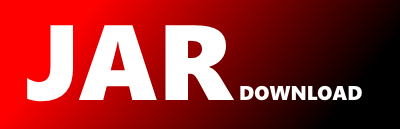
com.adobe.xfa.text.markup.MarkupRtfOut Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aem-sdk-api Show documentation
Show all versions of aem-sdk-api Show documentation
The Adobe Experience Manager SDK
//----------------------------------------------------------------------
//
// ADOBE CONFIDENTIAL
// __________________
//
// Copyright 1995 - 2003 Adobe Systems Incorporated. All
// Rights Reserved.
//
// NOTICE: All information contained herein is, and remains
// the property of Adobe Systems Incorporated and its
// suppliers, if any. The intellectual and technical
// concepts contained herein are proprietary to Adobe Systems
// Incorporated and its suppliers and may be covered by U.S.
// and Foreign Patents, patents in process, and are protected
// by trade secret or copyright law. Dissemination of this
// information or reproduction of this material is strictly
// forbidden unless prior written permission is obtained from
// Adobe Systems Incorporated.
//
//----------------------------------------------------------------------
package com.adobe.xfa.text.markup;
import com.adobe.xfa.gfx.GFXColour;
import com.adobe.xfa.text.TextAttr;
import java.util.ArrayList;
import java.util.List;
/**
* This class is the RTF output markup engine. To use it, one creates
* an instance, passing the string to be translated in a constructor
* parameter. Then the engine is passed to a Markup() method on either
* a text stream or range. Finally, one calls the Translation() method
* on this class to obtain the resulting markup.
*
*
* For more information, please see the extenral documentation.
*
*
* @exclude from published api -- Mike Tardif, May 2006.
*/
public class MarkupRtfOut extends MarkupEngineOut {
private final List moFontTable = new ArrayList();
private final List moColourTable = new ArrayList();
private final boolean mbWriteFontTable;
/**
* Constructor.
* @param pMarkupAttr - (optional) Markup attribute table to use instead
* of the defalt. It is strongly recommended that you pass NULL
* (default).
* @param pColorTable - (optional) Colour table.
* @param pFontTable - (optional) Font table.
*/
public MarkupRtfOut (MarkupAttr pMarkupAttr, List pColorTable, List pFontTable) {
super ((pMarkupAttr == null) ? RTFAttr.GetDefault() : pMarkupAttr);
mbWriteFontTable = pFontTable == null;
if (pColorTable != null) {
moColourTable.addAll(pColorTable);
}
if (pFontTable != null) {
moFontTable.addAll(pFontTable);
}
}
public MarkupRtfOut (MarkupAttr pMarkupAttr, List pColorTable) {
this(pMarkupAttr, pColorTable, null);
}
public MarkupRtfOut (MarkupAttr pMarkupAttr) {
this(pMarkupAttr, null, null);
}
public MarkupRtfOut () {
this(null, null, null);
}
//-----------------------------------------------------------------------------
// Function: Reset
//
// Description:
// Clears the font map.
//
// Parameters: None.
//
// Returns: Void.
//
//-----------------------------------------------------------------------------
// Javaport: not needed if method merely calls super.
// public void reset () {
// super.reset();
// }
// Inherited from MarkupEngineOut
/**
* Obtain the resulting markup.
* @return Generated markup, as a string.
*/
public String translation () {
insertFontTable (translationText());
return super.translation();
}
//-----------------------------------------------------------------------------
// Function: OutputFontFaceName
//
// Description:
// Appends the font table index for the face name to the translation
// text.
//
// Parameters:
// oAttr - text attributes to be translated.
