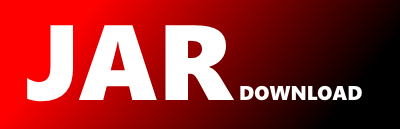
com.adobe.xfa.ut.Margins Maven / Gradle / Ivy
Show all versions of aem-sdk-api Show documentation
/*
* ADOBE CONFIDENTIAL
*
* Copyright 2007 Adobe Systems Incorporated All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of
* Adobe Systems Incorporated and its suppliers, if any. The intellectual and
* technical concepts contained herein are proprietary to Adobe Systems
* Incorporated and its suppliers and may be covered by U.S. and Foreign
* Patents, patents in process, and are protected by trade secret or copyright
* law. Dissemination of this information or reproduction of this material
* is strictly forbidden unless prior written permission is obtained from
* Adobe Systems Incorporated.
*/
package com.adobe.xfa.ut;
/**
* A class to describe a set of margins. It consists of left, top, right, and, bottom
* rectangle insets.
*
* Instances of this class are immutable.
*/
public final class Margins {
/**
* Instantiates Margins
with zero insets.
*/
public Margins() {
mMarginLeft = UnitSpan.ZERO;
mMarginTop = UnitSpan.ZERO;
mMarginRight = UnitSpan.ZERO;
mMarginBottom = UnitSpan.ZERO;
}
/**
* Instantiates Margins
specified by
* the given UnitSpan
insets.
*
* @param marginLeft the left margin.
* @param marginTop the top margin.
* @param marginRight the right margin.
* @param marginBottom the bottom margin.
*/
public Margins(UnitSpan marginLeft,
UnitSpan marginTop,
UnitSpan marginRight,
UnitSpan marginBottom) {
mMarginLeft = marginLeft;
mMarginTop = marginTop;
mMarginRight = marginRight;
mMarginBottom = marginBottom;
}
/**
* Instantiates Margins
from the
* given Margins
.
*
* @param source
* the Margins
to copy to this object.
*/
public Margins(Margins source) {
mMarginLeft = source.mMarginLeft;
mMarginTop = source.mMarginTop;
mMarginRight = source.mMarginRight;
mMarginBottom = source.mMarginBottom;
}
/**
* Determines if this object is equal to the given margins.
*
* @param object
* the margins to compare.
* @return
* true if equal, false otherwise.
*/
public boolean equals(Object object) {
if (this == object)
return true;
// This overrides Object.equals(boolean) directly, so...
if (object == null)
return false;
if (object.getClass() != getClass())
return false;
Margins oCompare = (Margins) object;
return mMarginLeft.equals(oCompare.mMarginLeft) &&
mMarginTop.equals(oCompare.mMarginTop) &&
mMarginRight.equals(oCompare.mMarginRight) &&
mMarginBottom.equals(oCompare.mMarginBottom);
}
/**
* Returns a hash code value for the object. This method is unsupported.
* @exclude from published api.
*/
public int hashCode() {
int hash = 13;
hash = (hash * 31) ^ mMarginLeft.hashCode();
hash = (hash * 31) ^ mMarginTop.hashCode();
hash = (hash * 31) ^ mMarginRight.hashCode();
hash = (hash * 31) ^ mMarginBottom.hashCode();
return hash;
}
/**
* Gets this object's bottom margin.
*
* @return
* the bottom margin.
*/
public UnitSpan marginBottom() {
return mMarginBottom;
}
// Javaport: disabled to make class immutable.
// public void marginBottom(UnitSpan oNewMarginBottom) {
// moMarginBottom = oNewMarginBottom;
// }
/**
* Gets this object's left margin.
*
* @return
* the left margin.
*/
public UnitSpan marginLeft() {
return mMarginLeft;
}
// Javaport: disabled to make class immutable.
// public Margins marginLeft(UnitSpan oNewMarginLeft) {
// moMarginLeft = oNewMarginLeft;
// }
/**
* Gets this object's top margin.
*
* @return
* the top margin.
*/
public UnitSpan marginTop() {
return mMarginTop;
}
// Javaport: disabled to make class immutable.
// public Margins marginTop(UnitSpan oNewMarginTop) {
// moMarginTop = oNewMarginTop;
// }
/**
* Gets this object's right margin.
*
* @return
* the right margin.
*/
public UnitSpan marginRight() {
return mMarginRight;
}
// Javaport: disabled to make class immutable.
// public Margins marginRight(UnitSpan oNewMarginRight) {
// moMarginRight = oNewMarginRight;
// }
// Javaport: disabled to make class immutable.
// public void scale(double dScale) {
// moMarginLeft.multiply(dScale);
// moMarginRight.multiply(dScale);
// moMarginTop.multiply(dScale);
// moMarginBottom.multiply(dScale);
// }
private final UnitSpan mMarginLeft;
private final UnitSpan mMarginTop;
private final UnitSpan mMarginRight;
private final UnitSpan mMarginBottom;
}