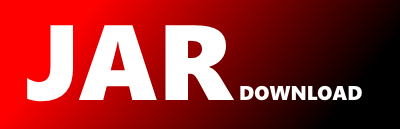
com.adobe.xfa.ut.Angle Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2005 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.ut;
/**
* A class to represent an angle between 0° and 360°.
*
* Instances of this class are immutable. All change operations
* return a new instance of this Angle
class.
*/
public final class Angle {
private static final int ANGLE_360_RESVALUE = 360000;
private static final int ANGLE_MAX_RESVALUE = 360000;
private static final int ANGLE_MIN_RESVALUE = 0;
/**
* The Angle
corresponding to the 0 angle.
*
* @exclude from published api.
*/
public final static Angle ZERO = new Angle();
private final int mlResValue;
/**
* Instantiates an Angle
of 0°.
*/
public Angle() {
mlResValue = 0;
}
/**
* Instantiates an Angle
from the
* given Angle
.
*
* @param source
* the Angle
to copy to this object.
* @deprecated Angle is immutable, so there is no need to copy an instance.
*/
public Angle(Angle source) {
mlResValue = source.mlResValue;
}
/**
* Instantiates an Angle
from the given double value.
* Angle values are expressed in degrees: e.g., 15.5 means 15
* and 1/2 degrees, not 15 degrees and 50 minutes.
*
* @param dDegrees
* the degree of the angle.
*/
public Angle(double dDegrees) {
mlResValue = resolve(dDegrees);
}
/**
* Instantiates an Angle
from the given int value.
*
* @param nDegrees
* the degree of the angle.
*/
public Angle(int nDegrees) {
mlResValue = resolve(nDegrees);
}
/**
* Instantiates an Angle
from the given long value.
*
* @param nDegrees
* the degree of the angle.
*/
public Angle(long nDegrees) {
mlResValue = resolve(nDegrees);
}
/**
* Instantiates an Angle
from the given int value.
*
* @param value
* the value of the angle.
* @param isResolved
* when true, interpret the angle value as in unit
* of internal resolution; when false, interpret
* the angle value as a degree.
*
* @exclude from published api.
*/
public Angle(int value, boolean isResolved) {
mlResValue = isResolved ? value : resolve(value);
}
/**
* Returns an Angle
representing the
* addition of this object and the given Angle
.
*
* @param oAdd
* the Angle
to subtract.
* @return
* an angle of the addition.
*/
public Angle add(Angle oAdd) {
//
// add the angle passed in to this angle.
//
int lNewresValue = resValue() + oAdd.resValue();
//
// if the sum of the two angles is > 360 degrees, subtract
// one rotation.
//
if (lNewresValue > ANGLE_MAX_RESVALUE)
lNewresValue -= ANGLE_360_RESVALUE;
return new Angle(lNewresValue, true);
}
/**
* Gets this object's angle value.
*
* @return
* the angle, in degrees, rounded
* to the closest integer.
*/
public int degrees() {
return ((int) Math.round(resValue() / 1000.0d));
}
/**
* Determines if this object is equal to the given Object.
*
* @param object
* the angle to compare.
* @return
* true if equal, false otherwise.
*/
public boolean equals(Object object) {
if (this == object)
return true;
// This overrides Object.equals(boolean) directly, so...
if (object == null)
return false;
if (object.getClass() != getClass())
return false;
return resValue() == ((Angle)object).resValue();
}
/**
* Returns a hash code value for the object.
* @exclude from published api.
*/
public int hashCode() {
int hash = 11;
return (hash * 31) ^ resValue();
}
/**
* Gets this object's angle value.
*
* @return
* the angle in degrees.
*/
public double getAngle() {
return (resValue() / 1000.0d);
}
/**
* Determines if this object is greater than the given
* Angle
.
*
* @param compare
* the Angle
to compare.
* @return
* true if greater than, false otherwise.
*/
public boolean gt(Angle compare) {
return resValue() > compare.resValue();
}
/**
* Determines if this object is greater than or equal to the given
* Angle
.
*
* @param compare
* the Angle
to compare.
* @return
* true if greater than, or equal to, false otherwise.
*/
public boolean gte(Angle compare) {
return resValue() >= compare.resValue();
}
/**
* Determines if this object is less than the given
* Angle
.
*
* @param compare
* the Angle
to compare.
* @return
* true if less than, false otherwise.
*/
public boolean lt(Angle compare) {
return resValue() < compare.resValue();
}
/**
* Determines if this object is less than or equal to the given
* Angle
.
*
* @param compare
* the Angle
to compare.
* @return
* true if less than or equal to, false otherwise.
*/
public boolean lte(Angle compare) {
return resValue() <= compare.resValue();
}
// Javaport: commented out. Internal representations are private.
// private int resolution() {
// return 1000;
// }
private static int resolve(double value) {
// TODO: why not just reduce the value mod 360, rather than throwing error?
// check that new angle is not out of the range.
if ((value < ANGLE_MIN_RESVALUE) || (value > ANGLE_MAX_RESVALUE))
throw new ExFull(ResId.ANGLE_ERR_RANGE);
return (int) (value * 1000.0d); // note: truncated, not rounded
}
private static int resolve(int value) {
// TODO: why not just reduce the value mod 360, rather than throwing error?
// check that new angle is not out of the range.
if ((value < ANGLE_MIN_RESVALUE) || (value > ANGLE_MAX_RESVALUE))
throw new ExFull(ResId.ANGLE_ERR_RANGE);
return value * 1000;
}
private static int resolve(long value) {
// TODO: why not just reduce the value mod 360, rather than throwing error?
// check that new angle is not out of the range.
if ((value < ANGLE_MIN_RESVALUE) || (value > ANGLE_MAX_RESVALUE))
throw new ExFull(ResId.ANGLE_ERR_RANGE);
return (int) (value * 1000L); // note: truncated, not rounded
}
private int resValue() {
return mlResValue;
}
/**
* Returns an Angle
representing the
* subtraction of this object and the given Angle
.
*
* @param subtract
* the Angle
to subtract.
* @return
* an angle of the subtraction.
*/
public Angle subtract(Angle subtract) {
//
// subtract the angle passed in from this angle.
//
int lNewresValue = resValue() - subtract.resValue();
//
// if the difference is < 0 degrees, add one rotation.
//
if (lNewresValue < ANGLE_MIN_RESVALUE)
lNewresValue += ANGLE_360_RESVALUE;
return new Angle(lNewresValue, true);
}
/**
* Returns a string representation of this object.
* @exclude from published api.
*/
public String toString() {
return Double.toString(getAngle());
}
/**
* The zero angle.
*
* @return
* the angle equal to 0°.
*/
public static Angle zero() {
return ZERO;
}
}