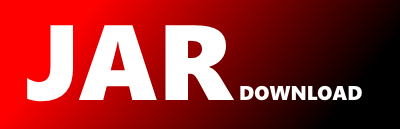
com.adobe.xfa.template.formatting.Occur Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.template.formatting;
import com.adobe.xfa.Attribute;
import com.adobe.xfa.Element;
import com.adobe.xfa.Int;
import com.adobe.xfa.Node;
import com.adobe.xfa.ProtoableNode;
import com.adobe.xfa.XFA;
/**
* An element that describes the constraints over the number of allowable
* instances for a subform or page
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public final class Occur extends ProtoableNode {
public Occur(Element parent, Node prevSibling) {
super(parent, prevSibling, null, XFA.OCCUR, XFA.OCCUR, null, XFA.OCCURTAG, XFA.OCCUR);
}
// -----------------------------------------
// Overridden methods of Node
// -----------------------------------------
public Attribute defaultAttribute(int eTag) {
// and elements have
// special default values, namely min=0 and max=-1.
Element poParent = getXFAParent();
if (null != poParent) {
if (poParent.isSameClass(XFA.PAGEAREATAG)
|| poParent.isSameClass(XFA.PAGESETTAG)) {
if (eTag == XFA.MINTAG)
return new Int(XFA.MIN, 0, false);
else if (eTag == XFA.MAXTAG)
return new Int(XFA.MAX, -1, false);
}
}
// min defaults to 1 defined in TemplateSchema
// initial defaults to min
if (eTag == XFA.INITIALTAG) {
return getAttribute(XFA.MINTAG);
}
// initial defaults to min
else if (eTag == XFA.MAXTAG) {
Int oMin = (Int)getAttribute(XFA.MINTAG);
if (oMin.getValue() > 0)
return oMin;
}
return super.defaultAttribute(eTag);
}
public Attribute getAttribute(int eTag, boolean bPeek, boolean bValidate) {
Attribute oProperty = super.getAttribute(eTag, bPeek, bValidate);
if (oProperty == null)
return oProperty;
if (eTag == XFA.INITIALTAG) {
Int oValue = (Int)oProperty;
if (isPropertySpecified(XFA.MINTAG,true,0)) {
Int minVal = (Int)getAttribute(XFA.MINTAG);
if (oValue.getValue() < minVal.getValue()) {
foundBadAttribute(eTag, oValue.toString());
return minVal;
}
}
if (isPropertySpecified(XFA.MAXTAG,true,0)) {
Int maxVal = (Int)getAttribute(XFA.MAXTAG);
if ((maxVal.getValue() != -1)
&& (oValue.getValue() > maxVal.getValue())) {
foundBadAttribute(eTag, oValue.toString());
return maxVal;
}
}
}
if (eTag == XFA.MINTAG) {
Int oValue = (Int)oProperty;
if (oValue.getValue() < 0) {
foundBadAttribute(eTag, oValue.toString());
return defaultAttribute(eTag);
}
}
if (eTag == XFA.MAXTAG) {
Int oValue = (Int)oProperty;
Int minVal = (Int)getAttribute(XFA.MINTAG);
if ((oValue.getValue() < -1)
|| ((oValue.getValue() != -1) && (oValue.getValue() < minVal
.getValue()))) {
foundBadAttribute(eTag, oValue.toString());
return minVal;
}
}
return oProperty;
}
/**
* Get the internal maximum as a 32 bit integer
*
* @return the internal value as a 32 bit integer
*/
int getMaximum() {
Int oMax = (Int)getAttribute(XFA.MAXTAG);
return oMax.getValue();
}
/**
* Get the internal minimum as a 32 bit integer
*
* @return the internal value as a 32 bit integer
*/
int getMinimum() {
Int oMin = (Int)getAttribute(XFA.MINTAG);
return oMin.getValue();
}
/**
* Get the internal initial as a 32 bit integer
*
* @return the internal value as a 32 bit integer
*/
int getInitial() {
Int oInit = (Int)getAttribute(XFA.INITIALTAG);
return oInit.getValue();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy