* The protocol designated must support uploading,
* e.g., http[s] PUT, or ftp[s] STOR.
* @param sFileName the file to be uploaded.
* @param sUrl the URL to upload the file to.
*/
public void put(String sFileName, String sUrl);
/**
* Puts (uploads) the given input stream's data to the given URL.
* The protocol designated must support uploading,
* e.g., http[s] PUT, or ftp[s] STOR.
* @param inputStream the input stream to be uploaded.
* @param sUrl the URL to upload the input stream to.
*/
public void put(InputStream inputStream, String sUrl);
/**
* Posts simple form data to the given URL.
* Only http[s] protocols support posting of data.
* @param data a description of the simple form data being posted to the URL.
* @param sUrl the URL to post the data to.
* @return a PostRsvp object containing the server's response.
* the server designed.
*/
public PostRsvp post(SimplePostData data, String sUrl);
/**
* Posts multipart form data to the given URL.
* Only http[s] protocols support posting of data.
* @param data a description of the multipart form data being posted to the URL.
* @param sUrl the URL to post the data to.
* @return a PostRsvp object containing the server's response.
*/
public PostRsvp post(List extends MultiPartDesc> data, String sUrl);
/**
* Gets the protocol's authentication handler.
* @return an AuthenticationHandler, or null
.
*/
public AuthenticationHandler getAuthenticationHandler();
/**
* Determines if the given URL can be trusted according to the given type of enforcement rule.
* @param sURL the URL to be trusted.
* @param eTrustType the type of trust enforcement needed.
* @param bThrow when true
, requests that a trust exception (ExFull)
* be thrown if the URL is not trusted.
* @return true
if trusted, otherwise false
.
*/
public boolean isTrusted(String sURL, TrustType eTrustType /* = TrustType.URL */, boolean bThrow /* = true */);
/**
* Gets the protocol's URL stream handler.
* This method is required for all implementations of custom protocols
* and must return a non-null value.
* @return an URL stream handler.
*/
public URLStreamHandler getURLStreamHandler();
}