//
// Returns: void
//
//-----------------------------------------------------------------------------
protected String outputFontFaceName (TextAttr oAttr) {
StringBuilder sOut = new StringBuilder();
MarkupAttr pMkAttr = markupAttr();
int nIndex = 0;
boolean bFound = false;
while (nIndex < moFontTable.size()) {
// Ensure that it exists in the map
String typeface = moFontTable.get (nIndex);
if (typeface.equals (oAttr.typeface())) {
bFound = true;
break; // Don't increment nIndex
}
++nIndex;
}
if (! bFound) {
// Add to map if needed - nIndex is the proper value
moFontTable.add (oAttr.typeface());
}
sOut.append (pMkAttr.lookup (MarkupAttr.MARKUP_FONT_NAME));
sOut.append (Integer.toString (nIndex));
sOut.append (pMkAttr.delimiter());
return sOut.toString();
}
protected String outputColour (GFXColour oColour) {
int nIndex = 0;
boolean bFound = false;
while (nIndex < moColourTable.size()) {
// Ensure that it exists in the map
GFXColour test = moColourTable.get (nIndex);
if (test.equals (oColour)) {
bFound = true;
break; // Don't increment nIndex
}
++nIndex;
}
if (! bFound) {
// Add to map if needed - nIndex is the proper value
moColourTable.add (oColour);
}
StringBuilder sOut = new StringBuilder (markupAttr().lookup (MarkupAttr.MARKUP_COLOUR));
sOut.append (Integer.toString (nIndex));
sOut.append (markupAttr().delimiter());
return sOut.toString();
}
//----------------------------------------------------------------------
//
// Special character handling - implementations
//
//----------------------------------------------------------------------
protected boolean isSpecialChar (char c) {
if (c < 31 || c > 126) {
// Check if we're outside the range of printable characters
return true;
}
return false;
}
protected String convertSpecialChar (char c) {
if (c < 31 || c > 126) {
// RTF outputs unprintable characters as hex values eg: \'a9
int ic = ((int) c) & 0xFFFF;
return Integer.toHexString (ic);
}
String sReturn = "";
return sReturn + c;
}
//-----------------------------------------------------------------------------
// Function: Translation
//
// Description:
// Ancestor override to insert the font table information into the
// translated RTF string before returning it.
//
// Parameters: None.
//
// Returns: Void.
//
//-----------------------------------------------------------------------------
private void insertFontTable (StringBuilder oStrTranslation) {
if (! mbWriteFontTable) {
return;
}
StringBuilder oStrFontTableText = new StringBuilder ("{\\fonttbl{}");
// jfString oStrFontCommand;
// jfString oStrFontName;
// jfString oStrFontTableIndex;
// int iFontTableInsertionIndex;
for (int nIndex = 0; nIndex < moFontTable.size(); ++nIndex) {
// Linear insertion preceding second last scoped block closing brace '}'
// iFontTableInsertionIndex = oStrFontTableText.length() - 2;
StringBuilder oStrFontCommand = new StringBuilder ("{"); // Begin new scoped block
// Construct the font spec string in the form: "\f3\fcharset0\fprq2 Courier"
oStrFontCommand.append (markupAttr().lookup (MarkupAttr.MARKUP_FONT_NAME));
oStrFontCommand.append (Integer.toString (nIndex));
oStrFontCommand.append ("\\fcharset0\\fprq2 "); // ASCII charset
oStrFontCommand.append (moFontTable.get (nIndex)); // The goods
oStrFontCommand.append ('}'); // End of block
oStrFontTableText.append (oStrFontCommand);
}
oStrFontTableText.append ('}');
// Add the font table and the rtf1 directive
oStrTranslation.insert (0, oStrFontTableText);
oStrTranslation.insert (0, "{\\rtf1");
oStrTranslation.append ('}');
// Insert some carriage returns or else other RTF readers will have problems
// with line size
int i = oStrTranslation.indexOf ("{");
while (i >= 0) {
if ((oStrTranslation.charAt (i - 1) != '\n') && (oStrTranslation.charAt (i - 1) != '\\')) {
// Precede opening braces with a carriage return
oStrTranslation.insert ('\n', i);
i++;
}
i = oStrTranslation.indexOf ("{", i + 1);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy